java流程控制
1. 用户交互Scanner
我们可以通过scanner类来获取用户的输入
scanner类中的next()与nextLine()方法获取输入的字符串,在读取之前我们一般需要使用hasNext()与hasNextLine判断是否还有输入的数据。
import java.util.Scanner;
public class Demo01 {
public static void main(String[] args) {
Scanner s = new Scanner(System.in);
System.out.println("使用next方式接受:");
if (s.hasNext()){
String str = s.next();
System.out.println("输出" + str);
}
s.close();
}
}
next()与nextLine()区别
next()不能得到带有空格的字符串
2.顺序结构
上到下
public class Demo01 {
public static void main(String[] args) {
int score = 4;
switch (score){
case 1:
System.out.println("1分");
break;
case 2:
System.out.println("2分");
break;
case 3:
System.out.println("3分");
break;
case 4:
System.out.println("4分");
break;
case 5:
System.out.println("5分");
break;
}
}
}
3.循环结构
一个园
while(){}:先判断后执行
public class Demo01 {
public static void main(String[] args) {
int i = 0;
while (i<100){
i++;
System.out.println(i);
}
}
}
do{}while():先执行后判断
public class Demo01 {
public static void main(String[] args) {
int i = 0;
do {
i++;
System.out.println(i);
}while (i<100);
}
}
for(){}
public class Demo01 {
public static void main(String[] args) {
for (int i=0;i<100;i++){
System.out.println(i);
}
}
}
九九乘法表代码
public class Demo01 {
public static void main(String[] args) {
for (int i=1;i<=9;i++){
for (int j=1;j<=i;j++){
System.out.print(i+"*"+j+"="+(i*j)+"\t");
}
System.out.println();
}
}
}
遍历数组
扫描二维码关注公众号,回复:
9494986 查看本文章
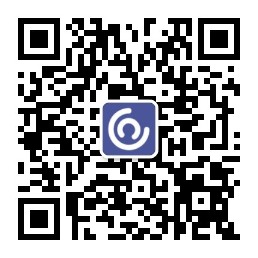
public class Demo01 {
public static void main(String[] args) {
int[] num = {10,20,30,40,50,60};
for (int x:num){
System.out.println(x);
}
}
}
4.条件结构
有限制
if(s.equals("hello")){
//equals:判断字符串是否相等
}
break与continue
- break用于强行退出循环
- continue用于终止某次循环
java方法
- 方法:一个方法只完成1个功能
public class Demo01 {
public static void main(String[] args) {
int max = max(10,10);
System.out.println(max);
}
public static int max(int a,int b){
int result=0;
if (a==b){
System.out.println("两数相等");
return 0;
}
if (a>b){
result = a;
}else {
result = b;
}
return result;
}
}
- 方法重载:一个类中,相同的函数名字,但形参不同
public class Demo01 {
public static void main(String[] args) {
double max = max(10.1,10.2);
System.out.println(max);
}
public static int max(int a,int b){
int result=0;
System.out.println("方法int");
if (a==b){
System.out.println("两数相等");
return 0;
}
if (a>b){
result = a;
}else {
result = b;
}
return result;
}
public static double max(double a,double b){
double result=0;
System.out.println("方法float");
if (a==b){
System.out.println("两数相等");
return 0;
}
if (a>b){
result = a;
}else {
result = b;
}
return result;
}
}
自己写的计算器小demo
import java.util.Scanner;
public class Demo01 {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
while (true) {
System.out.println("请写出两个数字,用空格隔开");
double a = scan.nextDouble();
double b = scan.nextDouble();
System.out.println("填写序列号:1.加2.减,3.乘4.除");
int c = scan.nextInt();
double d = 0;
switch (c) {
case 1:
d = a + b;
break;
case 2:
d = a - b;
break;
case 3:
d = a * b;
break;
case 4:
d = a / b;
break;
}
System.out.println("结果为:" + d);
}
}
}