使用Mybatis-generator生成实体类与dao层相关信息后, 需要用到一对多查询时, 修改SQL映射文件
1. 数据库结构
1.1 奖项(award)表
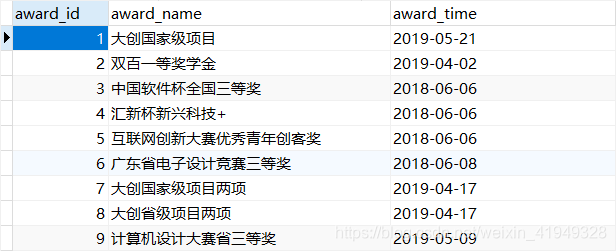
1.2奖项照片表(award_photo)

2. 实体类描述
2.1 Award
package com.turing.team_website.entity;
import com.fasterxml.jackson.annotation.JsonFormat;
import lombok.*;
import java.util.Date;
import java.util.List;
@Getter
@Setter
@NoArgsConstructor
@AllArgsConstructor
@ToString
public class Award {
private Integer awardId;
private String awardName;
@JsonFormat(pattern = "yyyy-MM-dd")
private Date awardTime;
private List<String> photoLocList;
}
2.2 AwardMapper
package com.turing.team_website.dao;
import com.turing.team_website.entity.Award;
import com.turing.team_website.entity.AwardExample;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Param;
import java.util.List;
@Mapper
public interface AwardMapper {
long countByExample(AwardExample example);
int deleteByExample(AwardExample example);
int insert(Award record);
int insertSelective(Award record);
List<Award> selectByExample(AwardExample example);
int updateByExampleSelective(@Param("record") Award record, @Param("example") AwardExample example);
int updateByExample(@Param("record") Award record, @Param("example") AwardExample example);
}
3.SQL映射文件
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.turing.team_website.dao.AwardMapper">
<resultMap id="BaseResultMap" type="com.turing.team_website.entity.Award">
<result column="award_id" jdbcType="INTEGER" property="awardId" />
<result column="award_name" jdbcType="VARCHAR" property="awardName" />
<result column="award_time" jdbcType="DATE" property="awardTime" />
</resultMap>
<resultMap id="WithPhotoBaseResultMap" type="com.turing.team_website.entity.Award">
<result column="award_id" jdbcType="INTEGER" property="awardId" />
<result column="award_name" jdbcType="VARCHAR" property="awardName" />
<result column="award_time" jdbcType="DATE" property="awardTime" />
<collection property="photoLocList" ofType="java.lang.String" javaType="java.util.List">
<result column="award_photo_loc" />
</collection>
</resultMap>
<sql id="Example_Where_Clause">
...
</sql>
<sql id="Base_Column_List">
award_id, award_name, award_time
</sql>
<sql id="With_PhotoLoc_Base_Column_List">
a.award_id, a.award_name, a.award_time, ap.award_photo_loc
</sql>
<select id="selectByExample" parameterType="com.turing.team_website.entity.AwardExample" resultMap="WithPhotoBaseResultMap">
select
<if test="distinct">
distinct
</if>
<include refid="With_PhotoLoc_Base_Column_List" />
from award a inner join award_photo ap where a.award_id = ap.award_id
<if test="_parameter != null">
<include refid="Example_Where_Clause" />
</if>
<if test="orderByClause != null">
order by ${orderByClause}
</if>
</select>
...其它省略
</mapper>
关键字 |
collection |
ofType |
javaType |
解释 |
一对多查询时使用的标签 |
多的一方的数据类型 |
多的一方的封装集合类型(数组,集合…) |