一、添加事件监听与方向判断
- 在
start()
中添加事件监听addEventHandler()方法
。
start() {
...
this.addEventHandler();
},
- 定义一个常量
MIN_LENGTH
指定划动的最短距离,过短就不触发事件。编写addEventHandler()方法,从bg节点获取划动起始位置startPoint
和结束位置endPoint
,两者相减为划动的向量vec
。在vec长度大于MIN_LENGTH的情况下,判断它往哪个方向划动,分别调用moveRight()
、moveLeft()
、moveUp()
、moveDown()
函数。
const MIN_LENGTH = 50;
...
addEventHandler() {
this.bg.on('touchstart', (event) => {
this.startPoint = event.getLocation();
});
this.bg.on('touchend', (event) => {
this.endPoint = event.getLocation();
let vec = this.endPoint.sub(this.startPoint);
if (vec.mag() > MIN_LENGTH) {
if (Math.abs(vec.x) > Math.abs(vec.y)) {
if (vec.x > 0) {
this.moveRight();
} else {
this.moveLeft();
}
} else {
if (vec.y > 0) {
this.moveUp();
} else {
this.moveDown();
}
}
}
});
},
- 简单在四个
move()函数
中打印相关log,点击预览。
moveLeft() {
console.log('move left');
},
moveRight() {
console.log('move right');
},
moveUp() {
console.log('move up');
},
moveDown() {
console.log('move down');
},
- 依次往上、往左、往下、往右划动(此时需要保证起始位置和保持结束位置均在画面中,不能在周围黑色处划动),可看到
console
中依次打印了四条语句。
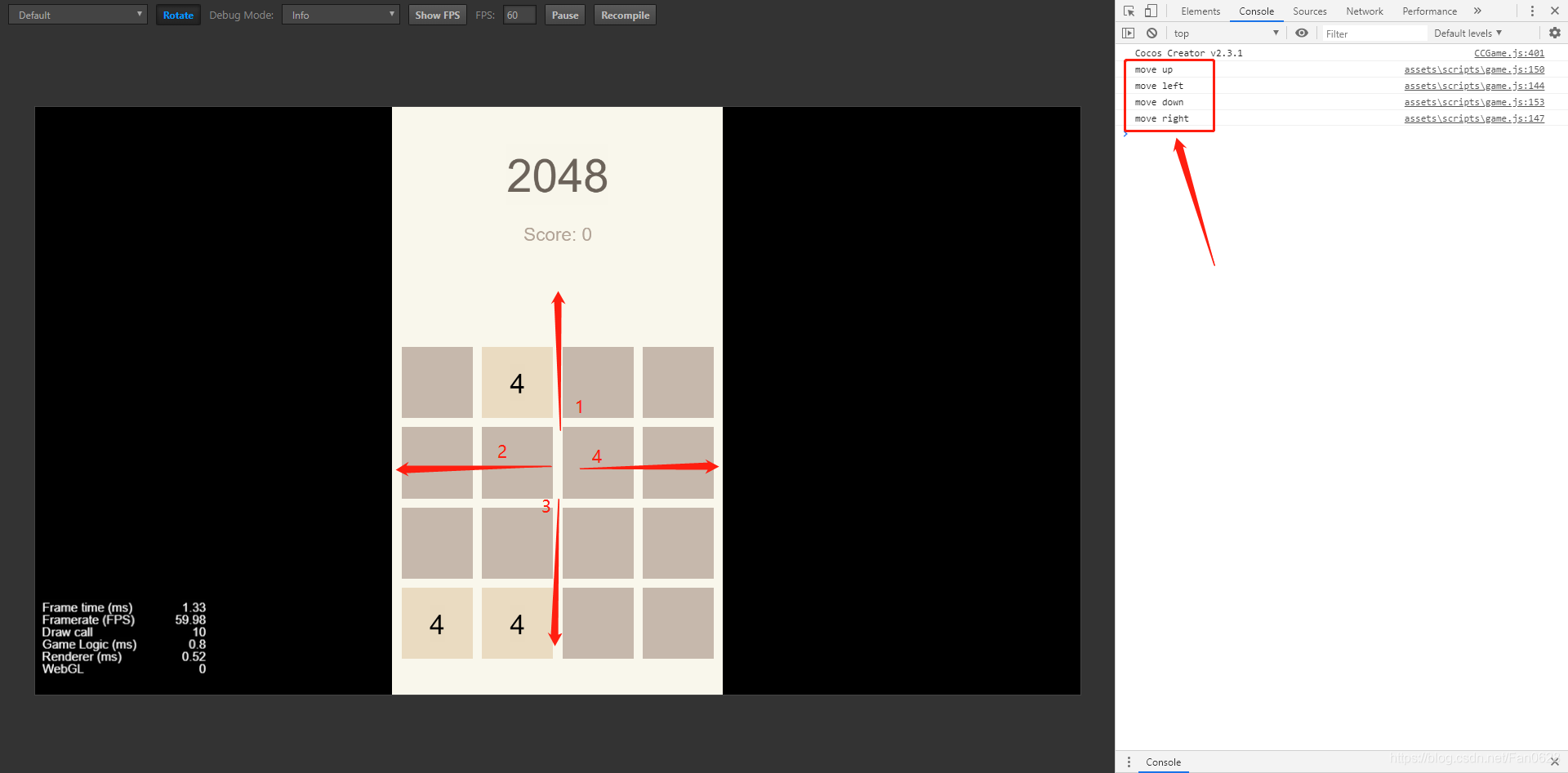
二、优化方向判断
- 实际情况存在划动结束位置在屏幕外的情况,因此需要进行相关优化。
- 将touchend事件的相关操作封装为
touchEnd(event)方法
。
touchEnd(event) {
this.endPoint = event.getLocation();
let vec = this.endPoint.sub(this.startPoint);
if (vec.mag() > MIN_LENGTH) {
if (Math.abs(vec.x) > Math.abs(vec.y)) {
if (vec.x > 0) {
this.moveRight();
} else {
this.moveLeft();
}
} else {
if (vec.y > 0) {
this.moveUp();
} else {
this.moveDown();
}
}
}
},
- 而后改进
addEventHandler()
,在touchend事件
和touchcancel事件
中添加touchEnd(event)方法
。
addEventHandler() {
...
this.bg.on('touchend', (event) => {
this.touchEnd(event);
});
this.bg.on('touchcancel', (event) => {
this.touchEnd(event);
});
},
- 此时点击预览,依次往左、往上、往右、往下划动,可划出屏幕外,可看到
console
中依次打印了四条语句。
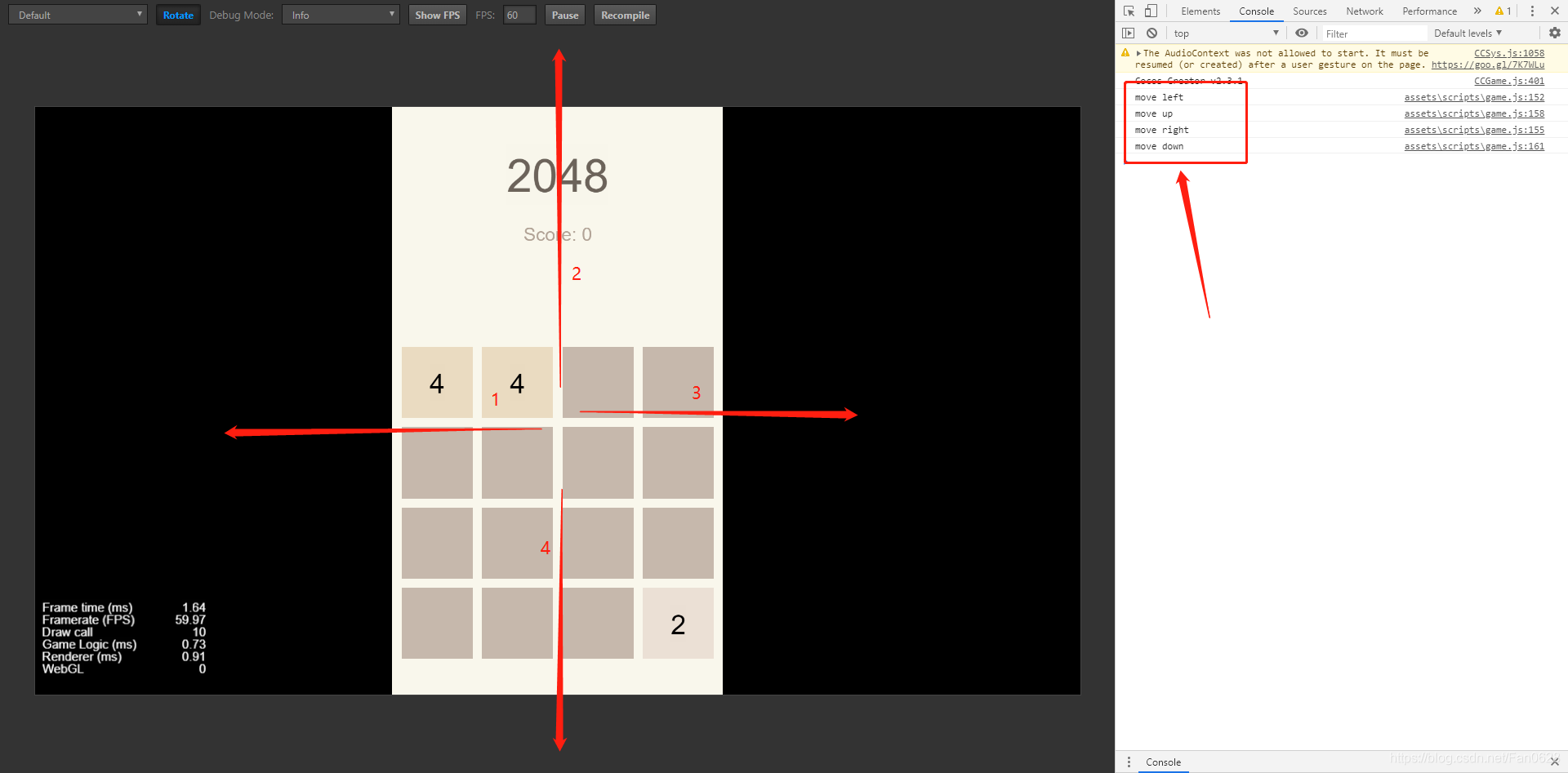