智慧源于勤奋 伟大出自平凡
模拟java.util.Array.sort工具
public class TestSort {
public static void main(String[] args) {
Student[] students = new Student[]{new Student("tom" , 20 , "male" , 90D) ,
new Student("jack" , 19 , "male" , 91D) , new Student("marry" , 21 , "male" , 90D)};
Tool.sort(students);//工具的调用者
for(int i = 0; i < students.length;i++){
System.out.println(students[i].name + "\t" + students[i].score +"\t" + students[i].age);
}
}
}
class Student implements Comparable<Student>{//接口的实现者
String name;
int age;
String sex;
double score;
public Student(){}
public Student(String name, int age, String sex, double score) {
super();
this.name = name;
this.age = age;
this.sex = sex;
this.score = score;
}
@Override
public int comparTo(Student stu) {
if(this.score > stu.score){
return 1;
}else if(this.score < this.score){
return -1;
}
else if(this.score == stu.score){
if(this.age > stu.age){
return 1;
}
}
return 0;
}
}
public class Tool <T>{//接口的使用者
public static void sort(Student[] stu){
for(int i = 0; i < stu.length - 1;i++){
Comparable com = (Comparable)stu[i];
int n = com.comparTo(stu[i+1]);
if(i > 0){
Student temp = stu[i];
stu[i] = stu[i+1];
stu[i+1] = temp;
}
}
}
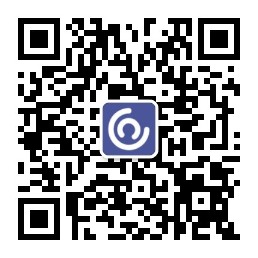
}
public interface Comparable<T>{//接口
int comparTo(T stu);
//this.与下一个比较 ,如果为正数 则是升序,如果是负数,则是降序,如果为0,不变
}
调用java.util.Arrays.sort写实现类进行排序
public class TestTeacher {
public static void main(String[] args) {
Teacher[] teachers = new Teacher[]{new Teacher("tom" , 28) , new Teacher("jack" , 30) ,
new Teacher("marry" , 25)};
java.util.Arrays.sort(teachers);
for(int i = 0; i < teachers.length;i++){
System.out.println(teachers[i].name + "\t" + teachers[i].age);
}
}
}
class Teacher implements Comparable<Teacher>{
String name;
int age;
public Teacher(){}
public Teacher(String name, int age) {
super();
this.name = name;
this.age = age;
}
@Override
public int compareTo(Teacher t) {
if(this.age > t.age){
return 1;
}
else if(this.age < t.age){
return -1;
}
return 0;
}
}
Usb接口来说明耦合度低的好处
public class TestUsb {
public static void main(String[] args) {
Computer computer = new Computer();
Fan fan = new Fan();
Light light = new Light();
computer.on(fan);
System.out.println("-------------------");
computer.on(fan,light);
}
}
class Computer{
Usb u1;
Usb u2;
public void on(Usb u){
this.u1 = u;
u1.service();
}
public void on(Usb u1 , Usb u2){
this.u1 = u1;
this.u2 = u2;
u1.service();;
u2.service();
}
}
interface Usb{
void service();
}
class Fan implements Usb{
@Override
public void service() {
System.out.println("旋转。。。");
}
}
class Light implements Usb{
@Override
public void service() {
System.out.println("发光。。。");
}
}
public class TestUsb {
public static void main(String[] args) {
Computer computer = new Computer();
Fan fan = new Fan();
Light light = new Light();
computer.on(fan);
System.out.println("-------------------");
computer.on(fan,light);
}
}
class Computer{
Usb u1;
Usb u2;
public void on(Usb u){
this.u1 = u;
u1.service();
}
public void on(Usb u1 , Usb u2){
this.u1 = u1;
this.u2 = u2;
u1.service();;
u2.service();
}
}
interface Usb{
void service();
}
class Fan implements Usb{
@Override
public void service() {
System.out.println("旋转。。。");
}
}
class Light implements Usb{
@Override
public void service() {
System.out.println("发光。。。");
}
}