ImageView:获取图片的原始大小,利用一个进度栏控制图片缩放和旋转
前端XML代码
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<ImageView android:id="@+id/imageview"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/beijing1"
android:scaleType="fitCenter"
>
</ImageView>
<TextView
android:id="@+id/textview1"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:text=""
/>
<SeekBar
android:id="@+id/seekbar1"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:max="240"
android:progress="120"
/>
<TextView
android:id="@+id/textview2"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:text="0度"
/>
<SeekBar
android:id="@+id/seekbar2"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:max="360"
/>
</LinearLayout>
Activity代码
public class MainActivity extends AppCompatActivity implements SeekBar.OnSeekBarChangeListener {
private int minWidth = 100;
private ImageView imageView;
private TextView textView1, textView2;
private Matrix matrix = new Matrix();
int width;
int height;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.image);
imageView = (ImageView) this.findViewById(R.id.imageview);
SeekBar seekBar1 = (SeekBar) this.findViewById(R.id.seekbar1);
SeekBar seekBar2 = (SeekBar) this.findViewById(R.id.seekbar2);
textView1 = (TextView) this.findViewById(R.id.textview1);
textView2 = (TextView) this.findViewById(R.id.textview2);
seekBar1.setOnSeekBarChangeListener(this);
seekBar2.setOnSeekBarChangeListener(this);
DisplayMetrics dm = new DisplayMetrics();
getWindowManager().getDefaultDisplay().getMetrics(dm);
seekBar1.setMax(dm.widthPixels - minWidth);
}
@Override
public void onProgressChanged(SeekBar seekBar, int progress,
boolean fromUser) {
BitmapFactory.Options opts = new BitmapFactory.Options();
opts.inJustDecodeBounds = true;
BitmapFactory.decodeResource(getResources(), R.drawable.beijing1, opts);
opts.inSampleSize = 1;
opts.inJustDecodeBounds = false;
width = opts.outWidth;
height = opts.outHeight;
textView1.setText("图像宽度:" + width + " 图像高度:" + height);
if (seekBar.getId() == R.id.seekbar1) {
int newWidth = progress + minWidth;
int newHeight = (int) (newWidth * height / width);
imageView.setLayoutParams(new LinearLayout.LayoutParams(newWidth, newHeight));
textView1.setText("图像宽度:" + newWidth + " 图像高度:" + newHeight);
} else
if (seekBar.getId() == R.id.seekbar2) {
matrix.setRotate(progress);
Bitmap bitmap = ((BitmapDrawable) (getResources().getDrawable(R.drawable.beijing1))).getBitmap();
bitmap = Bitmap.createBitmap(bitmap, 0, 0, bitmap.getWidth(), bitmap.getHeight(), matrix, true);
imageView.setImageBitmap(bitmap);
textView2.setText(progress + "度");
}
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) {
}
@Override
public void onStopTrackingTouch(SeekBar seekBar) {
}
}
效果
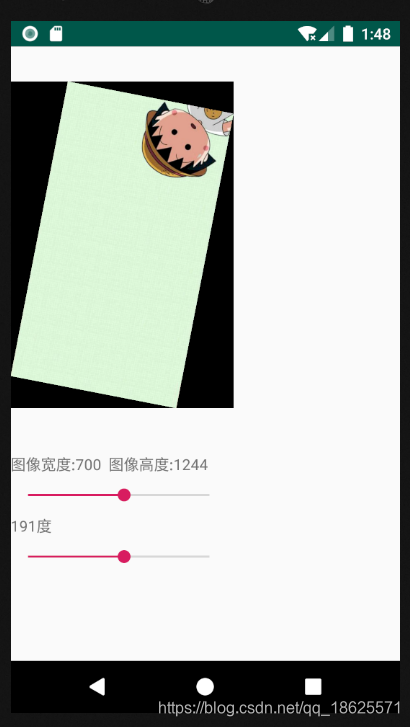