获取所有队列 http://127.0.1.1:15672/api/queues
@RequestMapping("getAllQueues")
public Result getAllQueues() {
String url = "http://" + host + ":15672/api/queues";
String result = null;
try {
result = HttpKit.Get(url, username, password);
List list = JSON.parseObject(result, List.class);
return new Result(true, "请求成功", list);
} catch (IOException e) {
return new Result(true,"请求失败");
}
}
获取到的结果
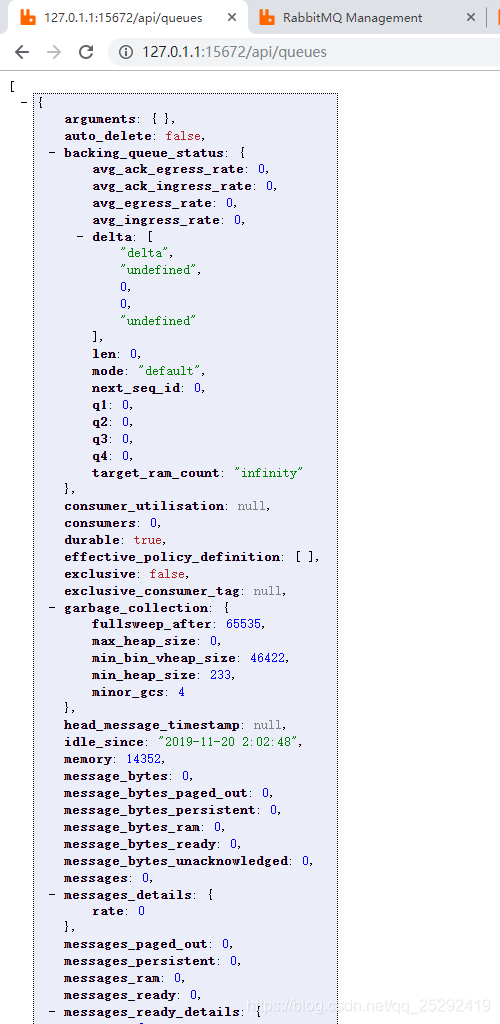
[
{
"arguments": {},
"auto_delete": false,
"backing_queue_status": {
"avg_ack_egress_rate": 0.0,
"avg_ack_ingress_rate": 0.0,
"avg_egress_rate": 0.0,
"avg_ingress_rate": 0.0,
"delta": [
"delta",
"undefined",
0,
0,
"undefined"
],
"len": 0,
"mode": "default",
"next_seq_id": 0,
"q1": 0,
"q2": 0,
"q3": 0,
"q4": 0,
"target_ram_count": "infinity"
},
"consumers": 0,
"durable": true,
"effective_policy_definition": [],
"exclusive": false,
"garbage_collection": {
"fullsweep_after": 65535,
"max_heap_size": 0,
"min_bin_vheap_size": 46422,
"min_heap_size": 233,
"minor_gcs": 5
},
"idle_since": "2019-11-20 3:40:56",
"memory": 14352,
"message_bytes": 0,
"message_bytes_paged_out": 0,
"message_bytes_persistent": 0,
"message_bytes_ram": 0,
"message_bytes_ready": 0,
"message_bytes_unacknowledged": 0,
"messages": 0,
"messages_details": {
"rate": 0.0
},
"messages_paged_out": 0,
"messages_persistent": 0,
"messages_ram": 0,
"messages_ready": 0,
"messages_ready_details": {
"rate": 0.0
},
"messages_ready_ram": 0,
"messages_unacknowledged": 0,
"messages_unacknowledged_details": {
"rate": 0.0
},
"messages_unacknowledged_ram": 0,
"name": "115_hiwatchclient",
"node": "rabbit@DESKTOP-QGC8I2T",
"reductions": 6624,
"reductions_details": {
"rate": 0.0
},
"state": "running",
"vhost": "/"
},
]
需要的工具类
import java.io.IOException;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.auth.AuthScope;
import org.apache.http.auth.UsernamePasswordCredentials;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.CredentialsProvider;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.BasicCredentialsProvider;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.http.util.EntityUtils;
public class HttpKit {
public static String Get(String url, String username, String password) throws IOException{
HttpClientBuilder httpClientBuilder = HttpClientBuilder.create();
CredentialsProvider provider = new BasicCredentialsProvider();
AuthScope scope = new AuthScope(AuthScope.ANY_HOST, AuthScope.ANY_PORT, AuthScope.ANY_REALM);
UsernamePasswordCredentials credentials = new UsernamePasswordCredentials(username, password);
provider.setCredentials(scope, credentials);
httpClientBuilder.setDefaultCredentialsProvider(provider);
CloseableHttpClient closeableHttpClient = httpClientBuilder.build();
String result = "";
HttpGet httpGet = null;
HttpResponse httpResponse = null;
HttpEntity entity = null;
httpGet = new HttpGet(url);
try {
httpResponse = closeableHttpClient.execute(httpGet);
entity = httpResponse.getEntity();
if( entity != null ){
result = EntityUtils.toString(entity);
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
closeableHttpClient.close();
return result;
}
}