源代码组织方式
Java程序由package+class组成,package对应目录的相对路径,class对应文件,如
E:\Workspaces\MyEclipse 10\JavaStudy\src\com\happyframework\javastudy\hello\Hello.java
1 package com.happyframework.javastudy.hello;
2
3 public final class Hello {
4 public static void hello(){
5 System.out.println("hello!");
6 }
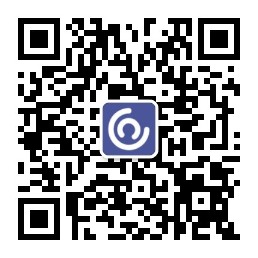
7 }
关于class有如下几点规则:
文件的名字必须和class的名字一致(public级别的class名字)。
文件必须只包含一个public访问基本的class(可以包含多个非public级别的class)。
package名字必须和目录一致。
入口方法
App.java
1 public class App {
2 public static void main(String[] args) {
3 com.happyframework.javastudy.hello.Hello.hello();
4 }
5 }
最终的项目结构
数据类型
8种原子类型
整数类型:byte、short、int和long。
小数类型:float和double。
字符类型:char。
布尔类型:bool。
除此之外的是interface、class和array。
小数类型的常量默认是double类型,声明float类型的常量需要使用F作为后缀。
1 public class Program {
2
3 /**
4 * @param args
5 */
6 public static void main(String[] args) {
7 float age = 28.0F;
8 System.out.println(age);
9 }
10
11 }
运算符
算术运算符:+、-、*、/ 和 %,两个整数相除,结果还是整数。
赋值运算符:=、+=、-=、*=、/=、%=、&=、|=、~=、^=、<<=、>>= 、 >>>=、++ 和 --。
比较运算符:==、!=、<、<=、> 和 >=。
逻辑运算符:&&、|| 和 !。
位运算符:&、|、~、^、<<、>> 和 >>>。
字符串
String是拥有“值语义”的引用类型,字符串常量实现了“享元模式”,equals会按照内容进行比较,==按照地址比较。
1 public class Program {
2
3 /**
4 * @param args
5 */
6 public static void main(String[] args) {
7 String x = "段光伟";
8 String y = new String("段光伟");
9
10 System.out.println(x.equals(y)); // true
11 System.out.println(x == y); // false
12 }
13
14 }
为了高效的修改字符串Java引入了StringBuffer。
1 {
2 StringBuffer sb =
3 new StringBuffer()
4 .append("段")
5 .append("光")
6 .append("伟");
7
8 System.out.println(sb.toString());
9 }
数组
声明语法
DataType[] name 或 DataType name[]。
初始化语法
DataType[] name = new DataType[length]。
DataType[] name = new DataType[] { element1, element2, ...elementn }。
DataType[] name = { element1, element2, ...elementn }。
1 public class Program {
2
3 /**
4 * @param args
5 */
6 public static void main(String[] args) {
7 {
8 String[] strs = { "段", "光", "伟" };
9
10 for (String item : strs) {
11 System.out.print(item);
12 }
13 }
14 }
15
16 }
多维数组
只有不等长多维数组DataType[][],没有DataType[xxx, xxx]。
控制结构
条件:if-else if-else、switch-case-default和三元运算符(?:)。
循环:while、do-while、for和foreach。
Labeled block。
1 public class Program {
2
3 /**
4 * @param args
5 */
6 public static void main(String[] args) {
7 task: {
8 int age = 25;
9
10 System.out.println("start");
11
12 if (age < 30) {
13 break task;
14 }
15
16 System.out.println("end");
17 }
18 }
19 }
最近觉得label是个不错的东西,最起码多了一种选择。
方法
Java中所有的赋值和方法调用都是“按值“处理的,引用类型的值是对象的地址,原始类型的值是其自身。
Java支持变长方法参数。
1 public class Program {
2
3 /**
4 * @param args
5 */
6 public static void main(String[] args) {
7 print("段光伟", "段光宇");
8 print(new String[] { "段光伟", "段光宇" });
9 }
10
11 private static void print(String... args) {
12 for (String item : args) {
13 System.out.println(item);
14 }
15 }
16 }
类
1 public class Program {
2
3 /**
4 * @param args
5 */
6 public static void main(String[] args) {
7 Point point = new Point(100);
8
9 System.out.print(point);
10 }
11 }
12
13 class Point {
14 private int x = 0;
15 private int y = 0;
16
17 public Point(int x, int y) {
18 this.x = x;
19 this.y = y;
20 }
21
22 public Point(int x) {
23 this(x, x);
24 }
25
26 public String toString() {
27 return "(x:" + this.x + ",y:" + this.y + ")";
28 }
29 }
注意:调用自身的构造方法是用this(xxx,xxx,...)来完成,且必须位于第一行。
静态成员
Java中类似静态构造方法的结构,称之为:静态初始化代码块,与之对应的是实例初始化代码块,见下例:
1 public class Program {
2
3 /**
4 * @param args
5 */
6 public static void main(String[] args) {
7 System.out.println(Point.getValue());
8 System.out.println(new Point());
9 }
10 }
11
12 class Point {
13 private static int value = 0;
14
15 public static int getValue() {
16 return value;
17 }
18
19 static {
20 value++;
21 }
22
23 static {
24 value++;
25 }
26
27 private int x = 0;
28 private int y = 0;
29
30 {
31 this.x = 10;
32 }
33
34 {
35 this.y = 10;
36 }
37
38 public String toString() {
39 return "(x:" + this.x + ",y:" + this.y + ")";
40 }
41 }
继承
继承使用 extends,抽象类和抽象方法使用abstract声明,向下转型使用 (ChildType)instance,判断是否是某个类型使用 instanceof,见下例:
1 public class Program {
2
3 /**
4 * @param args
5 */
6 public static void main(String[] args) {
7 printAnimal(new Animal());
8 printAnimal(new Dog());
9 }
10
11 private static void printAnimal(Animal animal) {
12 if(animal instanceof Dog){
13 System.out.println("I am a " + (Dog) animal);
14 }
15 else
16 {
17 System.out.println("I am an " + animal);
18 }
19 }
20 }
21
22 class Animal {
23 public String toString() {
24 return "Animal";
25 }
26 }
27
28 class Dog extends Animal {
29 public String toString() {
30 return "Dog";
31 }
32 }
重写
Java中的重写规则比较灵活,具体如下:
除了 private 修饰之外的所有实例方法都可以重写,不需要显式的声明。
重写的方法为了显式的表达重写这一概念,使用 @Override进行注解。
重写的方法可以修改访问修饰符和返回类型,只要和父类的方法兼容(访问级别更高,返回类型更具体)。
可以使用final将某个方法标记为不可重写。
在构造方法中使用 super(xxx, xxx)调用父类构造方法,在常规实例方法中使用 super.method(xxx, xxx)调用父类方法。
Java不支持覆盖(new)。
1 public class Program {
2
3 /**
4 * @param args
5 */
6 public static void main(String[] args) {
7 Animal animal = new Animal();
8 Animal dog = new Dog();
9
10 animal.say();
11 dog.say();
12
13 animal.eat(animal);
14 dog.eat(dog);
15
16 System.out.println(animal.info());
17 System.out.println(dog.info());
18 }
19 }
20
21 class Animal {
22 private String name = "Animal";
23
24 protected void say() {
25 System.out.println("Animal" + " " + this.name);
26 }
27
28 public void eat(Animal food) {
29 System.out.println("Animal eat " + food);
30 }
31
32 public Object info() {
33 return "Animal";
34 }
35
36 @Override
37 public String toString() {
38 return "Animal";
39 }
40 }
41
42 class Dog extends Animal {
43 private String name = "Dog";
44
45 @Override
46 public final void say() {
47 System.out.println("Dog" + " " + this.name);
48 }
49
50 @Override
51 public final void eat(Animal food) {
52 super.eat(food);
53
54 System.out.println("Dog eated");
55 }
56
57 @Override
58 public final String info() {
59 return "Dog";
60 }
61
62 @Override
63 public final String toString() {
64 return "Dog";
65 }
66 }
包
包的名字和项目路径下的目录路径相对应,比如:项目路径为:C:\Study,有一个Java源文件位于:C:\Study\com\happyframework\study\App.java,那么App.java的包名字必须为:com.happyframework.study,且 App.java 的第一行语句必须为:package com.happyframework.study。
Java支持三种导入语法:
导入类型:import xxx.xxx.xxxClass。
导入包:import xxx.xxx.xxx.*。
导入静态成员:import static xxx.xxx.*。
1 import static util.Helper.*;
2
3 public class Program {
4
5 /**
6 * @param args
7 */
8 public static void main(String[] args) {
9 puts("段光伟");
10 }
11 }
访问级别
Java支持四种访问级别:public、private、protected 和 default(默认),类型和接口只能使用public 和 default,成员和嵌套类型可以使用所有,下面简单的解释一下 protected 和 default。
protected 修饰过的成员只能被自己、子类和同一个包里的(不包括子包)其他类型访问。
default 修改过的类型或成员只能被自己和同一个包里的(不包括子包)其他类型访问。
嵌套类
Java支持如下几种嵌套类:
nested class,定义在类型内部的类型。
static nested class,使用 static 声明的 nested class,static nested class 可以访问所有外部类的静态成员。
inner class,没有使用 static 声明的 nested class,inner class 可以访问所有外部类的实例成员,inner class 不能定义静态成员。
代码示例
1 public class Program {
2
3 /**
4 * @param args
5 */
6 public static void main(String[] args) {
7 OuterClass outer = new OuterClass();
8 OuterClass.InnerClass inner = outer.new InnerClass();
9 OuterClass.InnerClass.InnerInnerClass innerInner = inner.new InnerInnerClass();
10 outer.show();
11 inner.show();
12 innerInner.show();
13
14 OuterClass.StaticNestedClass staticNested=new OuterClass.StaticNestedClass();
15 OuterClass.StaticNestedClass.StaticNestedNestedClass staticNestedNested=new OuterClass.StaticNestedClass.StaticNestedNestedClass();
16
17 staticNested.show();
18 staticNestedNested.show();
19 }
20 }
21
22 class OuterClass {
23 int x = 1;
24 static int i = 1;
25
26 void show() {
27 System.out.println(x);
28 System.out.println(i);
29 }
30
31 class InnerClass {
32 int y = 2;
33
34 void show() {
35 System.out.println(x);
36 System.out.println(y);
37 }
38
39 class InnerInnerClass {
40 int z = 3;
41
42 void show() {
43 System.out.println(OuterClass.this.x);
44 System.out.println(y);
45 System.out.println(z);
46 }
47 }
48 }
49
50 static class StaticNestedClass {
51 static int j = 2;
52
53 void show() {
54 System.out.println(i);
55 System.out.println(j);
56 }
57
58 static class StaticNestedNestedClass {
59 static int k = 3;
60
61 void show() {
62 System.out.println(i);
63 System.out.println(j);
64 System.out.println(k);
65 }
66 }
67 }
68 }
特殊的inner class:local class
1 public class LocalClassExample {
2
3 static String staticValue = "static value";
4 String instanceValue = "instance value";
5
6 public void test() {
7
8 final String finalLocalValue = "final local value";
9
10 class LocalClass {
11 void test() {
12 System.out.println(staticValue);
13 System.out.println(instanceValue);
14 System.out.println(finalLocalValue);
15 }
16 }
17
18 LocalClass local = new LocalClass();
19 local.test();
20 }
21 }
除了inner class的规则之外,local class可以访问局部final变量,在Java8中有更多的改进。
特殊的local class:anonymous class
1 public class Program {
2
3 /**
4 * @param args
5 */
6 public static void main(String[] args) {
7 execute(new Action() {
8 @Override
9 public void execute() {
10 System.out.println("执行业务逻辑");
11 }
12 });
13 }
14
15 static void execute(Action action) {
16 System.out.println("事物开始");
17 action.execute();
18 System.out.println("事物结束");
19 }
20 }
21
22 interface Action {
23 void execute();
24 }
常量
不废话了,直接看代码:
1 public final class Program {
2 static final String STATIC_CONSTANTS = "STATIC_CONSTANTS";
3 final String INSTANCE_CONSTANTS = "INSTANCE_CONSTANTS";
4
5 public static void main(String[] args) {
6 final String LOCAL_CONSTANTS = "LOCAL_CONSTANTS";
7
8 System.out.println(STATIC_CONSTANTS);
9 System.out.println(new Program().INSTANCE_CONSTANTS);
10 System.out.println(LOCAL_CONSTANTS);
11 new Program().test("PARAMETER_CONSTANTS");
12 }
13
14 public final void test(final String msg) {
15 System.out.println(msg);
16 }
17 }
有一点需要注意的是:只有一种情况Java的常量是编译时常量(编译器会帮你替换),其它情况都是运行时常量,这种情况是:静态类型常量且常量的值可以编译时确定。
接口
Java的接口可以包含方法签名、常量和嵌套类,见下例:
1 public final class Program {
2 public static void main(String[] args) {
3 Playable.EMPTY.play();
4
5 new Dog().play();
6 }
7 }
8
9 interface Playable {
10 Playable EMPTY = new EmptyPlayable();
11
12 void play();
13
14 class EmptyPlayable implements Playable {
15
16 @Override
17 public void play() {
18 System.out.println("无所事事");
19 }
20
21 }
22 }
23
24 class Dog implements Playable {
25
26 @Override
27 public void play() {
28 System.out.println("啃骨头");
29 }
30
31 }
枚举
Java枚举是class,继承自java.lang.Enum,枚举中可以定义任何类型可以定义的内容,构造方法只能是private或package private,枚举成员会被编译器动态翻译为枚举实例常量,见下例:
1 public final class Program {
2 public static void main(String[] args) {
3 System.out.println(State.ON);
4 System.out.println(State.OFF);
5
6 for (State item : State.values()) {
7 System.out.println(item);
8 System.out.println(State.valueOf(item.name()));
9 }
10 }
11 }
12
13 enum State {
14 ON(1), OFF(0);
15
16 int value = 1;
17
18 State(int value) {
19 this.value = value;
20 }
21 }
调用枚举的构造方法格式是:常量名字(xxx, xxx),如果构造方法没有参数只需要:常量名子,如:
1 enum State {
2 ON, OFF
3 }
异常
Java中的异常分为checked和unchecked,checked异常必须声明在方法中或被捕获,这点我觉得比较好,必定:异常也是API的一部分,见下例:
1 public final class Program {
2 public static void main(String[] args) {
3 try {
4 test();
5 } catch (Exception e) {
6 System.out.println(e.getMessage());
7 }
8 }
9
10 public static void test() throws Exception {
11 throw new Exception("I am wrong!");
12 }
13 }
所有继承Exception的异常(除了RuntimeException和它的后代之外)都是checked异常。
装箱和拆箱
Java提供了原始类型对应的引用类型,在1.5之后的版本还提供了自动装箱和自动拆箱,结合最新版本的泛型,几乎可以忽略这块。
1 import java.util.*;
2
3 public final class Program {
4 public static void main(String[] args) {
5 ArrayList list = new ArrayList();
6
7 list.add(1);
8 int item1 = (Integer) list.get(0);
9
10 System.out.println(item1);
11 }
12 }
注意:自动装箱和自动拆箱是Java提供的语法糖。
泛型
Java的泛型是编译器提供的语法糖,官方称之为:类型参数搽除,先看一下语法,然后总结一点规律:
泛型方法
测试代码
1 static <T> void puts(T msg) {
2 println(msg);
3 }
4
5 static void println(Object msg) {
6 System.out.println("Object:" + msg);
7 }
8
9 static void println(String msg) {
10 System.out.println("String:" + msg);
11 }
调用泛型方法
1 System.out.println("generic method test");
2 puts("hello");
3 Program.<String> puts("hello");
输出的结果是
1 generic method test
2 Object:hello
3 Object:hello
泛型类
测试代码
1 class TestGenericClass<T> {
2 T value;
3
4 void setValue(T value) {
5 this.value = value;
6 }
7 }
调用代码
1 System.out.println("generic class test");
2 System.out.println(t.value);
输出结果
1 generic class test
2 1
泛型接口
测试代码
1 interface TestInterface<T> {
2 void test(T item);
3 }
4
5 class TestInterfaceImp1 implements TestInterface<String> {
6
7 @Override
8 public void test(String item) {
9 System.out.println(item);
10 }
11 }
12
13 class TestInterfaceImp2<T> implements TestInterface<T> {
14
15 @Override
16 public void test(T item) {
17 System.out.println(item);
18 }
19 }
调用代码
1 System.out.println("generic interface test");
2 TestInterface<String> testInterface1 = new TestInterfaceImp1();
3 testInterface1.test("hi");
4 for (Method item : testInterface1.getClass().getMethods()) {
5 if (item.getName() == "test") {
6 System.out.println(item.getParameterTypes()[0].getName());
7 }
8 }
9
10 TestInterface<String> testInterface2 = new TestInterfaceImp2<>();
11 testInterface2.test("hi");
12 for (Method item : testInterface2.getClass().getMethods()) {
13 if (item.getName() == "test") {
14 System.out.println(item.getParameterTypes()[0].getName());
15 }
16 }
输出结果
1 generic interface test
2 hi
3 java.lang.String
4 java.lang.Object
5 hi
6 java.lang.Object
类型参数约束
测试代码
1 class Animal {
2 }
3
4 class Dog extends Animal {
5 }
6
7 class Base<T extends Animal> {
8 public void test(T item) {
9 System.out.println("Base:" + item);
10 }
11 }
12
13 class Child extends Base<Dog> {
14
15 @Override
16 public void test(Dog item) {
17 System.out.println("Child:" + item);
18 }
19 }
调用代码
1 System.out.println("bounded type parameters test");
2 Base<Dog> base = new Child();
3 base.test(new Dog());
4 for (Method item : base.getClass().getMethods()) {
5 if (item.getName() == "test") {
6 System.out.println(item.getParameterTypes()[0].getName());
7 }
8 }
输出结果
1 bounded type parameters test
2 Child:Dog@533c2ac3
3 Dog
4 Animal
类型搽除过程
将泛型定义中的类型参数去掉。
class Base {
public void test(T item) {
System.out.println("Base:" + item);
}
}
将T换成extends指定的约束类型,默认是Object。
1 class Base {
2 public void test(Animal item) {
3 System.out.println("Base:" + item);
4 }
5 }
如果有非泛型类型继承或实现了泛型基类或接口,而且进行了重写,根据情况,编译器会自动生成一些方法。
1 class Child extends Base {
2 @Override
3 public void test(Animal item) {
4 this.test((Dog)item);
5 }
6
7 public void test(Dog item) {
8 System.out.println("Child:" + item);
9 }
10 }
根据泛型参数的实际参数搽除调用代码。
1 System.out.println("bounded type parameters test");
2 Base base = new Child();
3 base.test(new Dog());
4 for (Method item : base.getClass().getMethods()) {
5 if (item.getName() == "test") {
6 System.out.println(item.getParameterTypes()[0].getName());
7 }
8 }
这里说的不一定正确,特别是Java泛型的约束支持&(如:可以约束实行多个接口),不过过程估计差别不大,我没有看Java语言规范,这里只是大概的猜测。
备注
这几天完成了Java基本语法的学习,关于一些高级特性在后面再慢慢总结,如:运行时进程模型、类型加载机制、反射、注解、动态代理等。
源代码组织方式
Java程序由package+class组成,package对应目录的相对路径,class对应文件,如
E:\Workspaces\MyEclipse 10\JavaStudy\src\com\happyframework\javastudy\hello\Hello.java
1 package com.happyframework.javastudy.hello;
2
3 public final class Hello {
4 public static void hello(){
5 System.out.println("hello!");
6 }
7 }
关于class有如下几点规则:
文件的名字必须和class的名字一致(public级别的class名字)。
文件必须只包含一个public访问基本的class(可以包含多个非public级别的class)。
package名字必须和目录一致。
入口方法
App.java
1 public class App {
2 public static void main(String[] args) {
3 com.happyframework.javastudy.hello.Hello.hello();
4 }
5 }
最终的项目结构
数据类型
8种原子类型
整数类型:byte、short、int和long。
小数类型:float和double。
字符类型:char。
布尔类型:bool。
除此之外的是interface、class和array。
小数类型的常量默认是double类型,声明float类型的常量需要使用F作为后缀。
1 public class Program {
2
3 /**
4 * @param args
5 */
6 public static void main(String[] args) {
7 float age = 28.0F;
8 System.out.println(age);
9 }
10
11 }
运算符
算术运算符:+、-、*、/ 和 %,两个整数相除,结果还是整数。
赋值运算符:=、+=、-=、*=、/=、%=、&=、|=、~=、^=、<<=、>>= 、 >>>=、++ 和 --。
比较运算符:==、!=、<、<=、> 和 >=。
逻辑运算符:&&、|| 和 !。
位运算符:&、|、~、^、<<、>> 和 >>>。
字符串
String是拥有“值语义”的引用类型,字符串常量实现了“享元模式”,equals会按照内容进行比较,==按照地址比较。
1 public class Program {
2
3 /**
4 * @param args
5 */
6 public static void main(String[] args) {
7 String x = "段光伟";
8 String y = new String("段光伟");
9
10 System.out.println(x.equals(y)); // true
11 System.out.println(x == y); // false
12 }
13
14 }
为了高效的修改字符串Java引入了StringBuffer。
1 {
2 StringBuffer sb =
3 new StringBuffer()
4 .append("段")
5 .append("光")
6 .append("伟");
7
8 System.out.println(sb.toString());
9 }
数组
声明语法
DataType[] name 或 DataType name[]。
初始化语法
DataType[] name = new DataType[length]。
DataType[] name = new DataType[] { element1, element2, ...elementn }。
DataType[] name = { element1, element2, ...elementn }。
1 public class Program {
2
3 /**
4 * @param args
5 */
6 public static void main(String[] args) {
7 {
8 String[] strs = { "段", "光", "伟" };
9
10 for (String item : strs) {
11 System.out.print(item);
12 }
13 }
14 }
15
16 }
多维数组
只有不等长多维数组DataType[][],没有DataType[xxx, xxx]。
控制结构
条件:if-else if-else、switch-case-default和三元运算符(?:)。
循环:while、do-while、for和foreach。
Labeled block。
1 public class Program {
2
3 /**
4 * @param args
5 */
6 public static void main(String[] args) {
7 task: {
8 int age = 25;
9
10 System.out.println("start");
11
12 if (age < 30) {
13 break task;
14 }
15
16 System.out.println("end");
17 }
18 }
19 }
最近觉得label是个不错的东西,最起码多了一种选择。
方法
Java中所有的赋值和方法调用都是“按值“处理的,引用类型的值是对象的地址,原始类型的值是其自身。
Java支持变长方法参数。
1 public class Program {
2
3 /**
4 * @param args
5 */
6 public static void main(String[] args) {
7 print("段光伟", "段光宇");
8 print(new String[] { "段光伟", "段光宇" });
9 }
10
11 private static void print(String... args) {
12 for (String item : args) {
13 System.out.println(item);
14 }
15 }
16 }
类
1 public class Program {
2
3 /**
4 * @param args
5 */
6 public static void main(String[] args) {
7 Point point = new Point(100);
8
9 System.out.print(point);
10 }
11 }
12
13 class Point {
14 private int x = 0;
15 private int y = 0;
16
17 public Point(int x, int y) {
18 this.x = x;
19 this.y = y;
20 }
21
22 public Point(int x) {
23 this(x, x);
24 }
25
26 public String toString() {
27 return "(x:" + this.x + ",y:" + this.y + ")";
28 }
29 }
注意:调用自身的构造方法是用this(xxx,xxx,...)来完成,且必须位于第一行。
静态成员
Java中类似静态构造方法的结构,称之为:静态初始化代码块,与之对应的是实例初始化代码块,见下例:
1 public class Program {
2
3 /**
4 * @param args
5 */
6 public static void main(String[] args) {
7 System.out.println(Point.getValue());
8 System.out.println(new Point());
9 }
10 }
11
12 class Point {
13 private static int value = 0;
14
15 public static int getValue() {
16 return value;
17 }
18
19 static {
20 value++;
21 }
22
23 static {
24 value++;
25 }
26
27 private int x = 0;
28 private int y = 0;
29
30 {
31 this.x = 10;
32 }
33
34 {
35 this.y = 10;
36 }
37
38 public String toString() {
39 return "(x:" + this.x + ",y:" + this.y + ")";
40 }
41 }
继承
继承使用 extends,抽象类和抽象方法使用abstract声明,向下转型使用 (ChildType)instance,判断是否是某个类型使用 instanceof,见下例:
1 public class Program {
2
3 /**
4 * @param args
5 */
6 public static void main(String[] args) {
7 printAnimal(new Animal());
8 printAnimal(new Dog());
9 }
10
11 private static void printAnimal(Animal animal) {
12 if(animal instanceof Dog){
13 System.out.println("I am a " + (Dog) animal);
14 }
15 else
16 {
17 System.out.println("I am an " + animal);
18 }
19 }
20 }
21
22 class Animal {
23 public String toString() {
24 return "Animal";
25 }
26 }
27
28 class Dog extends Animal {
29 public String toString() {
30 return "Dog";
31 }
32 }
重写
Java中的重写规则比较灵活,具体如下:
除了 private 修饰之外的所有实例方法都可以重写,不需要显式的声明。
重写的方法为了显式的表达重写这一概念,使用 @Override进行注解。
重写的方法可以修改访问修饰符和返回类型,只要和父类的方法兼容(访问级别更高,返回类型更具体)。
可以使用final将某个方法标记为不可重写。
在构造方法中使用 super(xxx, xxx)调用父类构造方法,在常规实例方法中使用 super.method(xxx, xxx)调用父类方法。
Java不支持覆盖(new)。
1 public class Program {
2
3 /**
4 * @param args
5 */
6 public static void main(String[] args) {
7 Animal animal = new Animal();
8 Animal dog = new Dog();
9
10 animal.say();
11 dog.say();
12
13 animal.eat(animal);
14 dog.eat(dog);
15
16 System.out.println(animal.info());
17 System.out.println(dog.info());
18 }
19 }
20
21 class Animal {
22 private String name = "Animal";
23
24 protected void say() {
25 System.out.println("Animal" + " " + this.name);
26 }
27
28 public void eat(Animal food) {
29 System.out.println("Animal eat " + food);
30 }
31
32 public Object info() {
33 return "Animal";
34 }
35
36 @Override
37 public String toString() {
38 return "Animal";
39 }
40 }
41
42 class Dog extends Animal {
43 private String name = "Dog";
44
45 @Override
46 public final void say() {
47 System.out.println("Dog" + " " + this.name);
48 }
49
50 @Override
51 public final void eat(Animal food) {
52 super.eat(food);
53
54 System.out.println("Dog eated");
55 }
56
57 @Override
58 public final String info() {
59 return "Dog";
60 }
61
62 @Override
63 public final String toString() {
64 return "Dog";
65 }
66 }
包
包的名字和项目路径下的目录路径相对应,比如:项目路径为:C:\Study,有一个Java源文件位于:C:\Study\com\happyframework\study\App.java,那么App.java的包名字必须为:com.happyframework.study,且 App.java 的第一行语句必须为:package com.happyframework.study。
Java支持三种导入语法:
导入类型:import xxx.xxx.xxxClass。
导入包:import xxx.xxx.xxx.*。
导入静态成员:import static xxx.xxx.*。
1 import static util.Helper.*;
2
3 public class Program {
4
5 /**
6 * @param args
7 */
8 public static void main(String[] args) {
9 puts("段光伟");
10 }
11 }
访问级别
Java支持四种访问级别:public、private、protected 和 default(默认),类型和接口只能使用public 和 default,成员和嵌套类型可以使用所有,下面简单的解释一下 protected 和 default。
protected 修饰过的成员只能被自己、子类和同一个包里的(不包括子包)其他类型访问。
default 修改过的类型或成员只能被自己和同一个包里的(不包括子包)其他类型访问。
嵌套类
Java支持如下几种嵌套类:
nested class,定义在类型内部的类型。
static nested class,使用 static 声明的 nested class,static nested class 可以访问所有外部类的静态成员。
inner class,没有使用 static 声明的 nested class,inner class 可以访问所有外部类的实例成员,inner class 不能定义静态成员。
代码示例
1 public class Program {
2
3 /**
4 * @param args
5 */
6 public static void main(String[] args) {
7 OuterClass outer = new OuterClass();
8 OuterClass.InnerClass inner = outer.new InnerClass();
9 OuterClass.InnerClass.InnerInnerClass innerInner = inner.new InnerInnerClass();
10 outer.show();
11 inner.show();
12 innerInner.show();
13
14 OuterClass.StaticNestedClass staticNested=new OuterClass.StaticNestedClass();
15 OuterClass.StaticNestedClass.StaticNestedNestedClass staticNestedNested=new OuterClass.StaticNestedClass.StaticNestedNestedClass();
16
17 staticNested.show();
18 staticNestedNested.show();
19 }
20 }
21
22 class OuterClass {
23 int x = 1;
24 static int i = 1;
25
26 void show() {
27 System.out.println(x);
28 System.out.println(i);
29 }
30
31 class InnerClass {
32 int y = 2;
33
34 void show() {
35 System.out.println(x);
36 System.out.println(y);
37 }
38
39 class InnerInnerClass {
40 int z = 3;
41
42 void show() {
43 System.out.println(OuterClass.this.x);
44 System.out.println(y);
45 System.out.println(z);
46 }
47 }
48 }
49
50 static class StaticNestedClass {
51 static int j = 2;
52
53 void show() {
54 System.out.println(i);
55 System.out.println(j);
56 }
57
58 static class StaticNestedNestedClass {
59 static int k = 3;
60
61 void show() {
62 System.out.println(i);
63 System.out.println(j);
64 System.out.println(k);
65 }
66 }
67 }
68 }
特殊的inner class:local class
1 public class LocalClassExample {
2
3 static String staticValue = "static value";
4 String instanceValue = "instance value";
5
6 public void test() {
7
8 final String finalLocalValue = "final local value";
9
10 class LocalClass {
11 void test() {
12 System.out.println(staticValue);
13 System.out.println(instanceValue);
14 System.out.println(finalLocalValue);
15 }
16 }
17
18 LocalClass local = new LocalClass();
19 local.test();
20 }
21 }
除了inner class的规则之外,local class可以访问局部final变量,在Java8中有更多的改进。
特殊的local class:anonymous class
1 public class Program {
2
3 /**
4 * @param args
5 */
6 public static void main(String[] args) {
7 execute(new Action() {
8 @Override
9 public void execute() {
10 System.out.println("执行业务逻辑");
11 }
12 });
13 }
14
15 static void execute(Action action) {
16 System.out.println("事物开始");
17 action.execute();
18 System.out.println("事物结束");
19 }
20 }
21
22 interface Action {
23 void execute();
24 }
常量
不废话了,直接看代码:
1 public final class Program {
2 static final String STATIC_CONSTANTS = "STATIC_CONSTANTS";
3 final String INSTANCE_CONSTANTS = "INSTANCE_CONSTANTS";
4
5 public static void main(String[] args) {
6 final String LOCAL_CONSTANTS = "LOCAL_CONSTANTS";
7
8 System.out.println(STATIC_CONSTANTS);
9 System.out.println(new Program().INSTANCE_CONSTANTS);
10 System.out.println(LOCAL_CONSTANTS);
11 new Program().test("PARAMETER_CONSTANTS");
12 }
13
14 public final void test(final String msg) {
15 System.out.println(msg);
16 }
17 }
有一点需要注意的是:只有一种情况Java的常量是编译时常量(编译器会帮你替换),其它情况都是运行时常量,这种情况是:静态类型常量且常量的值可以编译时确定。
接口
Java的接口可以包含方法签名、常量和嵌套类,见下例:
1 public final class Program {
2 public static void main(String[] args) {
3 Playable.EMPTY.play();
4
5 new Dog().play();
6 }
7 }
8
9 interface Playable {
10 Playable EMPTY = new EmptyPlayable();
11
12 void play();
13
14 class EmptyPlayable implements Playable {
15
16 @Override
17 public void play() {
18 System.out.println("无所事事");
19 }
20
21 }
22 }
23
24 class Dog implements Playable {
25
26 @Override
27 public void play() {
28 System.out.println("啃骨头");
29 }
30
31 }
枚举
Java枚举是class,继承自java.lang.Enum,枚举中可以定义任何类型可以定义的内容,构造方法只能是private或package private,枚举成员会被编译器动态翻译为枚举实例常量,见下例:
1 public final class Program {
2 public static void main(String[] args) {
3 System.out.println(State.ON);
4 System.out.println(State.OFF);
5
6 for (State item : State.values()) {
7 System.out.println(item);
8 System.out.println(State.valueOf(item.name()));
9 }
10 }
11 }
12
13 enum State {
14 ON(1), OFF(0);
15
16 int value = 1;
17
18 State(int value) {
19 this.value = value;
20 }
21 }
调用枚举的构造方法格式是:常量名字(xxx, xxx),如果构造方法没有参数只需要:常量名子,如:
1 enum State {
2 ON, OFF
3 }
异常
Java中的异常分为checked和unchecked,checked异常必须声明在方法中或被捕获,这点我觉得比较好,必定:异常也是API的一部分,见下例:
1 public final class Program {
2 public static void main(String[] args) {
3 try {
4 test();
5 } catch (Exception e) {
6 System.out.println(e.getMessage());
7 }
8 }
9
10 public static void test() throws Exception {
11 throw new Exception("I am wrong!");
12 }
13 }
所有继承Exception的异常(除了RuntimeException和它的后代之外)都是checked异常。
装箱和拆箱
Java提供了原始类型对应的引用类型,在1.5之后的版本还提供了自动装箱和自动拆箱,结合最新版本的泛型,几乎可以忽略这块。
1 import java.util.*;
2
3 public final class Program {
4 public static void main(String[] args) {
5 ArrayList list = new ArrayList();
6
7 list.add(1);
8 int item1 = (Integer) list.get(0);
9
10 System.out.println(item1);
11 }
12 }
注意:自动装箱和自动拆箱是Java提供的语法糖。
泛型
Java的泛型是编译器提供的语法糖,官方称之为:类型参数搽除,先看一下语法,然后总结一点规律:
泛型方法
测试代码
1 static <T> void puts(T msg) {
2 println(msg);
3 }
4
5 static void println(Object msg) {
6 System.out.println("Object:" + msg);
7 }
8
9 static void println(String msg) {
10 System.out.println("String:" + msg);
11 }
调用泛型方法
1 System.out.println("generic method test");
2 puts("hello");
3 Program.<String> puts("hello");
输出的结果是
1 generic method test
2 Object:hello
3 Object:hello
泛型类
测试代码
1 class TestGenericClass<T> {
2 T value;
3
4 void setValue(T value) {
5 this.value = value;
6 }
7 }
调用代码
1 System.out.println("generic class test");
2 System.out.println(t.value);
输出结果
1 generic class test
2 1
泛型接口
测试代码
1 interface TestInterface<T> {
2 void test(T item);
3 }
4
5 class TestInterfaceImp1 implements TestInterface<String> {
6
7 @Override
8 public void test(String item) {
9 System.out.println(item);
10 }
11 }
12
13 class TestInterfaceImp2<T> implements TestInterface<T> {
14
15 @Override
16 public void test(T item) {
17 System.out.println(item);
18 }
19 }
调用代码
1 System.out.println("generic interface test");
2 TestInterface<String> testInterface1 = new TestInterfaceImp1();
3 testInterface1.test("hi");
4 for (Method item : testInterface1.getClass().getMethods()) {
5 if (item.getName() == "test") {
6 System.out.println(item.getParameterTypes()[0].getName());
7 }
8 }
9
10 TestInterface<String> testInterface2 = new TestInterfaceImp2<>();
11 testInterface2.test("hi");
12 for (Method item : testInterface2.getClass().getMethods()) {
13 if (item.getName() == "test") {
14 System.out.println(item.getParameterTypes()[0].getName());
15 }
16 }
输出结果
1 generic interface test
2 hi
3 java.lang.String
4 java.lang.Object
5 hi
6 java.lang.Object
类型参数约束
测试代码
1 class Animal {
2 }
3
4 class Dog extends Animal {
5 }
6
7 class Base<T extends Animal> {
8 public void test(T item) {
9 System.out.println("Base:" + item);
10 }
11 }
12
13 class Child extends Base<Dog> {
14
15 @Override
16 public void test(Dog item) {
17 System.out.println("Child:" + item);
18 }
19 }
调用代码
1 System.out.println("bounded type parameters test");
2 Base<Dog> base = new Child();
3 base.test(new Dog());
4 for (Method item : base.getClass().getMethods()) {
5 if (item.getName() == "test") {
6 System.out.println(item.getParameterTypes()[0].getName());
7 }
8 }
输出结果
1 bounded type parameters test
2 Child:Dog@533c2ac3
3 Dog
4 Animal
类型搽除过程
将泛型定义中的类型参数去掉。
class Base {
public void test(T item) {
System.out.println("Base:" + item);
}
}
将T换成extends指定的约束类型,默认是Object。
1 class Base {
2 public void test(Animal item) {
3 System.out.println("Base:" + item);
4 }
5 }
如果有非泛型类型继承或实现了泛型基类或接口,而且进行了重写,根据情况,编译器会自动生成一些方法。
1 class Child extends Base {
2 @Override
3 public void test(Animal item) {
4 this.test((Dog)item);
5 }
6
7 public void test(Dog item) {
8 System.out.println("Child:" + item);
9 }
10 }
根据泛型参数的实际参数搽除调用代码。
1 System.out.println("bounded type parameters test");
2 Base base = new Child();
3 base.test(new Dog());
4 for (Method item : base.getClass().getMethods()) {
5 if (item.getName() == "test") {
6 System.out.println(item.getParameterTypes()[0].getName());
7 }
8 }
这里说的不一定正确,特别是Java泛型的约束支持&(如:可以约束实行多个接口),不过过程估计差别不大,我没有看Java语言规范,这里只是大概的猜测。