文章目录
DOM基础
1、操作DOM的步骤
1)通过选择器找到DOM节点
2)修改DOM节点:
a、修改HTML
b、修改属性:基本属性、样式、事件
c、修改节点结构
2、选择器
1)通过选择器找到DOM节点
举例说明:
<body>
<div id="loginBox" class="box">
</div>
</body>
/**
* id选择器 元素选择器 类选择器
* **/
var divBox = document.getElementById('loginBox');//ID选择器
var divBox = document.getElementsByTagName('div')[0];//元素选择器
var divBox = document.getElementsByClassName('box')[0];//类选择器
divBox.innerHTML='hello';
alert(divBox.innerHTML);//alert不属于DOM
2)应用
a、UI举例1:左侧导航菜单
<body>
<h6 id="menu">系统管理</h6>
<div id="loginBox" class="box">
1、用户管理
2、权限管理
3、。。。。。
</div>
<!--
display的属性值:block inline inline-block none
-->
</body>
var menu = document.getElementById('menu');
menu.onclick = toggle;
function toggle(){
var loginBox = document.getElementById('loginBox');
var boxDisplay = loginBox.style.display;
if(boxDisplay=='none'){
show();
}else{
hide();
}
}
function hide() {
var loginBox = document.getElementById('loginBox');
loginBox.style.display = 'none';
}
function show() {
var loginBox = document.getElementById('loginBox');
loginBox.style.display = 'block';
}
3、修改DOM节点的属性
1)使用css对应的style属性
<script>
var divBox = document.getElementById("div");//获取元素id
divBox.style.display = "none";
</script>
2)用cssText
<script>
var div = document.getElementById("div");//获取元素id
div.style.cssText='width:600px;height:250px;border:1px red solid;
text-align: center;line-height: 250px;';
</script>
3)用seAttribute()
<div class="box" style="display: block;"></div>
<script>
var divBox = document.getElementsByClassName('box')[0];
divBox.setAttribute('style','height:100px; width:100px; background-color:red');
</script>
4)修改className
点击button之前div的样式为box
点击button后,调用check函数中的className将div的样式由box改为login-test
function check() {
var divBox = document.getElementsByClassName('box')[0];
divBox.className = 'login-test ';
}
<body>
<div class="box">
</div>
<button onclick="check()">click me</button>
</body>
.box{
width: 100px;
height: 100px;
background-color: green;
}
.login-test{
width: 200px;
height: 200px;
background-color: green;
}
5)使用classList()
function check() {
var divBox = document.getElementsByClassName('box')[0];
divBox.classList.remove('box');
divBox.classList.add('blue');
}
<body>
<div class="box">
</div>
<button onclick="check()">click on</button>
</body>
.box{
width: 100px;
height: 100px;
background-color: green;
}
.blue{
width: 100px;
height: 100px;
background-color: blue;
}
4、修改DOM节点的结构
添加组件的步骤
a、找到父容器
b、在内容创建dom节点
c、把创建的dom节点加入它对应的父容器中
举例说明:向指定div中加入一个标题
扫描二维码关注公众号,回复:
10045965 查看本文章
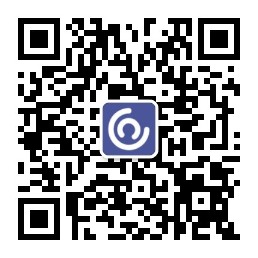
window.onload = function () {
addNodeToBox();
}
function addNodeToBox() {
//控制子元素:
//1、找到父容器;2、在内容中创建子元素;3、把子元素放到父容器中
var box = document.getElementsByClassName('box')[0];//找到父容器
//控制样式
box.classList.add('blue');
var header = document.createElement('h6');//创建子元素
//header.innerHTML='大标题1';
header.innerText='大标题(纯文本)';//设置子元素属性
box.appendChild(header);//把子元素加入到父容器中
//box.removeChild(header); 也可以删除子元素
var div1 = document.createElement('div');
div1.innerHTML='<p>hello</p>';
box.insertBefore(div1,header);//在父容器box中,把div1加到header之前
}
举例说明:创建无序列表
window.onload = function () {
addUListToBox();
}
var listItems = [
'项目1','项目2','项目3'
];
function addUListToBox() {
var box = document.getElementsByClassName('box')[0];//1、找到父容器
var uList = document.createElement('ul');//2、创建ul
box.appendChild(uList);//3、把ul加到父容器中
for(var i = 0;i<listItems.length;i++){
/**
* 1、创建li
* 2、添加li的文本
* 3、把li添加到ul中
*/
var listItem = document.createElement('li');
listItem.innerText = listItems[i];
uList.appendChild(listItem);
}
}
举例说明:利用json数组创建无序列表
json——JavaScript对象表示法
window.onload = function () {
addNewUListToBox();
}
var newItems=[{url:'test1',menuText:'项目1'},{url:'test1',menuText:'项目1'},{url:'test1',menuText:'项目1'}];
function addNewUListToBox() {
var box = document.getElementsByClassName('box')[0];//1、找到父容器
var uList = document.createElement('ul');//2、创建ul
box.appendChild(uList);//3、把ul加到父容器中
for(var i = 0;i<newItems.length;i++){/**把对象做一个抽象**/
/**
* 1、创建li
* 2、添加li的文本
* 3、把li添加到ul中
*/
var listItem = document.createElement('li');
var achor = document.createElement('a');
achor.href = newItems[i].url;
achor.innerText = newItems[i].menuText;
listItem.appendChild(achor);
uList.appendChild(listItem);
}
}
练习热身
看了上面的这些基础知识,来几道编程小题热热身吧!
1)完善左侧导航
技术要求:
- a、使用ul嵌套的方式构建左侧导航,做一个二级导航
- b、导航的具体节点使用 li 标签和 a 标签
- c、点击父节点,子节点的导航内容呈现显示、消失两种状态交替
- d、去除样式标记
<body>
<div>
<ul class="siderbar">
<li onclick="toggle(this)">模块1
<ul class="menu1">
<li>模块1-1</li>
<li>模块1-2</li>
</ul>
</li>
<li onclick="toggle(this)">模块2
<ul class="menu2">
<li>模块2-1</li>
<li>模块2-2</li>
</ul>
</li>
</ul>
</div>
</body>
window.onload = function () {
/**
* 1、找到dom节点
* 2、修改
*/
var siderbar = document.getElementsByClassName('siderbar')[0];
siderbar.style.listStyle='none';
}
function toggle(menu){
var childMenu = menu.firstElementChild;
isDisplay(childMenu);
}
function isDisplay(menu) {
if(menu.style.display==='none'){//menu1.style.display的初始值为'block'
menu.style.display='block';
}else{
menu.style.display='none';
}
}
2) 在一个div元素中 ,完成简单的添加移除DOM节点
a、添加子元素;b、移除子元素;c、在一个子元素前面再添加一个元素
代码Demo:
<head>
<meta charset="UTF-8">
<title>斗地主小游戏(练习修改DOM节点的结构)</title>
<link rel="stylesheet" href="structure.css">
</head>
<body>
<div class = "box" style="display: block;">
<p>本王在此!谁敢造次!</p>
</div>
<br>
<br>
<button onclick="addNodeToBox()">斗地主(添加子元素)</button>
<button onclick="deleteNodeFromBox()">战斗失败(移除子元素)</button>
<button onclick="addNodeBeforeOld()">战斗胜利(在一个子元素前面再添加一个元素)</button>
</body>
function addNodeToBox(){
var box = document.getElementsByClassName('box')[0];//找到父容器
box.classList.add('box');//控制样式
var header = document.createElement('h6');//创建子元素
header.innerText='叫你嚣张,削你信不信?';//设置子元素属性
box.appendChild(header);//把子元素加入到父容器中
}
function deleteNodeFromBox(){
var box = document.getElementsByClassName('box')[0];//找到父容器
var header = document.getElementsByTagName('h6')[0];
box.removeChild(header);//可以删除子元素
}
function addNodeBeforeOld(){
var box = document.getElementsByClassName('box')[0];//找到父容器
var old = document.getElementsByTagName('p')[0];
var div1 = document.createElement('div');//创建子元素
div1.classList.add('newDiv');//控制样式
div1.innerText='易主!';//设置子元素属性
box.insertBefore(div1,old);//在父容器box中,把div1加到old之前
}
.box{
width:400px;
height: 400px;
background-color: red;
}
.newDiv{
width:400px;
height: 400px;
background-color: yellow;
}
3) 使用json数组,完成动态创建导航菜单
在页面上动态创建导航菜单 (单层导航/嵌套导航)
代码Demo:
单层导航:
window.onload=function () {
addNavToBox(); //单层导航
}
var newItems=[{url:'#',menuText:'项目1'},{url:'#',menuText:'项目2'},{url:'#',menuText:'项目3'}];
function addNavToBox(){
var box = document.getElementsByClassName('box')[0];//找到父容器
var uList = document.createElement('ul');//创建ul
box.appendChild(uList);//把ul加到父容器中
for(var i=0;i<newItems.length;i++){
var listItem = document.createElement('li');//创建li
var achor = document.createElement('a');
achor.href = newItems[i].url;
achor.innerText = newItems[i].menuText;//添加li的文本
listItem.appendChild(achor);
uList.appendChild(listItem);//把li添加到ul中
}
}
<head>
<meta charset="UTF-8">
<title>动态创建导航菜单(单层导航/嵌套导航)</title>
</head>
<body>
<div class="box">
</div>
</body>
嵌套导航:
window.onload=function () {
addNestNavToBox();//嵌套导航
}
var module1=[{url:'#',menuText:'模块1-1'},{url:'#',menuText:'模块1-2'}];
var module2=[{url:'#',menuText:'模块2-1'},{url:'#',menuText:'模块2-2'}];
var module3=[{url:'#',menuText:'模块3-1'},{url:'#',menuText:'模块3-2'}];
function addNestNavToBox() {
var box = document.getElementsByClassName('box')[0];//找到父容器
var uList = document.createElement('ul');
box.appendChild(uList);//把ul加到父容器中
var moduleArray = [module1];//创建json数组
moduleArray.push(module2);
moduleArray.push(module3);
for(var i=0;i<moduleArray.length;i++){
var listItem = document.createElement('li');
listItem.innerText = "模块"+(i+1);
uList.appendChild(listItem);//把li加到ul中
var uListItem = document.createElement('ul');
listItem.appendChild(uListItem);//li中,嵌套ul元素
for(var j=0;j<moduleArray[i].length;j++){
var lListItem = document.createElement('li');
var achor = document.createElement('a');
achor.href = moduleArray[i][j].url;
achor.innerText = moduleArray[i][j].menuText;
lListItem.appendChild(achor);//li中嵌套a元素
uListItem.appendChild(lListItem);//ul中,嵌套li元素
listItem.appendChild(uListItem);//将ul嵌套进li元素
}
}
}
<head>
<meta charset="UTF-8">
<title>动态创建导航菜单(单层导航/嵌套导航)</title>
</head>
<body>
<div class="box">
</div>
</body>
4)使用json数组,动态创建隔行换色的表格
在页面上动态创建一个table,并实现隔行换色
代码Demo:
window.onload=function () {
addTableToBox();
}
function addTableToBox() {//创建一个3行5列的表格
var box = document.getElementsByClassName('box')[0];
var table = document.createElement('table');//创建table标签
box.appendChild(table);
for(var i = 0;i < 3;i++){
var tr = document.createElement('tr');
table.appendChild(tr);
for(var j = 0;j < 5;j++){
var td = document.createElement('td');
td.innerText=(i+1)+"-"+(j+1);
tr.appendChild(td);
}
}
table.classList.add('table');
}
<head>
<meta charset="UTF-8">
<title>动态创建隔行换色的表格</title>
<link rel="stylesheet" href="table.css">
</head>
<body>
<div class="box">
</div>
</body>
tr:nth-child(even){
background-color: green;
}
tr:nth-child(odd){
background-color: yellow;
}
table{
width: 400px;
line-height: 25px;
text-align: center;
border: 1px black solid;
}
总结
1、EMCAScript
编程语言基础 —— 包括 数据类型、 流程控制 、 函数
2、 DOM
文档对象模型——包括
- 选择器
- 属性——基本属性、样式事件
- 结构—— 父容器 ,dom节点(父容器、兄弟节点 )
3、数据
Json (JavaScript对象表示法)
/*json对象*/
var emp1={empName:'Mat',gender:'Male'};
/*json对象数组*/
var arr=[
{empName:'Mat',gender:'Male'},
{empName:'Green',gender:'Male'},
{empName:'Mary',gender:'Male'}
]
4、循环
1)单层循环
2)多层循环(如果出现两层以上的循环,一般认为是程序设计出现了问题——修改程序结构)