一、概述
Websocket 是 H5 自带的一个 API,随着越来越多的浏览器都自适应了 H5 的特性,许多浏览器也内置了 WebSocket API。也就是说 WebSocket 和 window、document 一样作为全局变量可以直接使用。
要在浏览器端使用 WebSocket,首先需要服务端支持 WebSocket,假设现在服务端已提供 WebSocket 服务,访问地址:ws://localhost:8080
,简单介绍下浏览器使用方法。
// 连接 Websocket 服务端
const ws = new WebSocket("ws://localhost:8080");
// 监听连接上 Websocket 服务端触发事件
ws.onopen = function (e) {
console.log('连接上 ws 服务端了');
// ws.send() 给服务端发送数据
ws.send('我是客户端,我接收到你的请求了');
}
// 监听 Websocket 服务端传来消息触发事件
ws.onmessage = function(msg) {
// msg.data 接收服务端传递过来的数据
console.log('接收服务端发过来的消息: %o', msg.data);
};
// 监听 Websocket 服务端连接断开触发事件
ws.onclose = function (e) {
console.log('ws 连接关闭了');
}
注意事项: WebSocket 通信传递的数据是字符串,即便浏览器端传给服务端的是个对象,在服务端接收时也会变成字符串,可以通过 JSON.parse(msg.data)
解析成对象。
二、dva 中使用 WebSocket
下面是我写小说爬虫用到的部分代码,点击爬取,在浏览器端打印服务端爬虫日志。
dva 是基于 React 的状态管理器,不能直接对 Dom 进行操作。要持续不断的接收服务端响应数据,需要在构造器中定义一个 state 属性进行接收。直接在 routes 目录下的页面中连接服务端 WebSocket 并调用 API 接口。
(用箭头函数写组件的写法是没有 state 特性的,这一点在 React 官方文档中有详细说明,具体可参考 React 开发中不得不注意的两个大坑)
// src/routes/novel/index.js
class NovelComp {
constructor (props) {
super(props);
this.state = { result: '' }; // 接收 Websocket 响应数据
}
handleSteal (flag) {
const ws = new WebSocket('ws://localhost:8080');
let result = this.state.result;
ws.onopen = function (e) {
console.log('连接上 ws 服务端了');
ws.send(JSON.stringify({ flag: flag, data: currentItem }));
}
ws.onmessage = function(msg) {
console.log('接收服务端发过来的消息: %o', msg);
result += msg.data + '\n';
that.setState({ result: result });
};
ws.onclose = function (e) {
console.log('ws 连接关闭了');
}
}
render () {
return (
<Modal>
<Button></Button>
....
<Modal/>
);
}
}
三、一直连接 WebSocket
上面的 WebSocket 应用场景是点击按钮才会连接 WebSocket,关闭模态框 WebSocket 连接即断开。
有些需求需要 WebSocket 一直连接,比如 待办事项主动提醒
。如果其它操作新增了一条通知,当前用户的通知条目应当自动变成 6,而不是下一次刷新完再更新。
由于 dva 本身的特性,如果刷新页面,dva 所有状态容器中的值都会清空,与此同时 WebSocket 连接也会断开,也就没法监听服务端传过来的数据。
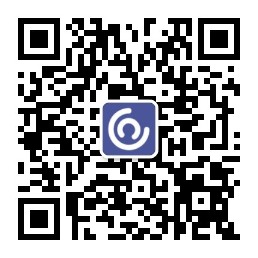
解决方案: 修改 src/models/novel.js 的 subscriptions 属性。
subscriptions: {
setupHistory ({ dispatch, history }) {
history.listen((location) => {
if (location.pathname.indexOf('reptile/novel')) {
const ws = new WebSocket('ws://localhost:8080');
dispatch({
type: 'updateState',
payload: { ws: ws },
});
}
})
},
},
subscriptions 下面 setupHistory 的作用是监听浏览器地址栏地址如果变成当前页面 reptile/novel
就创建 WebSocket 连接,这样就能保证每次进入这个页面的时候都已连接 WebSocket。
对于 待办事项主动提醒
那个需求,只需在布局文件中的 subscriptions 中添加上述代码即可。