管理页面
- 创建html
- 添加整体架构
- ajax表单验证
- 读取数据库数据
第一步:创建indexpage.html
<!doctype html>
<html lang="zh">
<script src="js/loginJS.js"></script>
<script src="js/searchJS.js"></script>
<script src="js/jquery-1.7.1.min.js"></script>
<link rel="stylesheet" href="css/bootstrap-3.3.7.min.css">
<script src="js/bootstrap-3.3.7.min.js"></script>
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>整体架构</title>
<!--<link rel="stylesheet" type="text/css" href="css/styles.css">-->
</head>
<body>
<nav class="navbar navbar-default">
<div class="container-fluid">
<div class="navbar-header">
<a href="#" class="navbar-brand">图书管理系统</a>
</div>
<div class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<li class="dropdown">
<a href="#" id="username" class="dropdown-toggle" data-toggle="dropdown">
<script type="text/javascript">
online();
</script>
</a>
<ul class="dropdown-menu">
<li><a href="#">修改密码</a></li>
<li><a href="#">借阅记录</a></li>
<li><a href="#">退出登录</a></li>
</ul>
</li>
</ul>
<div class="navbar-form navbar-left">
<div class="form-group">
<input type="text" id="searchTxt" class="form-control" placeholder="Search">
</div>
<button type="submit" class="btn btn-default" id="search_bt" onclick="search()"/>Search</div>
</div>
</div>
</div>
</nav>
<div class="form-group">
<table id="postTable" class="table table-striped">
<tr style="font-weight: bold">
<td>书名</td>
<td>作者</td>
<td>出版社</td>
<td>借阅状态</td>
</tr>
</table>
</div>
</body>
第二步:创建searchJS.js
function search() {
var bookName = document.getElementById("searchTxt");
var data = {};
data["bookName"] = bookName.value;
$.ajax({
url:"search",
type:"POST",
data:data,
dataType:"JSON",
success:function (result) {
console.log(result);
$("#postTable").html("<tr style=\"font-weight:bold\"><td>书名</td><td>作者</td><td>出版社</td><td>借阅状态</td><td>一键借阅</td></tr>");
$.each(result, function (i, item) {
var isrent;
if(item.isrent == 0){
isrent = "在架上";
$("#postTable").append(
'<tr><td>'+item.bookName+'</td><td>'+item.bookWriter+'</td><td>'+item.Publisher+'</td><td>'+isrent+'</td><td><a class="btn btn-primary btn-xs" href="#" role="button" id="'+item.bookId+'" onclick="borrowBook(this)">借阅</a></td></tr>'
);
}else{
isrent = "已借出";
$("#postTable").append(
'<tr><td>'+item.bookName+'</td><td>'+item.bookWriter+'</td><td>'+item.Publisher+'</td><td>'+isrent + '</td><td><a class="btn btn-primary btn-xs" href="#" disabled="true" role="button" id="'+item.bookId+'">借阅</a></td></tr>'
);
}
})
},
error:function () {
alert("请求出错!")
}
});
}
第三步:修改BookMapper.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.ray.dao.BookMapper">
<resultMap id="BaseResultMap" type="com.ray.entity.Book">
<id column="id" jdbcType="BIGINT" property="id" />
<result column="book_name" jdbcType="VARCHAR" property="bookName" />
<result column="book_writer" jdbcType="VARCHAR" property="bookWriter" />
<result column="book_publisher" jdbcType="VARCHAR" property="bookPublisher" />
<result column="book_isrent" jdbcType="BIGINT" property="bookIsrent" />
<result column="book_person" jdbcType="VARCHAR" property="bookPerson" />
</resultMap>
<sql id="Base_Column_List">
id, book_name, book_writer, book_publisher, book_isrent, book_person
</sql>
<select id="selectByPrimaryKey" parameterType="java.lang.Long" resultMap="BaseResultMap">
select
<include refid="Base_Column_List" />
from book
where id = #{id,jdbcType=BIGINT}
</select>
<!-- 模糊查询 -->
<select id="selectBookByName" parameterType="com.ray.entity.Book" resultMap="BaseResultMap">
select
<include refid="Base_Column_List"/>
from book
where book_name like concat('%',#{bookName},'%')
</select>
<delete id="deleteByPrimaryKey" parameterType="java.lang.Long">
delete from book
where id = #{id,jdbcType=BIGINT}
</delete>
<insert id="insert" parameterType="com.ray.entity.Book">
insert into book (id, book_name, book_writer,
book_publisher, book_isrent, book_person
)
values (#{id,jdbcType=BIGINT}, #{bookName,jdbcType=VARCHAR}, #{bookWriter,jdbcType=VARCHAR},
#{bookPublisher,jdbcType=VARCHAR}, #{bookIsrent,jdbcType=BIGINT}, #{bookPerson,jdbcType=VARCHAR}
)
</insert>
<insert id="insertSelective" parameterType="com.ray.entity.Book">
insert into book
<trim prefix="(" suffix=")" suffixOverrides=",">
<if test="id != null">
id,
</if>
<if test="bookName != null">
book_name,
</if>
<if test="bookWriter != null">
book_writer,
</if>
<if test="bookPublisher != null">
book_publisher,
</if>
<if test="bookIsrent != null">
book_isrent,
</if>
<if test="bookPerson != null">
book_person,
</if>
</trim>
<trim prefix="values (" suffix=")" suffixOverrides=",">
<if test="id != null">
#{id,jdbcType=BIGINT},
</if>
<if test="bookName != null">
#{bookName,jdbcType=VARCHAR},
</if>
<if test="bookWriter != null">
#{bookWriter,jdbcType=VARCHAR},
</if>
<if test="bookPublisher != null">
#{bookPublisher,jdbcType=VARCHAR},
</if>
<if test="bookIsrent != null">
#{bookIsrent,jdbcType=BIGINT},
</if>
<if test="bookPerson != null">
#{bookPerson,jdbcType=VARCHAR},
</if>
</trim>
</insert>
<update id="updateByPrimaryKeySelective" parameterType="com.ray.entity.Book">
update book
<set>
<if test="bookName != null">
book_name = #{bookName,jdbcType=VARCHAR},
</if>
<if test="bookWriter != null">
book_writer = #{bookWriter,jdbcType=VARCHAR},
</if>
<if test="bookPublisher != null">
book_publisher = #{bookPublisher,jdbcType=VARCHAR},
</if>
<if test="bookIsrent != null">
book_isrent = #{bookIsrent,jdbcType=BIGINT},
</if>
<if test="bookPerson != null">
book_person = #{bookPerson,jdbcType=VARCHAR},
</if>
</set>
where id = #{id,jdbcType=BIGINT}
</update>
<update id="updateByPrimaryKey" parameterType="com.ray.entity.Book">
update book
set book_name = #{bookName,jdbcType=VARCHAR},
book_writer = #{bookWriter,jdbcType=VARCHAR},
book_publisher = #{bookPublisher,jdbcType=VARCHAR},
book_isrent = #{bookIsrent,jdbcType=BIGINT},
book_person = #{bookPerson,jdbcType=VARCHAR}
where id = #{id,jdbcType=BIGINT}
</update>
</mapper>
第四步:修改BookMapper.java
package com.ray.dao;
import com.ray.entity.Book;
import org.apache.ibatis.annotations.Param;
import java.util.List;
public interface BookMapper {
int deleteByPrimaryKey(Long id);
int insert(Book record);
int insertSelective(Book record);
Book selectByPrimaryKey(Long id);
int updateByPrimaryKeySelective(Book record);
int updateByPrimaryKey(Book record);
List<Book> selectBookByName(@Param("bookName") String bookName);
}
第五步:修改BookService和BookServiceImpl
/**
* @author Ray
* @date 2018/5/23 0023
*/
public interface BookService {
List<Book> selectByName(String bookName);
Book selectBookById(Long id);
List<Book> selectBookByBorrowPerson(String PersonName);
void save(Book book);
void borrow(Long id,String username);
void returnBook(Long id);
}
package com.ray.service.impl;
import com.ray.dao.BookMapper;
import com.ray.entity.Book;
import com.ray.service.BookService;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import javax.annotation.Resource;
import java.util.List;
/**
* @author Ray
* @date 2018/5/23 0023
*/
@Service
@Transactional(rollbackFor = Exception.class)
public class BookServiceImpl implements BookService {
@Resource
private BookMapper bookMapper;
@Override
public List<Book> selectByName(String bookName) {
List<Book> books = bookMapper.selectBookByName(bookName);
return books;
}
@Override
public Book selectBookById(Long id) {
return null;
}
@Override
public List<Book> selectBookByBorrowPerson(String PersonName) {
return null;
}
@Override
public void save(Book book) {
}
@Override
public void borrow(Long id, String username) {
}
@Override
public void returnBook(Long id) {
}
}
第六步:创建BookController
package com.ray.controller;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import com.ray.entity.Book;
import com.ray.service.BookService;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import javax.annotation.Resource;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.util.List;
/**
* @author Ray
* @date 2018/5/23 0023
*/
@Controller
public class BookController {
@Resource
private BookService bookService;
@RequestMapping("search")
@ResponseBody
public JSONArray searchBook(HttpServletRequest request, HttpServletResponse response){
String bookName = request.getParameter("bookName");
System.out.println("search: " + bookName);
List<Book> books = bookService.selectByName(bookName);
//创建JSONArray对象
JSONArray jsonArray = new JSONArray();
for (Book book : books){
//创建JSONObject对象
JSONObject jsonObject = new JSONObject();
//添加键值对
jsonObject.put("bookId",book.getId());
jsonObject.put("bookName",book.getBookName());
jsonObject.put("bookWriter",book.getBookWriter());
jsonObject.put("Publisher",book.getBookPublisher());
jsonObject.put("isrent",book.getBookIsrent());
jsonArray.add(jsonObject);
}
HttpSession session = request.getSession();
session.setAttribute("searchResult",jsonArray);
System.out.println("jsonArray: " + jsonArray.toString());
return jsonArray;
}
}
第七步:测试运行
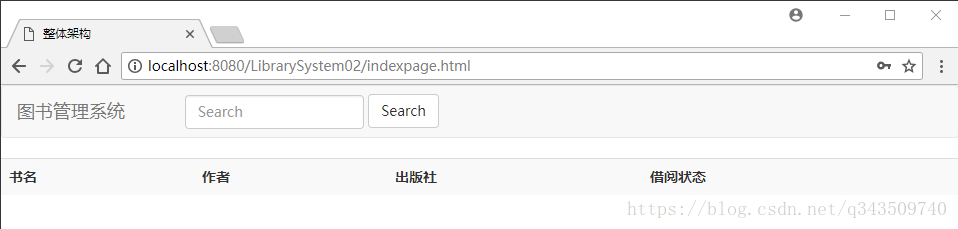
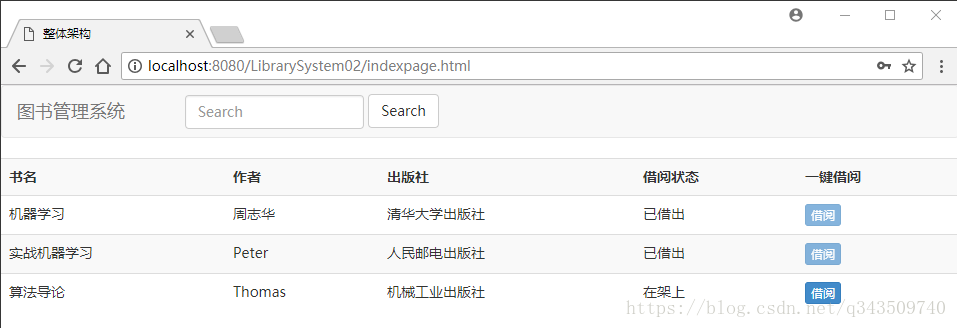
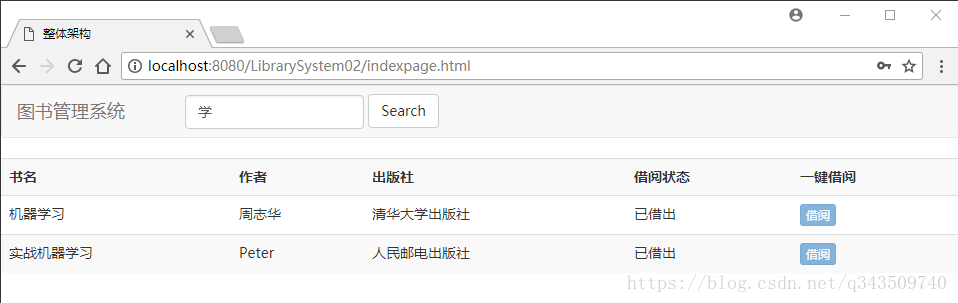