开发技术
- 开发系统: Win10+MySQL8+Spring4+Mybatis3+Tomcat8.5+IDEA
- 开发技术: JSP/Servlet+MySQL+Spring+Mybatis
开发流程
- 首先完成数据库的设计
- 完成控制模块的开发
- 完成部门及员工管理实现
- 完成登录与个人中心实现
- 最后完成日志处理实现
开发要点
Spring与Mabtis的整合
<!--Spring 整合 Mybatis-->
<!--数据源-->
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.cj.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/sm?useUnicode=true&characterEncoding=UTF-8&useJDBCCompliantTimezoneShift=true&useLegacyDatetimeCode=false&serverTimezone=GMT%2B8"/>
<property name="username" value="root"/>
<property name="password" value="1234"/>
</bean>
<!--session工厂-->
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource"/>
<property name="typeAliasesPackage" value="com.jesse.sm.entity"/>
</bean>
<!--持久化对象-->
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="basePackage" value="com.jesse.sm.dao"/>
<property name="sqlSessionFactoryBeanName" value="sqlSessionFactory"/>
</bean>
Spring事务管理
<!--声明式事务-->
<!--事务管理器-->
<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dataSource"/>
</bean>
<!--通知-->
<tx:advice id="txAdvice" transaction-manager="transactionManager">
<tx:attributes>
<tx:method name="get*" read-only="true"/>
<tx:method name="find*" read-only="true"/>
<tx:method name="search*" read-only="true"/>
<tx:method name="*" propagation="REQUIRED"/>
</tx:attributes>
</tx:advice>
<!--植入-->
<aop:config>
<aop:pointcut id="txPointcut" expression="execution(* com.jesse.sm.service.*.*(..))"/>
<aop:advisor advice-ref="txAdvice" pointcut-ref="txPointcut"/>
</aop:config>
Spring的IoC容器使用
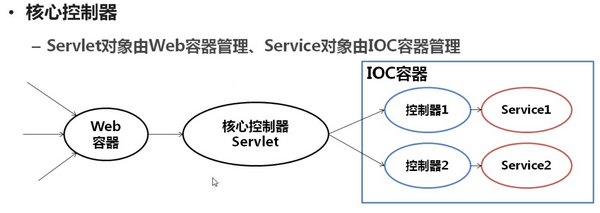
SpringAOP实现日志管理
@Component
@Aspect
public class LogAdvice {
@Autowired
private LogService logService;
@AfterReturning("execution(* com.jesse.sm.controller.*.*(..)) && !execution(* com.jesse.sm.controller.SelfController.*(..)) && !execution(* com.jesse.sm.controller.*.to*(..))")
public void operationLog(JoinPoint joinPoint){
Log log = new Log();
log.setModule(joinPoint.getTarget().getClass().getSimpleName());
log.setOperation(joinPoint.getSignature().getName());
HttpServletRequest request = (HttpServletRequest) joinPoint.getArgs()[0];
HttpSession session = request.getSession();
Object obj = session.getAttribute("USER");
Staff staff = (Staff)obj;
log.setOperator(staff.getAccount());
log.setResult("成功");
logService.addOperationLog(log);
}
@AfterThrowing(throwing = "e",pointcut = "execution(* com.jesse.sm.controller.*.*(..)) && !execution(* com.jesse.sm.controller.SelfController.*(..))")
public void systemLog(JoinPoint joinPoint, Throwable e){
Log log = new Log();
log.setModule(joinPoint.getTarget().getClass().getSimpleName());
log.setOperation(joinPoint.getSignature().getName());
HttpServletRequest request = (HttpServletRequest) joinPoint.getArgs()[0];
HttpSession session = request.getSession();
Object obj = session.getAttribute("USER");
Staff staff = (Staff)obj;
log.setOperator(staff.getAccount());
log.setResult(e.getClass().getSimpleName());
logService.addSystemLog(log);
}
@AfterReturning("execution(* com.jesse.sm.controller.SelfController.login(..))")
public void loginLog(JoinPoint joinPoint){
log(joinPoint);
}
@Before("execution(* com.jesse.sm.controller.SelfController.logout(..))")
public void logoutLog(JoinPoint joinPoint){
log(joinPoint);
}
private void log(JoinPoint joinPoint){
Log log = new Log();
log.setModule(joinPoint.getTarget().getClass().getSimpleName());
log.setOperation(joinPoint.getSignature().getName());
HttpServletRequest request = (HttpServletRequest) joinPoint.getArgs()[0];
HttpSession session = request.getSession();
Object obj = session.getAttribute("USER");
if (obj == null){
log.setOperator(request.getParameter("account"));
log.setResult("失败");
} else {
Staff staff = (Staff) obj;
log.setOperator(staff.getAccount());
log.setResult("成功");
}
logService.addLoginLog(log);
}
}
注解与配置的选用
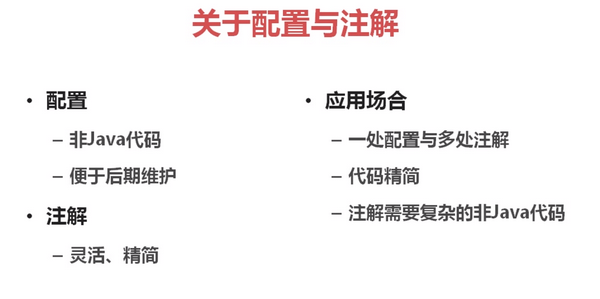
项目展示
个人中心
个人信息
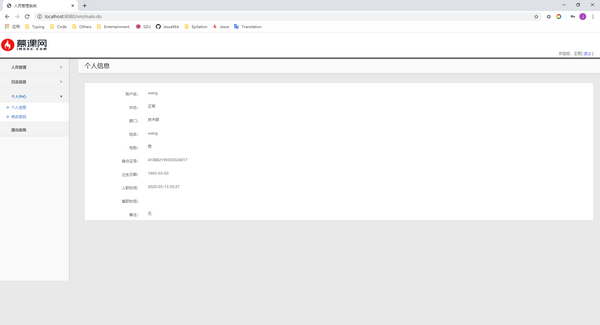
修改密码
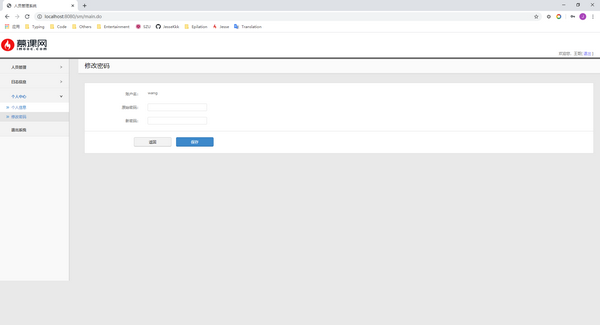
人员管理
员工列表
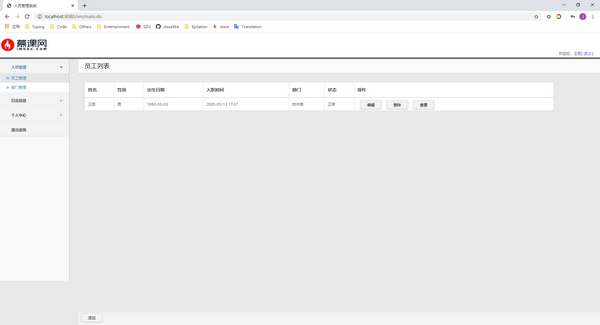
部门列表
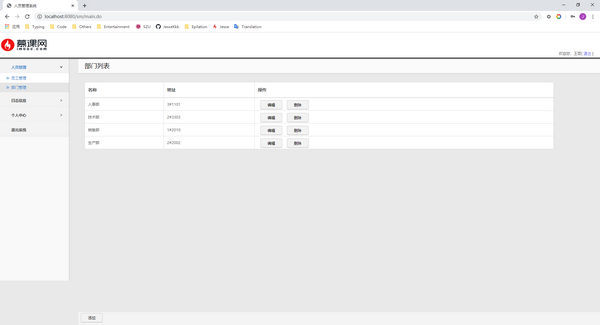
日志管理
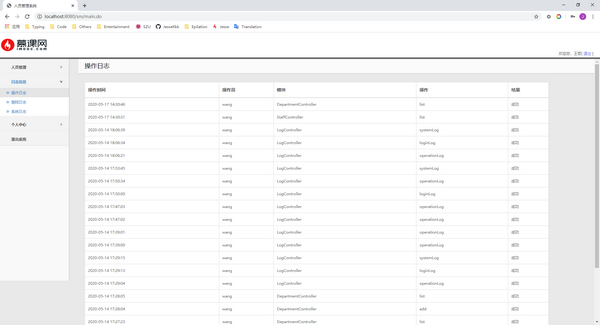