之前面试遇到的一道机试题,当时时间不够没有调出来,有时间把它整了一下
代码
public class MainActivity extends ActionBarActivity implements OnClickListener {
private ArrayList<TextView> allViews;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initView();
}
private void initView() {
allViews = new ArrayList<TextView>();
allViews.add((TextView) findViewById(R.id.a));
allViews.add((TextView) findViewById(R.id.b));
allViews.add((TextView) findViewById(R.id.c));
allViews.add((TextView) findViewById(R.id.d));
allViews.add((TextView) findViewById(R.id.e));
findViewById(R.id.f).setOnClickListener(this);
findViewById(R.id.g).setOnClickListener(this);
}
// 开始排序(运行在子线程,需要暂停)
private void starSort(final ArrayList<TextView> allViews) {
new Thread(new Runnable() {
@Override
public void run() {
// 冒泡排序
maoPao(allViews);
// 这里子线程 必须先Looper.prepare(); 才能toast
Looper.prepare();
Toast.makeText(MainActivity.this, "排序完成", 0).show();
Looper.loop();
}
}).start();
}
// 冒泡排序
private void maoPao(final ArrayList<TextView> allViews) {
for (int i = 0; i < allViews.size() - 1; i++) {
for (int j = 0; j < allViews.size() - 1 - i; j++) {
if (getTextNum(allViews.get(j)) < getTextNum(allViews
.get(j + 1))) {
// 开始动画
startMove(MainActivity.this, allViews.get(j),
allViews.get(j + 1));
// 交换位置
TextView tempView = allViews.get(j + 1);
// 先移除 一个
allViews.remove(j + 1);
// 在对应位置添加移除的达到交换位置(这里要注意越界)
allViews.add(
j == allViews.size() ? allViews.size() - 1 : j,
tempView);
try {
// 休眠3秒
Thread.sleep(3000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
}
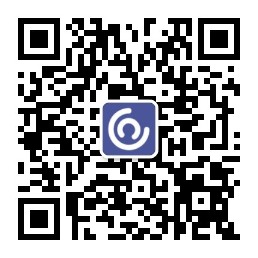
// 开始动画
private void startMove(Activity a, final TextView textView,
final TextView textView2) {
final float t1Y = textView.getY();// 获得view的纵坐标
final float t2Y = textView2.getY();
final float dY = Math.abs(t1Y - t2Y);
// 动画要运行在主线程(操作了textview)
a.runOnUiThread(new Runnable() {
@Override
public void run() {
// 用值动画 完成移动 ,作用对象、属性名称、(Y代表Y轴移动 后面2个代表 起点跟终点)
ObjectAnimator.ofFloat(textView, "Y", t1Y, t1Y + dY)
// 设置执行时间(1000ms)
.setDuration(1000)
// 开始动画
.start();
ObjectAnimator.ofFloat(textView2, "Y", t2Y, t2Y - dY)
.setDuration(1000).start();
}
});
}
// 获取textView的数字
private int getTextNum(TextView tx) {
try {
return Integer.parseInt(tx.getText().toString());
} catch (NumberFormatException e) {
e.printStackTrace();
}
return 0;
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.f:// 点击开始
starSort(allViews);
break;
case R.id.g:// 点击重新
setContentView(R.layout.activity_main);
initView();
break;
}
}
}
---布局-----------------------------
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.example.testanimation.MainActivity" >
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center_horizontal"
android:orientation="vertical" >
<TextView
android:id="@+id/a"
android:layout_width="20dp"
android:layout_height="wrap_content"
android:layout_marginTop="30dp"
android:background="#fd2288"
android:gravity="center"
android:text="1" />
<TextView
android:id="@+id/b"
android:layout_width="20dp"
android:layout_height="wrap_content"
android:layout_marginTop="30dp"
android:background="#fd2288"
android:gravity="center"
android:text="2" />
<TextView
android:id="@+id/c"
android:layout_width="20dp"
android:layout_height="wrap_content"
android:layout_marginTop="30dp"
android:background="#fd2288"
android:gravity="center"
android:text="3" />
<TextView
android:id="@+id/d"
android:layout_width="20dp"
android:layout_height="wrap_content"
android:layout_marginTop="30dp"
android:background="#fd2288"
android:gravity="center"
android:text="4" />
<TextView
android:id="@+id/e"
android:layout_width="20dp"
android:layout_height="wrap_content"
android:layout_marginTop="30dp"
android:background="#fd2288"
android:gravity="center"
android:text="5" />
<TextView
android:id="@+id/f"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:background="#fd2288"
android:text="开始" />
<TextView
android:id="@+id/g"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:background="#fd2288"
android:text="重新" />
</LinearLayout>
</RelativeLayout>