tree前端实现
前言
昨天学习了树(tree)形结构
今天来把数据库的表实现到页面上
完成目标
今天写的时候还遇到个错误
在请求目标中发现无效字符。有效字符在RFC 7230和RFC 3986中定义
然后百度了下发现这个问题是高版本tomcat中的新特性:就是严格按照 RFC 3986规范进行访问解析,而 RFC 3986规范定义了Url中只允许包含英文字母(a-zA-Z)、数字(0-9)、-_.~4个特殊字符以及所有保留字符(RFC3986中指定了以下字符为保留字符:! * ’ ( ) ; : @ & = + $ , / ? # [ ])。而我们的系统在通过地址传参时,在url中传了一段json,传入的参数中有”{“不在RFC3986中的保留字段中,所以会报这个错。
这里有三种解决方法 我用的第一种
- 编辑Tomcat /config/catalina.properties 配置文件,找到 tomcat.util.http.parser.HttpParser.requestTargetAllow=|,解开注释,并配置tomcat.util.http.parser.HttpParser.requestTargetAllow=|{}。(此属性只支持| { } 三种字符)。
解决办法2:
最轻便的方法,更换tomcat版本。此方法比较快。
解决办法3:
对相应的参数进行编码,就是将所有的参数都进行编码
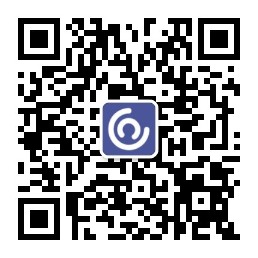
------------参考文章------------
实现
要实现页面我们要写dao方法 写dao方法要继承basedao 我们需要mvc的架包
首先导入mvc的架包 再把配置文件conf 和下面的mvc.xml也C过来
然后写dao方法
package com.zhuchenxi.dao;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.zhuchenxi.entity.Permission;
import com.zhuchenxi.util.BaseDao;
import com.zhuchenxi.util.BuildTree;
import com.zhuchenxi.util.PageBean;
import com.zhuchenxi.vo.TreeVo;
public class PermissionDao extends BaseDao<Permission> {
/**
*
* 是直接从数据库获取到的数据
* @param permission
* @param pageBean
* @return
* @throws Exception
*/
public List<Permission> list(Permission permission,PageBean pageBean) throws Exception {
String sql="select * from t_easyui_Permission";
return super.executeQuery(sql, Permission.class, pageBean);
}
/**
* 能够将数据库中的数据,体现夫子结构
* @param permission
* @param pageBean
* @return
* @throws Exception
*/
// public TreeVo<Permission> topNode(Permission permission,PageBean pageBean) throws Exception {
//
// List<Permission> list = this.list(permission, pageBean);
//
// List<TreeVo<Permission>> nodes = new ArrayList<TreeVo<Permission>>();
//
// TreeVo treeVo = null;
//
// for (Permission p : list) {
// treeVo = new TreeVo<>();
// treeVo.setId(p.getId()+"");
// treeVo.setText(p.getName());
// treeVo.setParentId(p.getPid()+"");
// Map<String, Object> attributes = new HashMap<String, Object>();
// attributes.put("self", p);
// treeVo.setAttributes(attributes);
// nodes.add(treeVo);
// }
//
// return BuildTree.build(nodes);
//
// }
public List<TreeVo<Permission>> topNode(Permission permission,PageBean pageBean) throws Exception {
List<Permission> list = this.list(permission, pageBean);
List<TreeVo<Permission>> nodes = new ArrayList<TreeVo<Permission>>();
TreeVo treeVo = null;
for (Permission p : list) {
treeVo = new TreeVo<>();
treeVo.setId(p.getId()+"");
treeVo.setText(p.getName());
treeVo.setParentId(p.getPid()+"");
Map<String, Object> attributes = new HashMap<String, Object>();
attributes.put("self", p);
treeVo.setAttributes(attributes);
nodes.add(treeVo);
}
return BuildTree.buildList(nodes,"0");
}
public static void main(String[] args) throws Exception {
PermissionDao pd = new PermissionDao();
List<Permission> list = pd.list(null, null);
// 通过工具类完成指定格式的输出
List<TreeVo<Permission>> nodes = new ArrayList<TreeVo<Permission>>();
// Permission的格式不满足easyui的tree组件的展现的数据格式的
// 将List<Permission>转换成<TreeVo<T>>
// 实现,将List<Permission>得到的单个permission转成TreeVo,将Treev加入到nodes
TreeVo treeVo = null;
for (Permission p : list) {
treeVo = new TreeVo<>();
treeVo.setId(p.getId()+"");
treeVo.setText(p.getName());
treeVo.setParentId(p.getPid()+"");
// Map<String, Object> attributes = new HashMap<String, Object>();
// attributes.put("self", p);
// treeVo.setAttributes(attributes);
nodes.add(treeVo);
}
TreeVo<Permission> parent = BuildTree.build(nodes);
ObjectMapper om = new ObjectMapper();
String jsonstr = om.writeValueAsString(parent);
System.out.println(jsonstr);
}
}
这里面的topNode是来自于辅助类BuildTree String idParam 就是顶级节点需要什么传什么
然后配置在中央控制器里面
package com.zhuchenxi.web;
import java.util.ArrayList;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.zhuchenxi.dao.PermissionDao;
import com.zhuchenxi.entity.Permission;
import com.zhuchenxi.framework.ActionSupport;
import com.zhuchenxi.framework.ModelDriven;
import com.zhuchenxi.util.PageBean;
import com.zhuchenxi.vo.TreeVo;
import com.zhuchenxi.util.ResponseUtil;
public class PermissionAction extends ActionSupport implements ModelDriven<Permission>{
private Permission ps = new Permission();
private PermissionDao pd = new PermissionDao();
@Override
public Permission getModel() {
// TODO Auto-generated method stub
return ps;
}
public String menuTree(HttpServletRequest req,HttpServletResponse resp) {
try {
// TreeVo<Permission> topNode = this.pd.topNode(null, null);
// List<TreeVo<Permission>> list = new ArrayList<TreeVo<Permission>>();
// list.add(topNode);
ResponseUtil.writeJson(resp, this.pd.topNode(null, null));
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
}
在配置路徑
<action path="/permission" type="com.zhuchenxi.web.PermissionAction">
<!-- <forward name="index" path="/index.jsp" redirect="false" /> -->
</action>
在主界面上写上input 配置在index.js里面
完成效果
实现 t_easyui_menu 表
照搬就行啦
昨天写过的 就不写实体类了 直接改dao方法
package com.zhuchenxi.dao;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.zhuchenxi.entity.Menu;
import com.zhuchenxi.entity.Permission;
import com.zhuchenxi.util.BaseDao;
import com.zhuchenxi.util.BuildTree;
import com.zhuchenxi.util.PageBean;
import com.zhuchenxi.vo.TreeVo;
public class MenuDao extends BaseDao<Menu>{
public List<Menu> list(Menu menu,PageBean pageBean) throws Exception {
String sql="select * from t_easyui_menu where true";
return super.executeQuery(sql, Menu.class, pageBean);
}
public List<TreeVo<Menu>> topNode(Menu menu,PageBean pageBean) throws Exception {
List<Menu> list = this.list(menu, pageBean);
List<TreeVo<Menu>> nodes = new ArrayList<TreeVo<Menu>>();
TreeVo treeVo = null;
for (Menu p : list) {
treeVo = new TreeVo<>();
treeVo.setId(p.getMenuid());
treeVo.setText(p.getMenuname());
treeVo.setParentId(p.getParentid());
Map<String, Object> attributes = new HashMap<String, Object>();
attributes.put("self", p);
treeVo.setAttributes(attributes);
nodes.add(treeVo);
}
return BuildTree.buildList(nodes,"-1");
}
public static void main(String[] args) throws Exception {
MenuDao md = new MenuDao();
List<Menu> list = md.list(null, null);
// 通锟斤拷锟斤拷锟斤拷锟斤拷锟斤拷锟街革拷锟斤拷锟绞斤拷锟斤拷锟斤拷
List<TreeVo<Menu>> nodes = new ArrayList<TreeVo<Menu>>();
TreeVo treeVo = null;
for (Menu p : list) {
treeVo = new TreeVo<>();
treeVo.setId(p.getMenuid());
treeVo.setText(p.getMenuname());
treeVo.setParentId(p.getParentid());
// Map<String, Object> attributes = new HashMap<String, Object>();
// attributes.put("self", p);
// treeVo.setAttributes(attributes);
nodes.add(treeVo);
}
TreeVo<Menu> parent = BuildTree.build(nodes);
ObjectMapper om = new ObjectMapper();
String jsonstr = om.writeValueAsString(parent);
System.out.println(jsonstr);
}
}
配置中央控制器
package com.zhuchenxi.web;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.zhuchenxi.dao.MenuDao;
import com.zhuchenxi.entity.Menu;
import com.zhuchenxi.framework.ActionSupport;
import com.zhuchenxi.framework.ModelDriven;
import com.zhuchenxi.util.ResponseUtil;
public class MenuAction extends ActionSupport implements ModelDriven<Menu> {
private Menu menu = new Menu();
private MenuDao md = new MenuDao();
@Override
public Menu getModel() {
// TODO Auto-generated method stub
return menu;
}
public String menuTree(HttpServletRequest req,HttpServletResponse resp) {
try {
// TreeVo<Permission> topNode = this.pd.topNode(null, null);
// List<TreeVo<Permission>> list = new ArrayList<TreeVo<Permission>>();
// list.add(topNode);
ResponseUtil.writeJson(resp, this.md.topNode(null, null));
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
}
重点来了 因为在dao方法里调的buidList方法 在index.js里面改下路径 就可以显示页面了 超级方便
效果图
现在思路越来越好了 很高兴 希望能保持下去~~