Spring Cloud 模块使用Mybatis
模块引用Mybatis依赖
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
<version>2.0.2.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-config</artifactId>
<version>2.0.2.RELEASE</version>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.0</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.47</version>
</dependency>
</dependencies>
对应数据库中的表建立实体类
CREATE TABLE `NewTable` (
`id` int(10) NOT NULL AUTO_INCREMENT ,
`name` varchar(100) CHARACTER SET utf8 COLLATE utf8_general_ci NULL DEFAULT NULL ,
`price` double(100,0) NULL DEFAULT NULL ,
`flavor` varchar(255) CHARACTER SET utf8 COLLATE utf8_general_ci NULL DEFAULT NULL ,
`type_id` int(11) NULL DEFAULT NULL ,
PRIMARY KEY (`id`)
)
ENGINE=InnoDB
DEFAULT CHARACTER SET=utf8 COLLATE=utf8_general_ci
AUTO_INCREMENT=1
ROW_FORMAT=COMPACT;
package com.li.menu.entity;
@Data
public class Menu {
private String name;
private Double price;
private String flavor;
private int type_id;
}
建立MenuMapper和对应的xml映射文件
package com.li.menu.repository;
import com.li.menu.entity.Menu;
import java.util.List;
public interface MenuRepository {
public List<Menu> findAll(int index,int limit);
public int count();
public Menu findById(Integer id);
public void save(Menu menu);
public void update(Menu menu);
public void deleteById(Integer id);
}
- MenuRepository.xml 文件位置放在resource的mapper下,该地址在配置文件中配置,让容器读到映射文件
<?xml version="1.0" encoding="utf-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.li.menu.repository.MenuRepository">
<select id="findAll" resultType="com.li.menu.entity.Menu">
select * from t_menu limit #{index},#{limit}
</select>
<select id="count" resultType="int">
select count(id) from t_menu
</select>
<select id="findById" parameterType="int" resultType="com.li.menu.entity.Menu">
select * from t_menu where id=#{id}
</select>
<insert id="save" parameterType="com.li.menu.entity.Menu">
insert into t_menu (name,price,flavor) values (#{name},#{price},#{flavor})
</insert>
<update id="update" parameterType="com.li.menu.entity.Menu">
update t_menu set name=#{name},price=#{price},flavor=#{flavor} where id=#{id}
</update>
<delete id="deleteById" parameterType="int">
delete from t_menu where id=#{id}
</delete>
</mapper>
配置文件中配置Mybatis
server:
port: 8011
spring:
application:
name: menu
datasource:
name: mysqlserver
url: jdbc:mysql://localhost:3306/shop?useUnicode=true
username: root
password: root
eureka:
client:
service-url:
defaultZone: http://localhost:8001/eureka/
instance:
prefer-ip-address: true
mybatis:
mapper-locations: classpath:/mapping/*.xml
使用Mapper中的数据操作接口
package com.li.menu.controller;
import com.li.menu.entity.Menu;
import com.li.menu.repository.MenuRepository;
import com.netflix.discovery.converters.Auto;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/menu")
public class MenuController {
@Autowired
MenuRepository menuRepository;
@GetMapping("/findAll/{index}/{limit}")
public List<Menu> findall(@PathVariable("index") int index,@PathVariable("limit") int limit){
return menuRepository.findAll(index,limit);
}
}
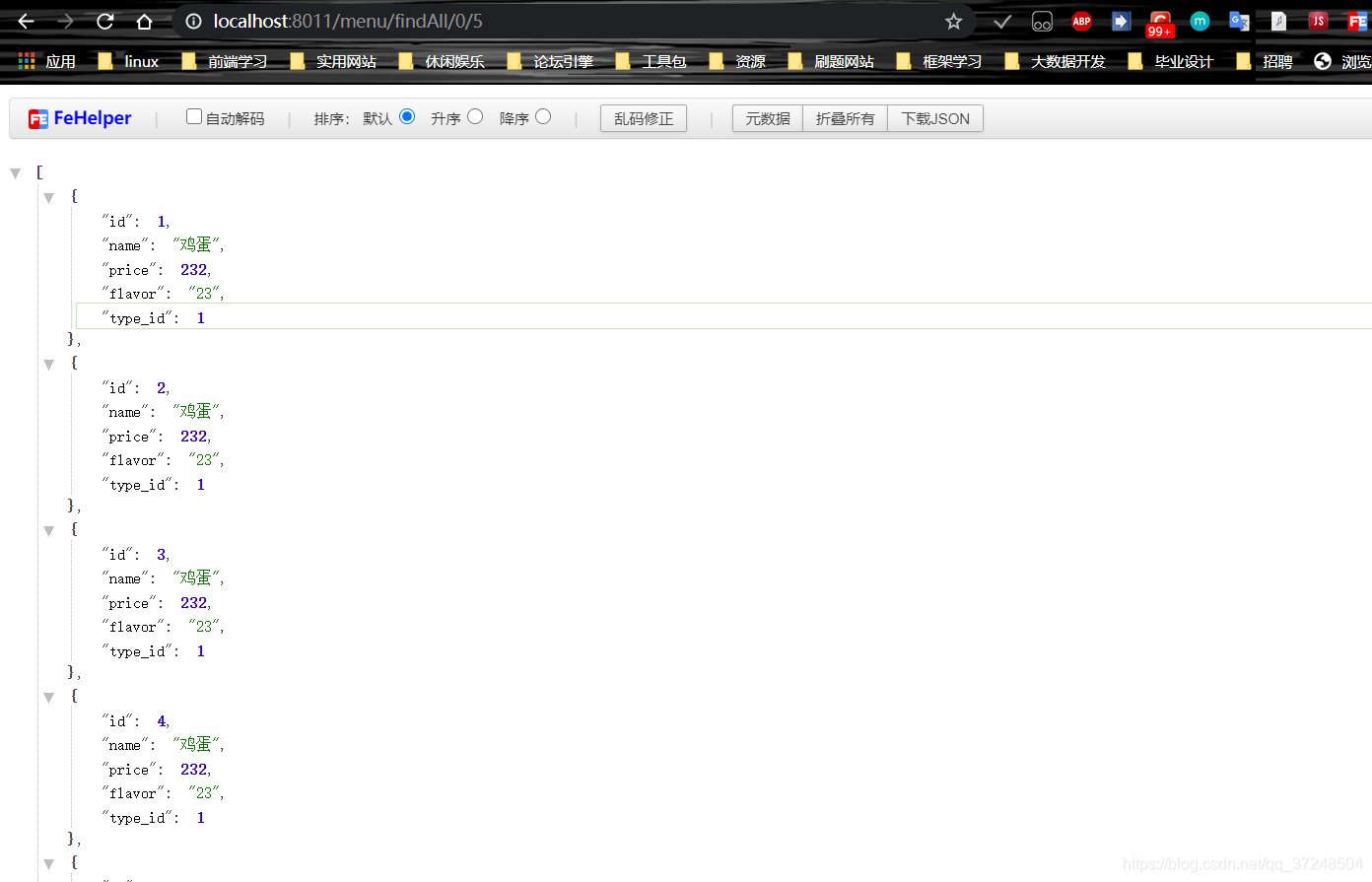
- 注意上面的代码中手动注入的MenuRepository不能被Ioc容器找到,所以应该在容器启动的时候通过扫描的方式注入Menu的Mapper,启动类加上扫描注解如下:
@SpringBootApplication
@MapperScan("com.li.menu.repository")
public class MenuApplication {
public static void main(String[] args) {
SpringApplication.run(MenuApplication.class, args);
}
}