作业要求:
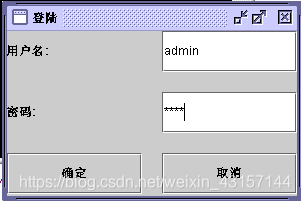
- 根据以上登陆界面内容设计一个数据表, 要求用户正确的输入用户名和密码允许用户登陆, 否则输出密码或用户名不正确.
- 根据下面要求编写程序
- 设计一个图形界面
- 在数据库中建立一个表
- 表名为学生
- 表结构为:学号, 姓名, 性别, 年龄, JAVA语言, 数据结构, 数据库, 总分
- 功能要求: 实现对对数据库的增, 删, 改, 查操作
界面展示与功能说明
登录界面:
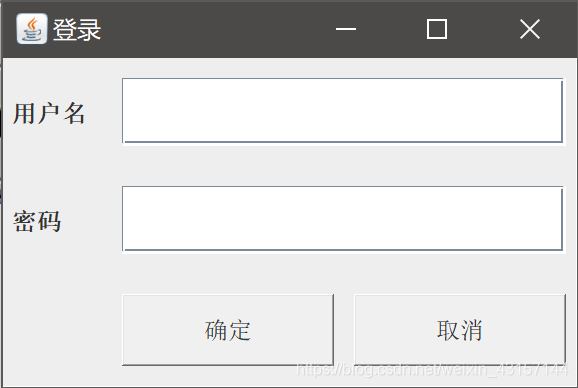
- 数据库中有三个字段, 分别为递增整数主键id, 表中唯一且可索引的可变长字符串(最长32个字符)字段username与64字节定长字节字段password(用来储存使用SHA-512进行散列的密码).
- 数据库中初始化了用户名为
admin
, 散列前密码也为admin
的管理员账户.
- 当输入的用户名不存在或用户名对应的密码不一致时, 点击确定按钮将弹出一个提示框.
- 点击取消按钮将结束程序.
- 当输入的用户名存在且数据库中的密码散列与输入框中的密码散列后的字节串相同, 点击确定按钮将弹出一个提示框.
- 单击确定后将隐藏登录界面并进入信息管理页面.
信息管理界面:
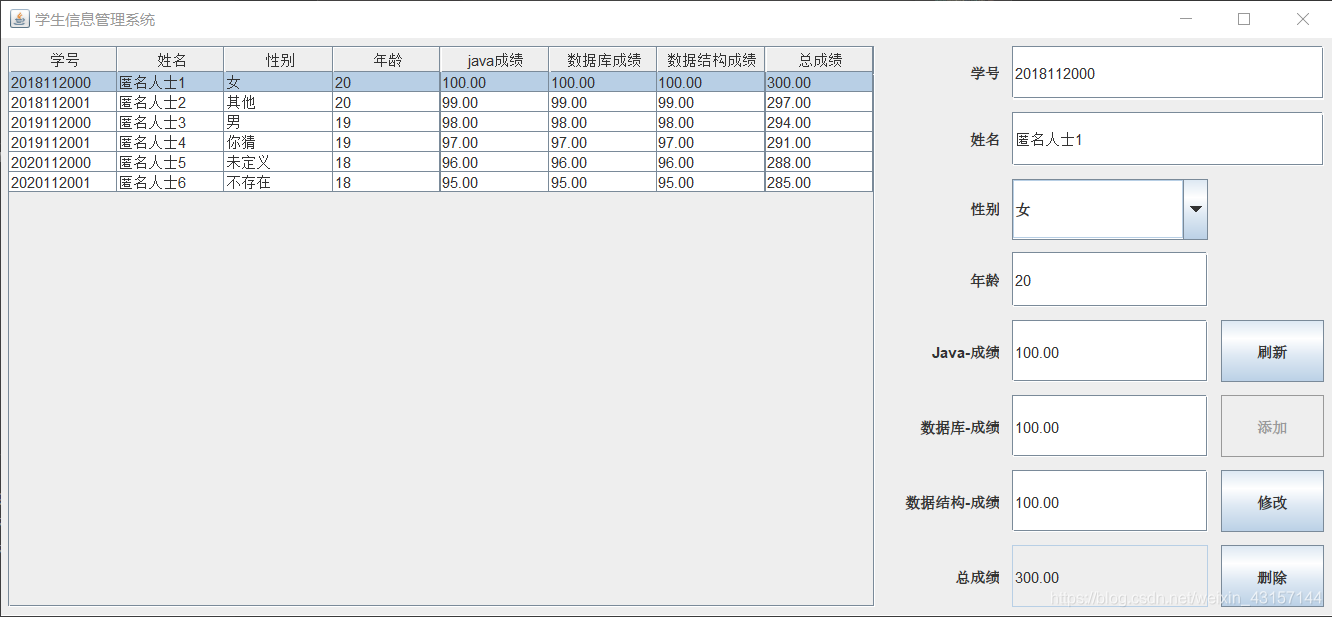
初始状态:
- 程序UI由左侧的显示面板与右侧的操作面板组成, 单击窗口关闭按钮将退出程序.
- 左侧显示面板实时显示数据库对应表中所有的记录与字段, 并具有滚动条进行翻页.
- 操作面板提供数据的增删改查功能, 并依靠业务逻辑设置按钮的活动与禁用, 以避免数据库的错误操作.
添加记录:
- 在右侧数据编辑面板编辑需要添加字段的信息.
- 学号必须为10位字符, 若不为10位字符, 则禁用添加, 修改与删除按钮.
- 若学号为10位字符且在数据库中具有能够匹配相应记录(学号为唯一字段), 则更新其他输入框中的内容并激活修改与删除按钮并禁用添加按钮, 可以使用该功能通过学号查询对应学生的信息.
- 若学号为10位字符且在数据库中没有能与其匹配的相应记录(学号为唯一字段), 则更新其他输入框中的内容并激活添加按钮并禁用修改与删除按钮.
- 若在添加时成绩具有小数点后第三位或更小的位数, 则会对小数点后第三位进行四舍五入操作.
- 性别下拉选项框可以在其选项中选择, 也可以通过单击选项框直接编辑.
- 若三门课的成绩不在0~100范围内, 则在点击添加按钮 / 点击修改按钮 / 输入完成后单击回车键时弹出一个提示框.
- 该提示框单击确定按钮后程序将不进行任何数据库操作, 直到所有数据都合理规范.
- 若在输入完成后单击回车键时弹出该对话框, 则会清除该输入框中的不合理数据.
- 若三门课成绩的输入框与年龄的输入框中存在非法字符, 则在点击添加按钮 / 点击修改按钮 / 输入完成后单击回车键时弹出一个提示框.
- 若在输入完成后单击回车键时弹出该对话框, 则会清除该输入框中的不合理数据.
修改记录:
- 在三门课程成绩的输入框中输入数据后单击回车键则会对输入框中的字符串进行处理, 若字符串可以解析为一个数字且其数值在0~100范围内, 则会保留两位小数并对小数点后第三位(若有)进行四舍五入操作.
- 总成绩不可编辑, 由系统对三门课程的成绩自动进行求和.
- 左侧信息显示区不能直接编辑, 但是单击对应的记录后该记录的信息将更新到右侧信息编辑区, 在编辑区便可以对数据进行修改, 修改完成后点击修改按钮则将修改后的字段同步更新至数据库, 左侧显示区也会自动刷新.
- 左侧信息显示区中的记录将会按照学号递增的顺序排序.
- 四个操作按钮(刷新/添加/修改/删除)响应后左侧显示区均会进行刷新.
删除记录:
- 只有在数据库中存在对应学号的记录时删除按钮才会激活.
- 删除后编辑区信息不会改变, 若误操作可以单击添加按钮将删除的信息重新添加进数据库.
程序源代码
package assignment.week_12;
import javax.swing.*;
import javax.swing.table.*;
import javax.swing.event.*;
import java.awt.event.*;
import java.awt.*;
import java.sql.*;
import java.util.*;
import java.security.*;
import java.math.BigDecimal;
import java.math.RoundingMode;
public class StudentManage implements ActionListener
{
private JLabel label_username;
private JLabel label_password;
private JTextField field_username;
private JPasswordField field_password;
private Button button_login;
private Button button_exit;
private JFrame frame_login;
private Connection connection;
private StudentInfoManage student_info_manage;
private static final String username = "admin";
private static final String password = "admin";
private static final String JDriver = "org.sqlite.JDBC";
private static final String conURL = "jdbc:sqlite:assignment\\assignment.db";
public static void main(String arg[])
{
new StudentManage().run();
}
public static void init()
{
try {
Class.forName(JDriver);
} catch (java.lang.ClassNotFoundException e) {
System.out.println("ForName: " + e.getMessage());
}
try {
Connection connection = DriverManager.getConnection(conURL);
Statement statement = connection.createStatement();
String query = "CREATE TABLE USER("
+ "id INTEGER NOT NULL UNIQUE PRIMARY KEY AUTOINCREMENT,"
+ "username VARCHAR(32) NOT NULL UNIQUE,"
+ "password BINARY(64) NOT NULL"
+ ");";
query += "CREATE UNIQUE INDEX index_user ON USER (id, username);";
statement.executeUpdate(query);
statement.close();
PreparedStatement p_statement = connection.prepareStatement("INSERT INTO USER(username, password) values(?, ?);");
p_statement.setString(1, StudentManage.username);
p_statement.setBytes(2, getHashBiteString(StudentManage.password));
p_statement.executeUpdate();
p_statement.close();
connection.close();
} catch (SQLException e) {
JOptionPane.showMessageDialog(null, String.format("异常信息:\n%s\n%s", e.toString(), e.getStackTrace()), "运行时异常", JOptionPane.ERROR_MESSAGE);
}
}
public StudentManage()
{
try {
Class.forName(JDriver);
this.connection = DriverManager.getConnection(conURL);
} catch (java.lang.ClassNotFoundException e) {
JOptionPane.showMessageDialog(null, String.format("异常信息:\n%s\n%s", e.toString(), e.getStackTrace()), "运行时异常", JOptionPane.ERROR_MESSAGE);
System.exit(0);
} catch (SQLException e) {
JOptionPane.showMessageDialog(null, String.format("异常信息:\n%s\n%s", e.toString(), e.getStackTrace()), "运行时异常", JOptionPane.ERROR_MESSAGE);
System.exit(0);
}
}
protected void finalize()
{
try {
this.connection.close();
} catch (SQLException e) {
JOptionPane.showMessageDialog(null, String.format("异常信息:\n%s\n%s", e.toString(), e.getStackTrace()), "运行时异常", JOptionPane.ERROR_MESSAGE);
System.exit(0);
}
}
public void run()
{
this.frame_login = new JFrame("登录");
frame_login.setSize(300, 200);
frame_login.setLocation(500, 300);
frame_login.setBackground(Color.lightGray);
frame_login.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame_login.setLayout(new GridBagLayout());
GridBagConstraints gbc = new GridBagConstraints();
gbc.anchor = GridBagConstraints.CENTER;
gbc.fill = GridBagConstraints.BOTH;
gbc.insets = new Insets(10, 5, 10, 5);
this.label_username = new JLabel("用户名", JLabel.LEFT);
this.label_password = new JLabel("密码", JLabel.LEFT);
this.field_username = new JTextField(20);
this.field_password = new JPasswordField(20);
this.button_login = new Button("确定");
this.button_exit = new Button("取消");
Container frame_contain = frame_login.getContentPane();
addComponent(label_username, frame_contain, gbc, 0, 0, 1, 1, 1, 1);
addComponent(field_username, frame_contain, gbc, 0, 1, 3, 1, 3, 1);
addComponent(label_password, frame_contain, gbc, 1, 0, 1, 1, 1, 1);
addComponent(field_password, frame_contain, gbc, 1, 1, 3, 1, 3, 1);
addComponent(button_login, frame_contain, gbc, 2, 1, 1, 1, 1, 1);
addComponent(button_exit, frame_contain, gbc, 2, 3, 1, 1, 1, 1);
this.button_login.addActionListener(this);
this.button_exit.addActionListener(this);
frame_login.setVisible(true);
}
private void addComponent(Component c, Container contain, GridBagConstraints gbc, int row, int column, int width, int height, int weightx, int weighty)
{
gbc.gridy = row;
gbc.gridx = column;
gbc.gridwidth = width;
gbc.gridheight = height;
gbc.weightx = weightx;
gbc.weighty = weighty;
gbc.ipadx = 2;
gbc.ipady = 2;
contain.add(c, gbc);
}
private static byte[] getHashBiteString(String str)
{
try {
MessageDigest digest = MessageDigest.getInstance("SHA-512");
return digest.digest(str.getBytes());
} catch (NoSuchAlgorithmException e) {
JOptionPane.showMessageDialog(null, String.format("异常信息:\n%s\n%s", e.toString(), e.getStackTrace()), "运行时异常", JOptionPane.ERROR_MESSAGE);
System.exit(0);
}
return null;
}
public void actionPerformed(ActionEvent event)
{
if (event.getSource() == this.button_login) {
try {
boolean auth_flag = false;
byte byte_string[] = getHashBiteString(String.valueOf(this.field_password.getPassword()));
PreparedStatement p_statement = this.connection.prepareStatement("SELECT * FROM USER WHERE username=?;");
p_statement.setString(1, this.field_username.getText());
ResultSet result_set = p_statement.executeQuery();
if (result_set.next()) {
if (Arrays.equals(byte_string, result_set.getBytes("password"))) auth_flag = true;
}
if (auth_flag) {
JOptionPane.showMessageDialog(null, "恭喜您, 登录成功!", "登录信息", JOptionPane.INFORMATION_MESSAGE);
this.student_info_manage = new StudentInfoManage();
this.student_info_manage.display();
this.frame_login.setVisible(false);
} else {
JOptionPane.showMessageDialog(null, "登录失败, 用户名或密码错误", "登录信息", JOptionPane.WARNING_MESSAGE);
}
p_statement.close();
} catch (SQLException e) {
JOptionPane.showMessageDialog(null, String.format("异常信息:\n%s\n%s", e.toString(), e.getStackTrace()), "运行时异常", JOptionPane.ERROR_MESSAGE);
System.exit(0);
} catch (Exception e) {
JOptionPane.showMessageDialog(null, String.format("异常信息:\n%s\n%s", e.toString(), e.getStackTrace()), "运行时异常", JOptionPane.ERROR_MESSAGE);
System.exit(0);
}
} else if (event.getSource() == this.button_exit) {
System.exit(0);
}
}
}
class StudentInfoManage implements ActionListener, ItemListener, CaretListener, ListSelectionListener
{
private JFrame frame;
private JLabel lable_student_id;
private JLabel lable_name;
private JLabel lable_gender;
private JLabel lable_age;
private JLabel lable_java;
private JLabel lable_database;
private JLabel lable_data_structure;
private JLabel lable_total;
private JTextField field_student_id;
private JTextField field_name;
private JTextField field_age;
private JTextField field_java;
private JTextField field_database;
private JTextField field_data_structure;
private JTextField field_total;
private JButton button_add;
private JButton button_alter;
private JButton button_delete;
private JButton button_refresh;
private JComboBox<String> combo_box_gender;
private JScrollPane scroll_pane_table;
private JTable table;
private static final String gender[] = {
"女", "男", "其他" };
private StudentInfo student_info;
private Connection connection;
private static final String JDriver = "org.sqlite.JDBC";
private static final String conURL = "jdbc:sqlite:assignment\\assignment.db";
private class StudentInfo
{
String student_id;
String name;
String gender;
int age;
BigDecimal java;
BigDecimal database;
BigDecimal data_structure;
BigDecimal total;
ResultSet result_set;
final String column_titles[] = {
"学号", "姓名", "性别", "年龄", "java成绩", "数据库成绩", "数据结构成绩", "总成绩" };
final BigDecimal __100__ = new BigDecimal(100);
final BigDecimal __0__ = new BigDecimal(0);
public StudentInfo()
{
this.java = new BigDecimal(0);
this.database = new BigDecimal(0);
this.data_structure = new BigDecimal(0);
this.total = new BigDecimal(0);
}
public void selectAll() throws SQLException
{
PreparedStatement p_statement = connection.prepareStatement("SELECT * FROM STUDENT ORDER BY student_id;");
this.result_set = p_statement.executeQuery();
}
public boolean next() throws SQLException
{
if (this.result_set.next()) {
this.student_id = result_set.getString("student_id");
this.name = result_set.getString("name");
this.gender = result_set.getString("gender");
this.age = result_set.getInt("age");
this.java = result_set.getBigDecimal("java").setScale(2, RoundingMode.HALF_UP);
this.database = result_set.getBigDecimal("database").setScale(2, RoundingMode.HALF_UP);
this.data_structure = result_set.getBigDecimal("data_structure").setScale(2, RoundingMode.HALF_UP);
this.total = result_set.getBigDecimal("total").setScale(2, RoundingMode.HALF_UP);
return true;
} else return false;
}
public boolean select(String student_id) throws SQLException
{
PreparedStatement p_statement = connection.prepareStatement("SELECT * FROM STUDENT WHERE student_id=?;");
p_statement.setString(1, student_id);
this.result_set = p_statement.executeQuery();
if (this.next()) return true;
else return false;
}
public void insert() throws SQLException
{
PreparedStatement p_statement = connection.prepareStatement("INSERT INTO STUDENT(student_id, name, gender, age, java, database, data_structure, total) VALUES(?, ?, ?, ?, ?, ?, ?, ?);");
p_statement.setString(1, this.student_id);
p_statement.setString(2, this.name);
p_statement.setString(3, this.gender);
p_statement.setInt(4, this.age);
p_statement.setBigDecimal(5, this.java);
p_statement.setBigDecimal(6, this.database);
p_statement.setBigDecimal(7, this.data_structure);
p_statement.setBigDecimal(8, this.total);
p_statement.executeUpdate();
p_statement.close();
}
public void update() throws SQLException
{
PreparedStatement p_statement = connection.prepareStatement("UPDATE STUDENT SET name=?, gender=?, age=?, java=?, database=?, data_structure=?, total=? WHERE student_id=?;");
p_statement.setString(1, this.name);
p_statement.setString(2, this.gender);
p_statement.setInt(3, age);
p_statement.setBigDecimal(4, java);
p_statement.setBigDecimal(5, database);
p_statement.setBigDecimal(6, data_structure);
p_statement.setBigDecimal(7, total);
p_statement.setString(8, this.student_id);
p_statement.executeUpdate();
p_statement.close();
}
public void delete() throws SQLException
{
PreparedStatement p_statement = connection.prepareStatement("DELETE FROM STUDENT WHERE student_id=?;");
p_statement.setString(1, this.student_id);
p_statement.executeUpdate();
p_statement.close();
}
public Vector<Vector<Object>> getDataVector()
{
Vector<Vector<Object>> table_data_vector = new Vector<>();
try {
for (this.selectAll(); this.next(); table_data_vector.add(this.getRowVector()))
;
} catch (SQLException e) {
JOptionPane.showMessageDialog(null, String.format("异常信息:\n%s\n%s", e.toString(), e.getStackTrace()), "运行时异常", JOptionPane.ERROR_MESSAGE);
System.exit(0);
}
return table_data_vector;
}
public Vector<Object> getRowVector()
{
Vector<Object> row_vector = new Vector<>();
row_vector.add(this.student_id);
row_vector.add(this.name);
row_vector.add(this.gender);
row_vector.add(this.age);
row_vector.add(this.java);
row_vector.add(this.database);
row_vector.add(this.data_structure);
row_vector.add(this.total);
return row_vector;
}
public Vector<Object> getTitleVector()
{
Vector<Object> title_vector = new Vector<>();
for (String title : this.column_titles)
title_vector.add(title);
return title_vector;
}
public BigDecimal getTotal()
{
this.total = this.java.add(this.database).add(this.data_structure);
return this.total;
}
public BigDecimal getValidScore(String input) throws NumberFormatException
{
BigDecimal temp = new BigDecimal(input);
temp = temp.setScale(2, RoundingMode.HALF_UP);
if (temp.compareTo(this.__0__) == -1 || temp.compareTo(this.__100__) == 1) throw new NumberFormatException("成绩应在合理区间内");
return temp;
}
}
public static void init()
{
try {
Class.forName(JDriver);
} catch (java.lang.ClassNotFoundException e) {
System.out.println("ForName: " + e.getMessage());
}
try {
Connection connection = DriverManager.getConnection(conURL);
Statement statement = connection.createStatement();
String query = "CREATE TABLE STUDENT("
+ "id INTEGER NOT NULL UNIQUE PRIMARY KEY AUTOINCREMENT,"
+ "student_id CHARACTER(10) NOT NULL UNIQUE,"
+ "name VARCHAR(16) NOT NULL,"
+ "gender VARCHAR(8),"
+ "age INTEGER,"
+ "java DECIMAL(3, 2),"
+ "database DECIMAL(3, 2),"
+ "data_structure DECIMAL(3, 2),"
+ "total DECIMAL(3, 2)"
+ ");";
query += "CREATE INDEX index_student ON STUDENT (id, student_id, name);";
statement.executeUpdate(query);
statement.close();
connection.close();
} catch (SQLException e) {
JOptionPane.showMessageDialog(null, String.format("异常信息:\n%s\n%s", e.toString(), e.getStackTrace()), "运行时异常", JOptionPane.ERROR_MESSAGE);
}
}
public StudentInfoManage()
{
try {
Class.forName(JDriver);
this.connection = DriverManager.getConnection(conURL);
} catch (java.lang.ClassNotFoundException e) {
JOptionPane.showMessageDialog(null, String.format("异常信息:\n%s\n%s", e.toString(), e.getStackTrace()), "运行时异常", JOptionPane.ERROR_MESSAGE);
System.exit(0);
} catch (SQLException e) {
JOptionPane.showMessageDialog(null, String.format("异常信息:\n%s\n%s", e.toString(), e.getStackTrace()), "运行时异常", JOptionPane.ERROR_MESSAGE);
System.exit(0);
}
}
protected void finalize()
{
try {
this.connection.close();
} catch (SQLException e) {
JOptionPane.showMessageDialog(null, String.format("异常信息:\n%s\n%s", e.toString(), e.getStackTrace()), "运行时异常", JOptionPane.ERROR_MESSAGE);
System.exit(0);
}
}
public void display()
{
this.student_info = new StudentInfo();
this.frame = new JFrame("学生信息管理系统");
this.frame.setSize(1080, 500);
this.frame.setLocation(100, 200);
this.frame.setBackground(Color.lightGray);
this.frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.frame.setLayout(new GridBagLayout());
GridBagConstraints gbc = new GridBagConstraints();
gbc.anchor = GridBagConstraints.CENTER;
gbc.fill = GridBagConstraints.BOTH;
gbc.insets = new Insets(5, 5, 5, 5);
this.lable_student_id = new JLabel("学号", JLabel.RIGHT);
this.lable_name = new JLabel("姓名", JLabel.RIGHT);
this.lable_gender = new JLabel("性别", JLabel.RIGHT);
this.lable_age = new JLabel("年龄", JLabel.RIGHT);
this.lable_java = new JLabel("Java-成绩", JLabel.RIGHT);
this.lable_database = new JLabel("数据库-成绩", JLabel.RIGHT);
this.lable_data_structure = new JLabel("数据结构-成绩", JLabel.RIGHT);
this.lable_total = new JLabel("总成绩", JLabel.RIGHT);
this.field_student_id = new JTextField();
this.field_name = new JTextField();
this.field_age = new JTextField();
this.field_java = new JTextField();
this.field_database = new JTextField();
this.field_data_structure = new JTextField();
this.field_total = new JTextField();
this.field_total.setEditable(false);
this.button_refresh = new JButton("刷新");
this.button_add = new JButton("添加");
this.button_add.setEnabled(false);
this.button_alter = new JButton("修改");
this.button_alter.setEnabled(false);
this.button_delete = new JButton("删除");
this.button_delete.setEnabled(false);
this.combo_box_gender = new JComboBox<String>(StudentInfoManage.gender);
this.combo_box_gender.setEditable(true);
;
this.table = new JTable(this.student_info.getDataVector(), this.student_info.getTitleVector())
{
private static final long serialVersionUID = -3960132093510822116L;
public boolean isCellEditable(int row, int column)
{
return false;
};
};
this.table.setSelectionMode(ListSelectionModel.SINGLE_SELECTION);
this.table.getTableHeader().setReorderingAllowed(false);
this.scroll_pane_table = new JScrollPane(this.table);
Container frame_contain = this.frame.getContentPane();
addComponent(this.lable_student_id, frame_contain, gbc, 0, 6, 1, 1, 1, 1);
addComponent(this.lable_name, frame_contain, gbc, 1, 6, 1, 1, 1, 1);
addComponent(this.lable_gender, frame_contain, gbc, 2, 6, 1, 1, 1, 1);
addComponent(this.lable_age, frame_contain, gbc, 3, 6, 1, 1, 1, 1);
addComponent(this.lable_java, frame_contain, gbc, 4, 6, 1, 1, 1, 1);
addComponent(this.lable_database, frame_contain, gbc, 5, 6, 1, 1, 1, 1);
addComponent(this.lable_data_structure, frame_contain, gbc, 6, 6, 1, 1, 1, 1);
addComponent(this.lable_total, frame_contain, gbc, 7, 6, 1, 1, 1, 1);
addComponent(this.field_student_id, frame_contain, gbc, 0, 7, 4, 1, 4, 1);
addComponent(this.field_name, frame_contain, gbc, 1, 7, 4, 1, 4, 1);
addComponent(this.field_age, frame_contain, gbc, 3, 7, 1, 1, 1, 1);
addComponent(this.field_java, frame_contain, gbc, 4, 7, 1, 1, 1, 1);
addComponent(this.field_database, frame_contain, gbc, 5, 7, 1, 1, 1, 1);
addComponent(this.field_data_structure, frame_contain, gbc, 6, 7, 1, 1, 1, 1);
addComponent(this.field_total, frame_contain, gbc, 7, 7, 1, 1, 1, 1);
addComponent(this.combo_box_gender, frame_contain, gbc, 2, 7, 3, 1, 3, 1);
addComponent(this.button_refresh, frame_contain, gbc, 4, 9, 2, 1, 2, 1);
addComponent(this.button_add, frame_contain, gbc, 5, 9, 2, 1, 2, 1);
addComponent(this.button_alter, frame_contain, gbc, 6, 9, 2, 1, 2, 1);
addComponent(this.button_delete, frame_contain, gbc, 7, 9, 2, 1, 2, 1);
addComponent(this.scroll_pane_table, frame_contain, gbc, 0, 0, 6, 8, 20, 10);
this.field_student_id.addCaretListener(this);
this.field_name.addCaretListener(this);
this.field_age.addActionListener(this);
this.field_java.addActionListener(this);
this.field_database.addActionListener(this);
this.field_data_structure.addActionListener(this);
this.field_total.addActionListener(this);
this.combo_box_gender.addActionListener(this);
this.button_refresh.addActionListener(this);
this.button_add.addActionListener(this);
this.button_alter.addActionListener(this);
this.button_delete.addActionListener(this);
this.table.getSelectionModel().addListSelectionListener(this);
this.frame.setVisible(true);
}
private void addComponent(Component c, Container contain, GridBagConstraints gbc, int row, int column, int width, int height, double weightx, double weighty)
{
gbc.gridy = row;
gbc.gridx = column;
gbc.gridwidth = width;
gbc.gridheight = height;
gbc.weightx = weightx;
gbc.weighty = weighty;
gbc.ipadx = 2;
gbc.ipady = 2;
contain.add(c, gbc);
}
private void refreshTable()
{
this.table.setModel(new DefaultTableModel(this.student_info.getDataVector(), this.student_info.getTitleVector()));
}
private void importField(boolean import_student_id_flag)
{
if (import_student_id_flag) this.field_student_id.setText(this.student_info.student_id);
this.field_name.setText(this.student_info.name);
this.combo_box_gender.setSelectedItem(this.student_info.gender);
this.field_age.setText(String.valueOf(this.student_info.age));
this.field_java.setText(this.student_info.java.toString());
this.field_database.setText(this.student_info.database.toString());
this.field_data_structure.setText(this.student_info.data_structure.toString());
this.field_total.setText(this.student_info.total.toString());
}
private void exportField() throws NumberFormatException
{
this.student_info.student_id = this.field_student_id.getText();
this.student_info.name = this.field_name.getText();
this.student_info.gender = String.valueOf(this.combo_box_gender.getSelectedItem());
this.student_info.age = Integer.valueOf(this.field_age.getText());
this.student_info.java = this.student_info.getValidScore(this.field_java.getText());
this.student_info.database = this.student_info.getValidScore(this.field_database.getText());
this.student_info.data_structure = this.student_info.getValidScore(this.field_data_structure.getText());
this.student_info.total = this.student_info.getTotal();
this.field_java.setText(this.student_info.java.toString());
this.field_database.setText(this.student_info.database.toString());
this.field_data_structure.setText(this.student_info.data_structure.toString());
this.field_total.setText(this.student_info.total.toString());
}
public void actionPerformed(ActionEvent event)
{
try {
if (this.button_refresh == event.getSource()) {
this.refreshTable();
} else if (this.button_add == event.getSource()) {
try {
this.exportField();
this.student_info.insert();
this.button_add.setEnabled(false);
this.button_alter.setEnabled(true);
this.button_delete.setEnabled(true);
this.refreshTable();
} catch (NumberFormatException e) {
JOptionPane.showMessageDialog(null, String.format("存在异常数据格式\n异常信息:\n%s\n%s", e.toString(), e.getStackTrace()), "运行时异常", JOptionPane.ERROR_MESSAGE);
}
} else if (this.button_alter == event.getSource()) {
try {
this.exportField();
this.student_info.update();
this.button_add.setEnabled(false);
this.button_alter.setEnabled(true);
this.button_delete.setEnabled(true);
this.refreshTable();
} catch (NumberFormatException e) {
JOptionPane.showMessageDialog(null, String.format("存在异常数据格式\n异常信息:\n%s\n%s", e.toString(), e.getStackTrace()), "运行时异常", JOptionPane.ERROR_MESSAGE);
}
} else if (this.button_delete == event.getSource()) {
try {
this.exportField();
this.student_info.delete();
this.button_add.setEnabled(true);
this.button_alter.setEnabled(false);
this.button_delete.setEnabled(false);
this.refreshTable();
} catch (NumberFormatException e) {
JOptionPane.showMessageDialog(null, String.format("存在异常数据格式\n异常信息:\n%s\n%s", e.toString(), e.getStackTrace()), "运行时异常", JOptionPane.ERROR_MESSAGE);
}
} else if (this.combo_box_gender == event.getSource()) {
this.student_info.gender = (String) this.combo_box_gender.getSelectedItem();
} else if (this.field_age == event.getSource()) {
this.student_info.age = Integer.valueOf(this.field_age.getText());
} else {
if (this.field_java == event.getSource()) {
this.student_info.java = this.student_info.getValidScore(this.field_java.getText());
this.field_java.setText(this.student_info.java.toString());
} else if (this.field_database == event.getSource()) {
this.student_info.database = this.student_info.getValidScore(this.field_database.getText());
this.field_database.setText(this.student_info.database.toString());
} else if (this.field_data_structure == event.getSource()) {
this.student_info.data_structure = this.student_info.getValidScore(this.field_data_structure.getText());
this.field_data_structure.setText(this.student_info.data_structure.toString());
}
this.field_total.setText(this.student_info.getTotal().toString());
}
} catch (SQLException e) {
JOptionPane.showMessageDialog(null, String.format("异常信息:\n%s\n%s", e.toString(), e.getStackTrace()), "运行时异常", JOptionPane.ERROR_MESSAGE);
} catch (NumberFormatException e) {
JOptionPane.showMessageDialog(null, String.format("数据格式输入异常\n异常信息:\n%s\n%s", e.toString(), e.getStackTrace()), "运行时异常", JOptionPane.ERROR_MESSAGE);
((JTextField) event.getSource()).setText("");
}
}
public void valueChanged(ListSelectionEvent event)
{
int index_row = this.table.getSelectedRow();
if (index_row == -1) return;
this.field_student_id.setText(this.table.getValueAt(index_row, 0).toString());
}
public void caretUpdate(CaretEvent event)
{
try {
if (this.field_student_id == event.getSource()) {
this.student_info.student_id = this.field_student_id.getText();
if (this.student_info.student_id.length() == 10) {
if (this.student_info.select(this.student_info.student_id)) {
this.importField(false);
this.button_add.setEnabled(false);
this.button_alter.setEnabled(true);
this.button_delete.setEnabled(true);
} else {
this.button_add.setEnabled(true);
this.button_alter.setEnabled(false);
this.button_delete.setEnabled(false);
}
} else {
this.button_add.setEnabled(false);
this.button_alter.setEnabled(false);
this.button_delete.setEnabled(false);
}
} else if (this.field_name == event.getSource()) {
this.student_info.name = this.field_name.getText();
}
} catch (SQLException e) {
JOptionPane.showMessageDialog(null, String.format("异常信息:\n%s\n%s", e.toString(), e.getStackTrace()), "运行时异常", JOptionPane.ERROR_MESSAGE);
System.exit(0);
}
}
public void itemStateChanged(ItemEvent event)
{
if (this.combo_box_gender == event.getSource()) {
this.student_info.gender = (String) this.combo_box_gender.getSelectedItem();
}
}
}