作者: kagula
发起日期: 2020-11-17
最后更新日期: 2020-12-08
概要
介绍Android java的开发, 这里假设你已经知道如何使用Android Studio运行你的android程序。
这里通过在第一个页面中加入text, 然后跳转到新的页面这样一个例子,建立android开发所需要的一个基础概念。
读者对象为对android Java开发不是很熟悉的专业程序员。
正文
学习环境
[1]Android Studio 4.1 英文版
[2]Microsoft Windows [版本 10.0.18363.1198]
基本概念
一个Android App可以由以下四个组件组成
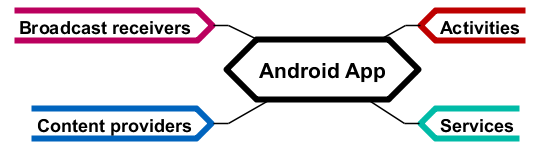
- 最常用的是Activities, 通过UI为用户服务, 例如我们用C++ MFC开发的桌面应用程序,每个activity可以看成是每张页面的处理逻辑。
- Services, 提供后台服务的程序, 例如VPN
- Content providers, 可以向其它App提供内容服务的程序, 例如电话号码本, 摄像头程序
- Broadcast receivers, 负责接收来自系统和其它App的通知信息
这四个组件是平等的, 所以Android App没有单一入口.本文主要介绍Activity的开发.
OS启动Android App的第一件事情是读取清单文件定位应用程序入口, Android清单文件存放在Android App项目的根目录中, 文件名为AndroidManifest.xml.
你必须在AndroidManifest.xml中声明所有上文中提到的四种组件, 这样OS才能知道要启动它们, AndroidManifest.xml中还做下图中指明的事情, 这样OS才能知道要如何配置VM运行你的App, 并为你的App分配资源.
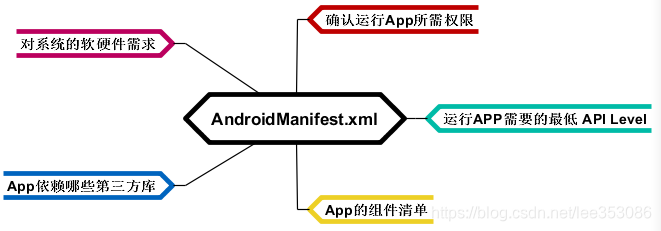
在AndroidManifest.xml中你还可以定义你的App名字, 桌面图标.
除了Broadcast Receiver可以在你的代码中动态创建, 其它Android App组件必须在清单中声明, 否则对OS来说是不可见的.
最重要的几个文件
新建项目Create New Project->Select a Project Template->Bottom Navigation Activity, 起名myfirstapp, 包名com.example。
熟悉下最重要的几个文件。
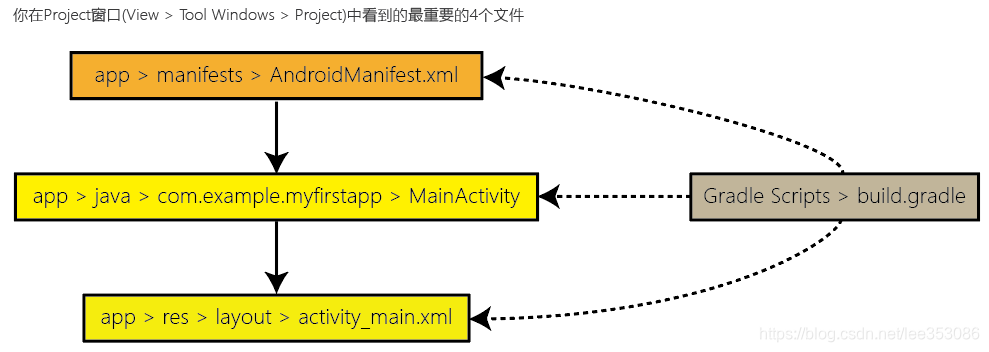
MainActivity是你默认的主Activity文件,对应的UI布局在activity_main.xml中定义。gradle负责如何build你的项目,有几个module(组件)就有几个build.gradle, 默认只有一个。
页面布局和字符串资源
我们第一个页面的布局和页面中的元素主要在activity_main.xml中设置, 双击这个xml文件我们就可以打开Android Studio内置的Layout Editor来编辑页面。
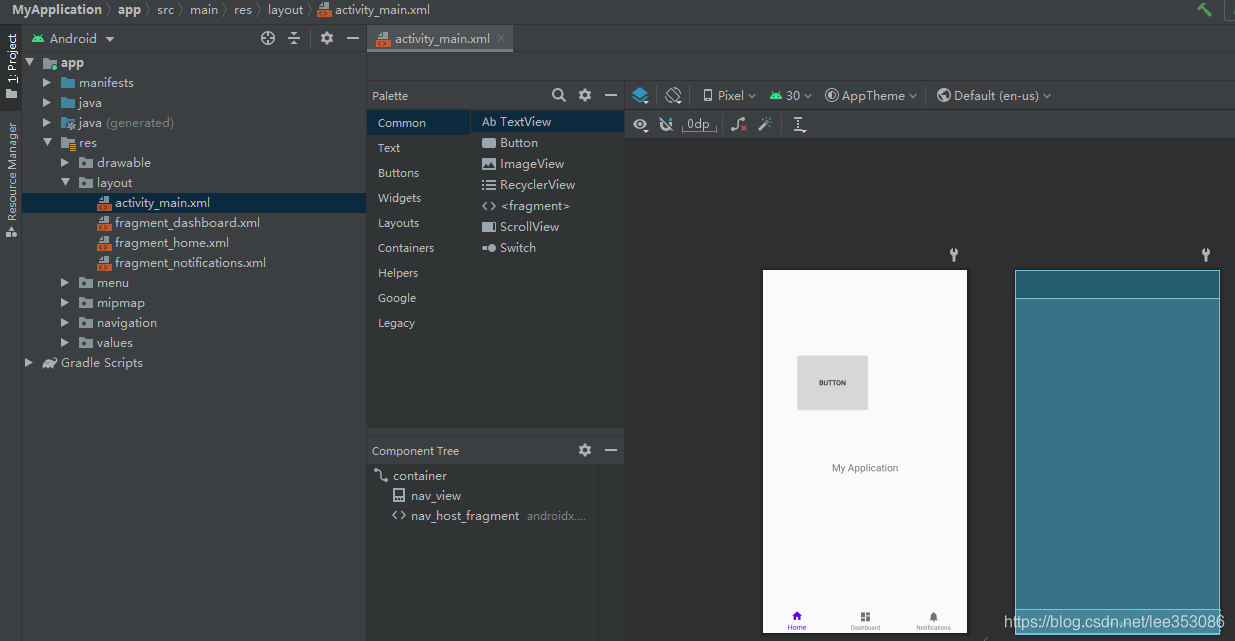
可以在这里选择设备(可以选择不同的dp,即设备分辨率)预览, 其它功能同Visual Studio C# WPF、Eclipse、QT Creator等图形化UI编辑功能差不多。
先在你的第一个页面上放一个“Plain Text”和“Button”控件,测试下constraint、baseline等各种对齐功能。
打开文件“[Project]->[app]->[res]->[values]->[strings.xml]”,熟悉文件内容后,右上角“open editor”打开“Translations Editor”,熟悉界面后“Add Key”,参考下面的截图添加“edit_message”,“button_send”
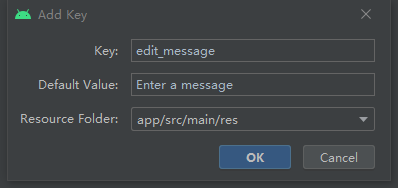
“button_send”的默认值设为"send".
回到布局编辑器, 清空你的按钮和文本输入控件的text属性, 然后在hint属性中绑定新添加的字符串资源。
运行你的App, 现在你的页面程序中可以看到Text和按钮控件了。
Activity和Fragment
可以把Activity看成是一个页面,Fragment看成子页面。一个页面可以含零个或多个fragment,也可以不含fragment。一个页面也可以当前只显示它所拥有的其中一个fragment,然后再多个fragment中切换显示。一个fragment也可以在多个activity中共享。
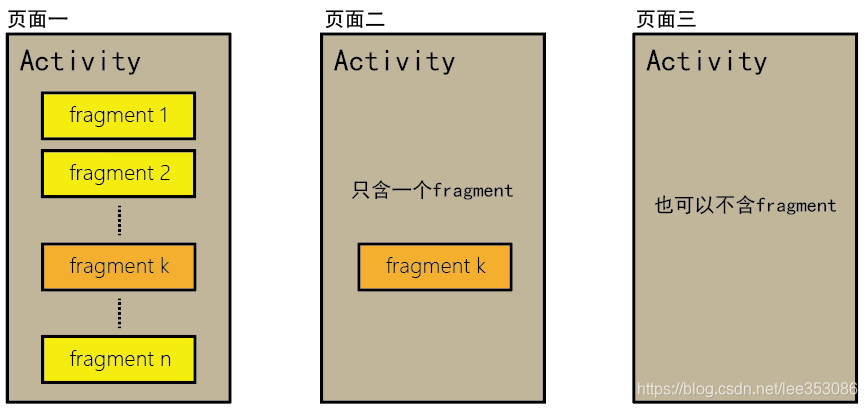
每个页面或子页面(fragment)至少由一个java和相应的UI布局xml文件组成,这个java文件就是一个activity类或则fragment类。
例如向导默认生成的fragment_home.xml就有对应的“com.example.myapplication.ui.home.HomeFragment.java”,你在project窗口中可以看到它。
现在尝试下页面跳转
为MainActivity.java添加按钮的onClick事件响应,代码如下
package com.example.myapplication;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import com.google.android.material.bottomnavigation.BottomNavigationView;
import androidx.appcompat.app.AppCompatActivity;
import androidx.navigation.NavController;
import androidx.navigation.Navigation;
import androidx.navigation.ui.AppBarConfiguration;
import androidx.navigation.ui.NavigationUI;
public class MainActivity extends AppCompatActivity {
public static final String EXTRA_MESSAGE = "com.example.myfirstapp.MESSAGE";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
BottomNavigationView navView = findViewById(R.id.nav_view);
// Passing each menu ID as a set of Ids because each
// menu should be considered as top level destinations.
AppBarConfiguration appBarConfiguration = new AppBarConfiguration.Builder(
R.id.navigation_home, R.id.navigation_dashboard, R.id.navigation_notifications)
.build();
NavController navController = Navigation.findNavController(this, R.id.nav_host_fragment);
NavigationUI.setupActionBarWithNavController(this, navController, appBarConfiguration);
NavigationUI.setupWithNavController(navView, navController);
}
/** Called when the user taps the Send button */
public void sendMessage(View view) {
Log.d("Debug", "MainActivity've been received a click event!");
Intent intent = new Intent(this, DisplayMessageActivity.class);
EditText editText = (EditText) findViewById(R.id.editText);
String message = editText.getText().toString();
intent.putExtra(EXTRA_MESSAGE, message);
startActivity(intent);
}
}
然后在相应的fragment_home.xml中为新添加的按钮,设置onClick事件响应函数为sendMessage,由于在相应的fragment java代码中没有该方法函数, 会出现警告不用理他。
这里的Intent实例可以看成是两个码头之间的渡轮,用来运货的,码头就是页面。除了DisplayMessageActivity这个class由于我们还没有创建,其它警告都可以通过“import class”来解决。
[project]->[New]->[Activity]->[Empty Activity],新建名为“DisplayMessageActivity”的activity, 消除sendMessage方法中的警告。
新建activity,Android Studio会为你做以下三件事:
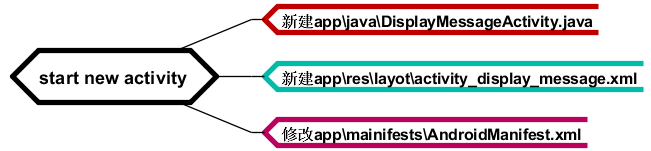
Android Studio修改AndroidManifest.xml是为了让Android操作系统知道新的activity的存在。
为了返回上一级activity,我们还需要对新添加的activity在AndroidManifest.xml中做如下修改
<activity android:name=".DisplayMessageActivity" android:parentActivityName=".MainActivity">
<!-- The meta-data tag is required if you support API level 15 and lower -->
<meta-data
android:name="android.support.PARENT_ACTIVITY"
android:value=".MainActivity" />
</activity>
为了显示从上个页面传过来的字符串,在新的activity页面中加入textView控件,然后在DisplayMessageActivity.java中加入代码如下。
package com.example.myapplication;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.widget.TextView;
public class DisplayMessageActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_display_message);
// Get the Intent that started this activity and extract the string
Intent intent = getIntent();
String message = intent.getStringExtra(MainActivity.EXTRA_MESSAGE);
// Capture the layout's TextView and set the string as its text
TextView textView = findViewById(R.id.textView);
textView.setText(message);
}
}
然后运行我们的第一个android app吧!
在第一个页面的text控件中输入文字, 然后点击Send按钮, 跳转到了第二个页面, 显示第一个页面输入过的文字。
重要快捷键
Alt+Enter 代码编辑器中自动补齐依赖
参考资料
[1]《Developer Guides》
https://developer.android.com/guide?utm_source=android-studio
[2]《Start another activity》
https://developer.android.com/training/basics/firstapp/starting-activity