- dva是基于redux和redux-saga(类似于redux-thunk的中间件)的数据流方案,然后为了简化开发体验,dva还额外内置了react-router和fetch
// model.ts
import React from 'react';
import {
Reducer, Effect, Subscription } from 'umi';
export type UserModelType = {
namespace: 'users',
state: {
},
reducers: {
getList: Reducer
},
effects: {
getList: Effect
},
subscriptions: {
getList: Subscription
}
}
const userModel = {
namespace: 'users',
state:{
},
reducers:{
getList( state, action ){
// state表示上一个状态(未更改)
// action = { type: '', payload: {} }
return {
...state,
...action.payload
}
}
},
effects:{
* getList( action, effects ){
// effects = { call: f, put: f, select: f }
// call(methodName) 调用service中的异步方法发请求
// put({type:'',payload}) // 调用reducers中的方法修改state 或者 调用effects中的方法重新发起请求(注意这里使用的是put,因为effects中的方法体中会有call)
// select(state => {...}) // 使用select可以获取当前state中的数据
yield put()
}
},
subscriptions:{
// 订阅
setup( {
dispatch, history }, done ){
return history.listen(({
pathname}) => {
if(pathname = '/'){
// 监听路由变化
dispatch({
type:'getList'
})
}
})
}
}
}
// 组件中使用 Users.tsx
import {
connect, UserModelType, Loading } from 'umi'; // Loading 是 stateMapToProps 中 loading对象的类型
// UserModelType 从model.ts中导出的类型可以直接从umi中导入!!!
const User = (props) => {
const [ record, setRecord ] = useState<SingleUserType | undefined>(undefined) // 为record添加类型校验
console.log(props.dataSource) // dataSource是state对象中namespace为users的状态里的数据 // 注意:props接收state的同时也接收到了dispatch(props.dispatch),可以通过dispatch({type:'',payload:{}})进行异步操作或修改state
return <div>用户</div>
}
const stateMapToProps = (state) => {
// 接收state作为参数
return {
users: state.users, // users对应namespace
loading: state.loading.models.users // loading(Object) 加载状态 (请求是否结束)
}
}
// 将stateMapToProps返回的值传入组件作为props
export default connect(stateMapToProps)(User) // User是组件名
// data.d.ts ts类型文件
export type singleUserType = {
name: string,
age: number,
id: string
}
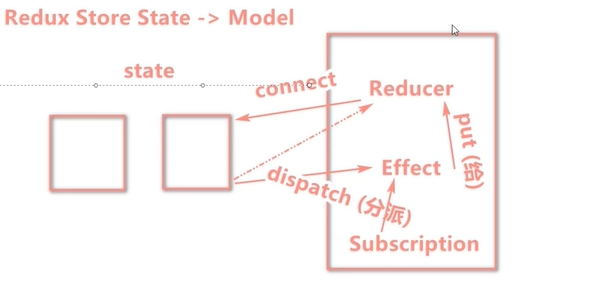