C#客户端连接阿里云物联网平台
创建新项目 Windows窗口应用(.NET Framework)
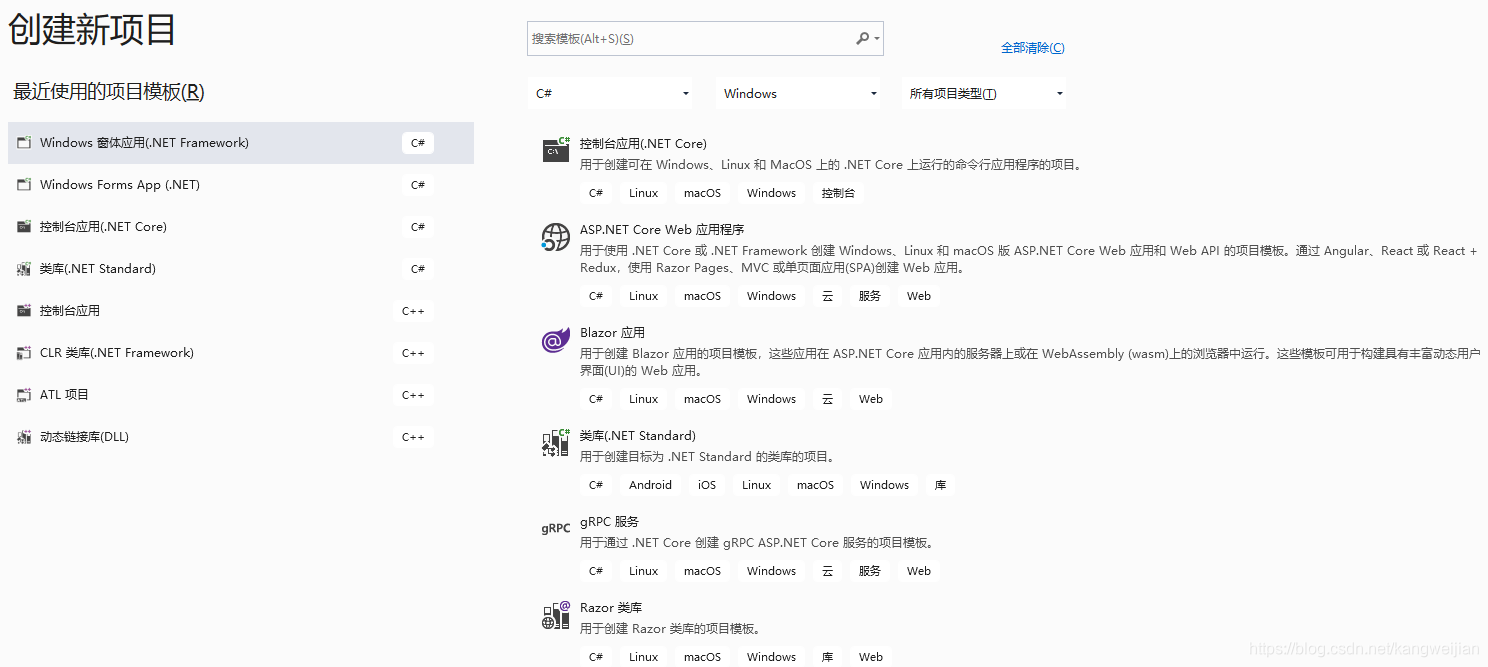
管理NuGet程序包
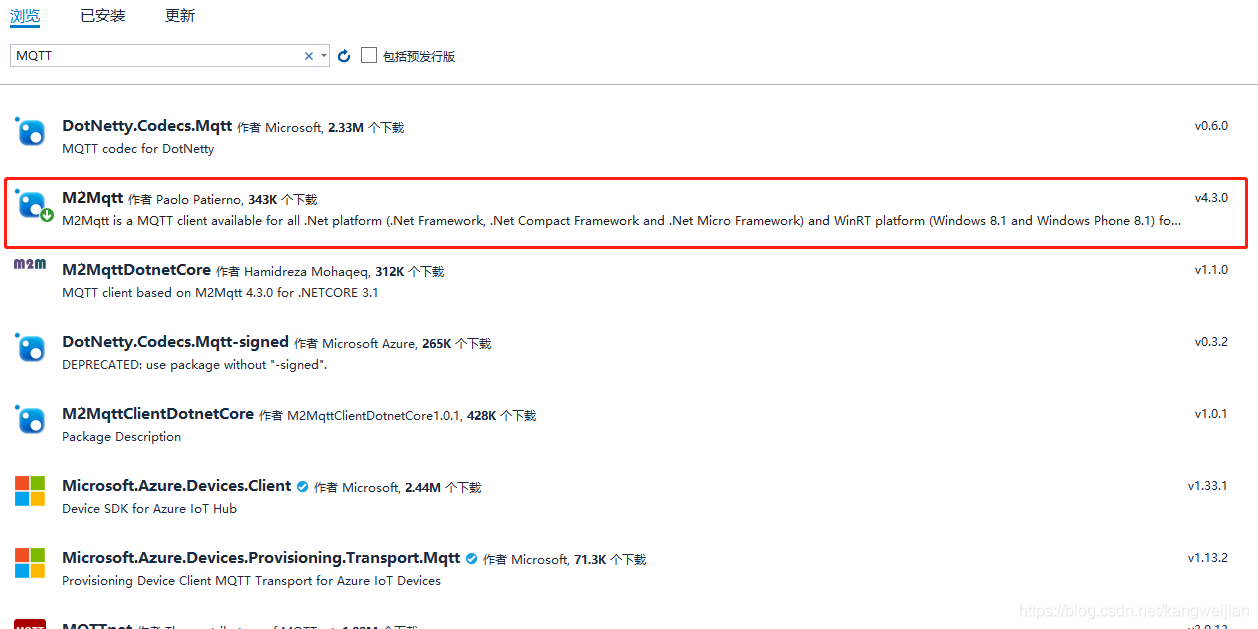
添加MqttSign.cs
using System;
using System.Security.Cryptography;
namespace WaterSamplePCNet
{
class CryptoUtil
{
public static String hmacSha256(String plainText, String key)
{
var encoding = new System.Text.UTF8Encoding();
byte[] plainTextBytes = encoding.GetBytes(plainText);
byte[] keyBytes = encoding.GetBytes(key);
HMACSHA256 hmac = new HMACSHA256(keyBytes);
byte[] sign = hmac.ComputeHash(plainTextBytes);
return BitConverter.ToString(sign).Replace("-", string.Empty);
}
}
public class MqttSign
{
private String username = "";
private String password = "";
private String clientid = "";
public String getUsername() {
return this.username; }
public String getPassword() {
return this.password; }
public String getClientid() {
return this.clientid; }
public bool calculate(String productKey, String deviceName, String deviceSecret)
{
if (productKey == null || deviceName == null || deviceSecret == null)
{
return false;
}
this.username = deviceName + "&" + productKey;
String timestamp = Convert.ToInt64((DateTime.UtcNow - new DateTime(1970, 1, 1)).TotalMilliseconds).ToString();
String plainPasswd = "clientId" + productKey + "." + deviceName + "deviceName" +
deviceName + "productKey" + productKey + "timestamp" + timestamp;
this.password = CryptoUtil.hmacSha256(plainPasswd, deviceSecret);
this.clientid = productKey + "." + deviceName + "|" + "timestamp=" + timestamp +
",_v=paho-c#-1.0.0,securemode=2,signmethod=hmacsha256|";
return true;
}
}
}
全局变量
string productKey = "a1X2bEn****";
string deviceName = "example1";
string deviceSecret = "ga7XA6KdlEeiPXQPpRbAjOZXwG8y****";
MqttClient mqttClient = null;
连接
private void connectBtn_Click(object sender, EventArgs e)
{
MqttSign sign = new MqttSign();
sign.calculate(productKey, deviceName, deviceSecret);
Console.WriteLine("username: " + sign.getUsername());
Console.WriteLine("password: " + sign.getPassword());
Console.WriteLine("clientid: " + sign.getClientid());
int port = 443;
string broker = productKey + ".iot-as-mqtt.cn-shanghai.aliyuncs.com";
mqttClient = new MqttClient(broker, port, true, MqttSslProtocols.TLSv1_2, null, null);
mqttClient.Connect(sign.getClientid(), sign.getUsername(), sign.getPassword());
Console.WriteLine("Broker: " + broker + " Connected");
}
订阅
private void subscribeBtn_Click(object sender, EventArgs e)
{
string topicReply = "/sys/" + productKey + "/" + deviceName + "/thing/event/property/post_reply";
mqttClient.MqttMsgPublishReceived += MqttPostProperty_MqttMsgPublishReceived;
mqttClient.Subscribe(new string[] {
topicReply }, new byte[] {
MqttMsgBase.QOS_LEVEL_AT_MOST_ONCE });
}
private static void MqttPostProperty_MqttMsgPublishReceived(object sender, uPLibrary.Networking.M2Mqtt.Messages.MqttMsgPublishEventArgs e)
{
Console.WriteLine("reply topic :" + e.Topic);
Console.WriteLine("reply payload:" + e.Message.ToString());
}
发布
private void publishBtn_Click(object sender, EventArgs e)
{
string topic = "/sys/" + productKey + "/" + deviceName + "/thing/event/property/post";
string message = "{\"id\":\"1\",\"version\":\"1.0\",\"params\":{\"LightSwitch\":0}}";
mqttClient.Publish(topic, Encoding.UTF8.GetBytes(message));
}
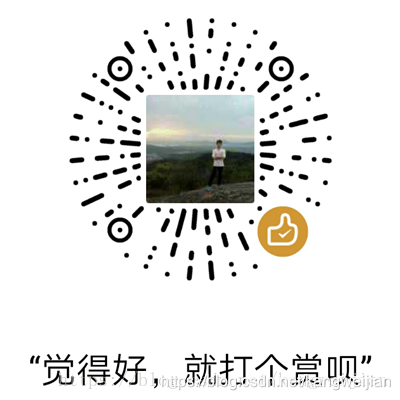