一、描叙
高可用性和高可靠性的存储服务,支持弹性扩容,服务 7x24 小时在线,按需收费,最大化节省存储成本。
二、注册申请
1、注册账号
注册完成后面 进入个人中心 完成个人实名认证
或者跳第二步 进入 存储对象创建空间,根据提示,快捷进入实名认证入口
2、选择 存储对象,然后 新建存储空间
创建完成,点击 【好的,我知道了】即可
3、创建空间完成过后自动获得测试域名
这个测试域名一般来说是用不山的, 测试域名主要作用于访问文件
4、找到域名管理,添加一个自己的访问域名
这里需要先用一个自己域名,我的是 xijia.plus, 然后我们添加二级域名指向七牛云提供的域名即可
1、添加域名,写入一个 xijia.plus 下的一个不存在的二级域名,我这里使用的是阿里云的域名
5、阿里云域名管理控制台中添加二级域名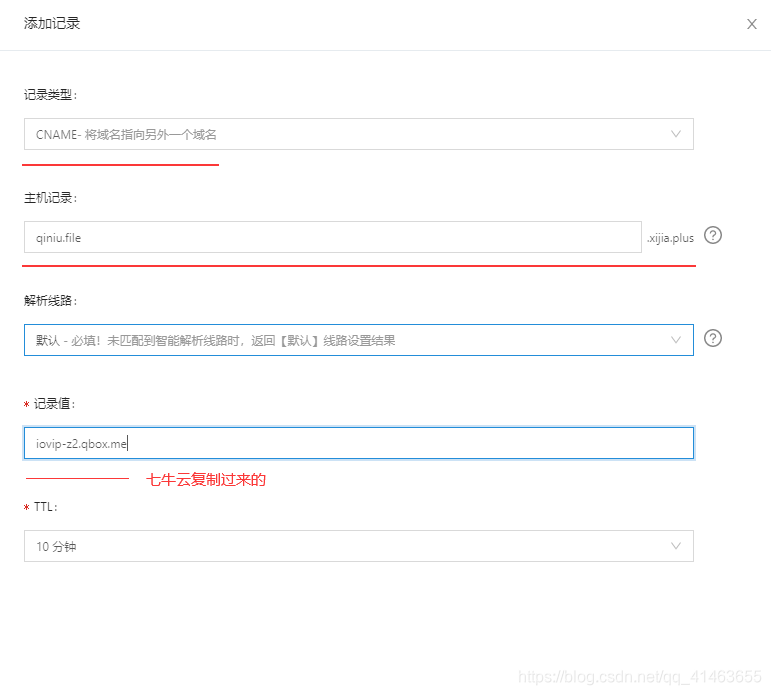
6、七牛云控制台默认访问域名,更换为新的域名
7、文件管理中上传一个文件,试一下能不能访问了,能访问了就ok了
8、如果想使用cdn 加速,和绑定新的访问地址流程类似
选择cdn 的绑定域名即可
三、代码实现
1、maven
<dependency>
<groupId>com.qiniu</groupId>
<artifactId>qiniu-java-sdk</artifactId>
<version>7.2.13</version>
</dependency>
2、yml 配置
## 七牛云
qiniu:
oss:
accessKey: "FetmKgfKFaS7ZAE12j5uzNSrXDkf6pp5LyAxtzWy"
secretKey: "iY74NEU14YUA3lDUb1vy3Rt18pnEQvTgx37WxH2r"
bucket: "*****"
hosts: "http://qiniu.file.xijia.plus/"
priKey: ""
## 地域(华东 z0 华北 z1 华南 z2 北美 na0 东南亚 as0)
area: "z2"
3、QiNiuUtils 基础信息配置类
package com.ws.ldy.modules.third.qiniu.config;
import com.ws.ldy.common.utils.ConsoleColors;
import lombok.Data;
import lombok.extern.slf4j.Slf4j;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.context.annotation.Configuration;
/**
* 七牛云 oss 配置类
* @author wangsong
* @date 2020/12/11 0011 17:10
* @return
* @version 1.0.0
*/
@SuppressWarnings("all")
@Configuration
@ConfigurationProperties(prefix = "qiniu.oss")
@Data
@Slf4j
public class QiNiuOssProperties {
public String accessKey; // 秘钥ak
public String secretKey; // 秘钥sk
public String bucket; // 自定义的bucket
public String hosts; // 访问域名
public String priKey; // 设置链接有效期 priKey
// 地域(华东 z0 华北 z1 华南 z2 北美 na0 东南亚 as0)
public String area;
public void println() {
// 打印加载信息
log.info(ConsoleColors.YELLOW_BRIGHT +
"\r\n" +
"|--- 七牛云 OSS配置加载成功 ---| \r\n" +
"| accessKey: {} \r\n" +
"| secretKey: {} \r\n" +
"| bucket: {} \r\n" +
"| hosts: {} \r\n" +
"| priKey: {} \r\n" +
"| area: {} \r\n" +
"| ----------------------------------|"
+ ConsoleColors.RESET, accessKey, secretKey, bucket, hosts, priKey, area);
}
}
4、QiNiuUtils 文件上传工具类
package com.ws.ldy.modules.third.qiniu.util;
import com.alibaba.fastjson.JSONObject;
import com.google.gson.Gson;
import com.qiniu.common.Zone;
import com.qiniu.http.Response;
import com.qiniu.storage.Configuration;
import com.qiniu.storage.UploadManager;
import com.qiniu.storage.model.DefaultPutRet;
import com.qiniu.util.Auth;
import com.ws.ldy.modules.third.qiniu.config.QiNiuOssProperties;
import com.ws.ldy.modules.third.qiniu.model.vo.TokenAndUrlVO;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import java.io.InputStream;
/**
* @author [email protected]
* @version 1.0
* @desc
* @date 2018/4/19
*/
@Slf4j
@Component
public class QiNiuUtils {
@Autowired
private QiNiuOssProperties qiNiuOssProperties;
/**
* 上传注册器
*/
private static UploadManager uploadManager = null;
/**
* 初始化上传管理器
*/
public void init() {
//上传管理器
if (uploadManager == null) {
// 华东 z0 华北 z1 华南 z2 北美 na0 东南亚 as0 文档:https://developer.qiniu.com/kodo/1671/region-endpoint-fq
Configuration cfg = null;
String area = qiNiuOssProperties.getArea();
if (area.equals("z0")) {
cfg = new Configuration(Zone.zone0());
} else if (area.equals("z1")) {
cfg = new Configuration(Zone.zone1());
} else if (area.equals("z2")) {
cfg = new Configuration(Zone.zone2());
} else if (area.equals("na0")) {
cfg = new Configuration(Zone.zoneNa0());
} else if (area.equals("as0")) {
cfg = new Configuration(Zone.zoneAs0());
}
uploadManager = new UploadManager(cfg);
}
}
/**
* 获取七牛云上传 token,用于前端直传使用
* @author wangsong
* @date 2020/10/16 0016 14:07
* @return java.lang.String
* @version 1.0.0
*/
public TokenAndUrlVO getUpToken() {
Auth auth = Auth.create(qiNiuOssProperties.getAccessKey(), qiNiuOssProperties.getSecretKey());
String upToken = auth.uploadToken(qiNiuOssProperties.getBucket());
TokenAndUrlVO vo = new TokenAndUrlVO();
vo.setToken(upToken);
vo.setHosts(qiNiuOssProperties.getHosts());
return vo;
}
/**
* 七牛云流文件上传
* @author wangsong
* @param uploadStream
* @param targetFileName
* @date 2020/12/25 0025 9:54
* @return java.lang.String
* @version 1.0.0
*/
public String uploadStream(InputStream uploadStream, String targetFileName) {
this.init();
if (targetFileName.startsWith("/")) {
targetFileName = targetFileName.replaceFirst("/", "");
}
Auth auth = Auth.create(qiNiuOssProperties.getAccessKey(), qiNiuOssProperties.getSecretKey());
try {
String upToken = auth.uploadToken(qiNiuOssProperties.getBucket());
Response response = uploadManager.put(uploadStream, targetFileName, upToken, null, null);
DefaultPutRet putRet = new Gson().fromJson(response.bodyString(), DefaultPutRet.class);
log.info("七牛云oss文件上传成功 {}", JSONObject.toJSONString(putRet));
return qiNiuOssProperties.getHosts() + targetFileName;
} catch (Exception ex) {
log.error("七牛云oss文件上传失败 {}", ex);
}
return null;
}
}
5、测试controller
@RestController
@Api(value = "QiNiuController", tags = "v-1.5 -- 文件管理 --> 七牛云OSS", consumes = BaseConstant.InterfaceType.PC_BASE)
@RequestMapping(BaseConstant.Sys.API + "/qiNiuOss")
public class QiNiuController extends BaseController {
@RequestMapping(value = "/findTokenAndUrl", method = RequestMethod.GET) //consumes = "multipart/*", headers = "content-type=multipart/form-data"
@ApiOperation("前端直传--获取七牛云上传Token和上传Url")
public R<TokenAndUrlVO> findToken() {
TokenAndUrlVO token = QiNiuUtils.getUpToken();
return R.success(token);
}
@RequestMapping(value = "/upload", method = RequestMethod.POST) //consumes = "multipart/*", headers = "content-type=multipart/form-data"
@ApiOperation("后端上传--OSS-文件上传,可在指定路径后追加子路径,以/结尾,返回完整可访问当前服务内网访问OSS的完整URL")
@ApiImplicitParams({
@ApiImplicitParam(name = "filePath", value = "文件路径,必须指定开头目录,当为任意文件时,后台不验证文件格式(" + "\r\n" +
"图片=image/" + "\r\n" +
"头像=image/head" + "\r\n" +
"音乐=music/" + "\r\n" +
"视频=video/" + "\r\n" +
"表格=excel/" + "\r\n" +
"PDF=pdf/" + "\r\n" +
"任意文件=file/" + "\r\n" +
")", required = true, example = "image/")
})
public R<String> upload(@RequestParam("file") MultipartFile file, @RequestParam("filePath") String filePath) {
// 验证文件格式及路径,并获取文件上传路径, file.getOriginalFilename()=原文件名
// 这行代码是获取最后上传到oss的文件名称和 文件验证,可自定义,可使用我的
String fileName = AliFileUtil.getPath(filePath, file.getOriginalFilename());
try {
// 上传
String url = QiNiuUtils.uploadStream(file.getInputStream(), fileName);
return R.success(url);
} catch (Exception e) {
return R.error(RType.FILE_UPLOAD_FAILED);
}
}
}
6、前端直传 vo 类
@Data
@ToString(callSuper = true)
@ApiModel(value = "TokenAndUrlVO 对象", description = "七牛云前端直传需要数据")
public class TokenAndUrlVO extends Convert {
@ApiModelProperty(notes = "上传接口" ,position = 0)
private String hosts;
@ApiModelProperty(notes = "上传token" ,position = 0)
private String token;
}
7、文件验证工具类 AliFileUtil
package com.ws.ldy.others.aliyun.oss.util;
import com.ws.ldy.common.utils.LocalDateTimeUtil;
import com.ws.ldy.config.error.ErrorException;
/**
* 上传文件后缀名处理工具类
* @author wangsong
* @date 2020/12/11 0011 14:01
* @return
* @version 1.0.0
*/
public class AliFileUtil {
// 文件保存路径地址
private final static String UPLOAD_PATH_IMAGE = "image"; // oss/file/image 图片
private final static String UPLOAD_PATH_MUSIC = "music"; // oss/file/music 音频
private final static String UPLOAD_PATH_VIDEO = "video"; // oss/file/video 视频
private final static String UPLOAD_PATH_EXCEL = "excel"; // oss/file/excel 表格
private final static String UPLOAD_PATH_PDF = "pdf"; // oss/file/pdf pdf文件
private final static String UPLOAD_PATH_FILE = "file"; // oss/file/file 任意文件
/**
* 上传路径文件格式判断
*
* @param filePath 文件上传路径 (上方静态变量)
* @param fileName 当前市场的文件名称
* @return fileName
*/
public static String getPath(String filePath, String fileName) {
if (filePath.lastIndexOf("/") != filePath.length() - 1) {
throw new ErrorException(10002, "路径必须已[/]结尾");
}
// 目录开头
String path = filePath.split("/")[0];
// 后缀名
String suffixName = fileName.substring(fileName.lastIndexOf(".") + 1, fileName.length());
if (UPLOAD_PATH_IMAGE.equals(path)) {
/**
* 图片 ( 图片重命名- (17位时间+3位随机数+原文件名称)-- 生成20位前缀
*/
if (!"jpg".equals(suffixName) && !"png".equals(suffixName) && !"jpeg".equals(suffixName) && !"gif".equals(suffixName)) {
throw new ErrorException(10002, "图片仅支持上传-[jpg,png,jpeg,jif]");
}
fileName = LocalDateTimeUtil.getTimeStr20() + "-" + fileName;
} else if (UPLOAD_PATH_MUSIC.equals(path)) {
/**
* 音频
*/
if (!"mp3".equals(suffixName)) {
throw new ErrorException(10002, "音乐仅支持上传-[mp3]");
}
} else if (UPLOAD_PATH_VIDEO.equals(path)) {
/**
* 视频
*/
if (!"mp4".equals(suffixName)) {
throw new ErrorException(10002, "视频仅支持上传-[mp4]");
}
} else if (UPLOAD_PATH_EXCEL.equals(path)) {
/**
* excel
*/
if (!"xlsx".equals(suffixName) && !"xls".equals(suffixName)) {
throw new ErrorException(10002, "EXCEL仅支持上传-[xlxs,xlx]");
}
} else if (UPLOAD_PATH_PDF.equals(path)) {
/**
* pdf
*/
if (!"pdf".equals(suffixName)) {
throw new ErrorException(10002, "PDF仅支持上传-[pdf]");
}
} else if (UPLOAD_PATH_FILE.equals(path)) {
/**
* 任意文件,不做限制
*/
} else {
throw new ErrorException(10002, "路径错误");
}
// 空格+逗号+括号+一些特殊符号 统一转为_,部分地方无法解析,如逗号分隔url, editor 编辑器, URL请求空格问题
fileName = fileName
.replaceAll(" ", "_")
.replace(",", "_")
.replaceAll("\\(", "_")
.replaceAll("\\)", "_")
.replaceAll("#", "_")
.replaceAll("@", "_");
return fileName;
}
-
个人开源项目(通用后台管理系统)–> https://gitee.com/wslxm/spring-boot-plus2 , 喜欢的可以看看
-
本文到此结束,如果觉得有用,动动小手点赞或关注一下呗,将不定时持续更新更多的内容…,感谢大家的观看!