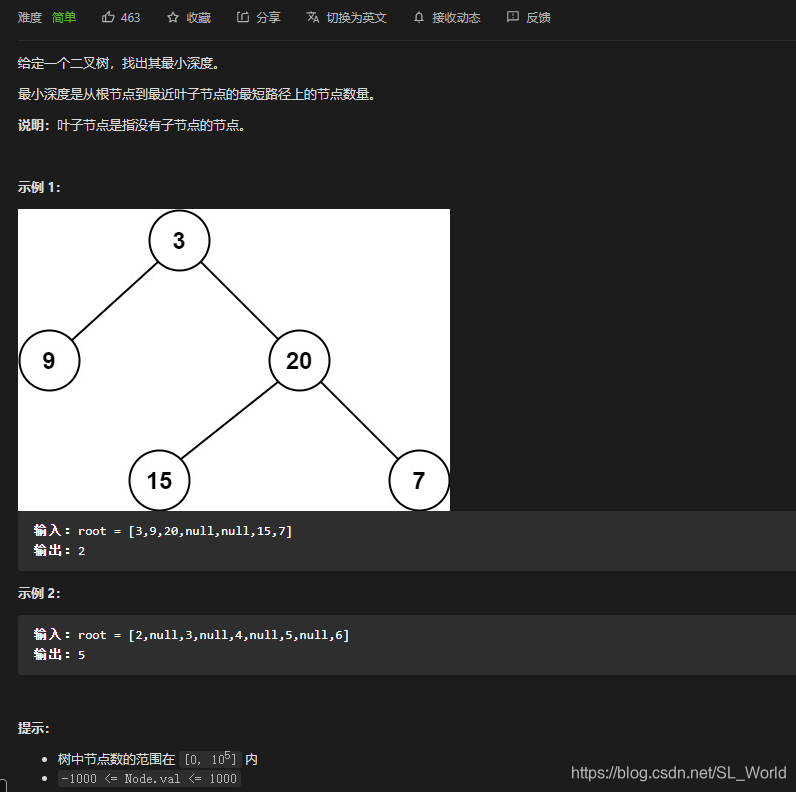
1 BFS(在最小深度问题上不用遍历所有树节点)
class Solution {
public:
int minDepth(TreeNode* root) {
if (!root) return 0;
queue<TreeNode*> q;
q.push(root);
int depth = 1;
while (!q.empty()) {
int sz = q.size();
for (int i=0;i < sz;i++) {
TreeNode* e_ptr = q.front(); q.pop();
if (!e_ptr->left && !e_ptr->right)
return depth;
if (e_ptr->left) q.push(e_ptr->left);
if (e_ptr->right) q.push(e_ptr->right);
}
depth++;
}
return depth;
}
};
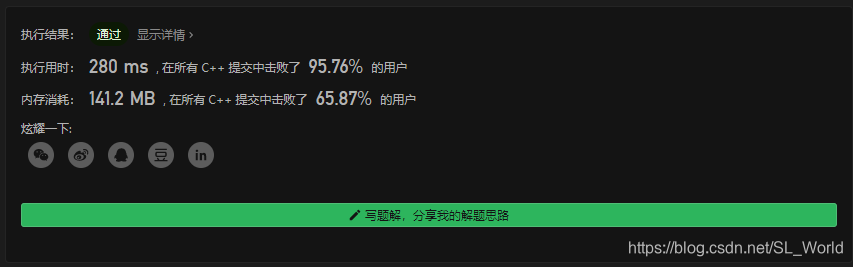
2 DFS(需要遍历所有树节点)
class Solution {
private:
int min_depth = 1e4;
public:
void dfs(TreeNode* root, int depth) {
if (!root->left && !root->right && depth < min_depth)
min_depth = depth;
if (root->left)
dfs(root->left, depth + 1);
if (root->right)
dfs(root->right, depth + 1);
}
int minDepth(TreeNode* root) {
if (!root) return 0;
dfs(root, 1);
return min_depth;
}
};
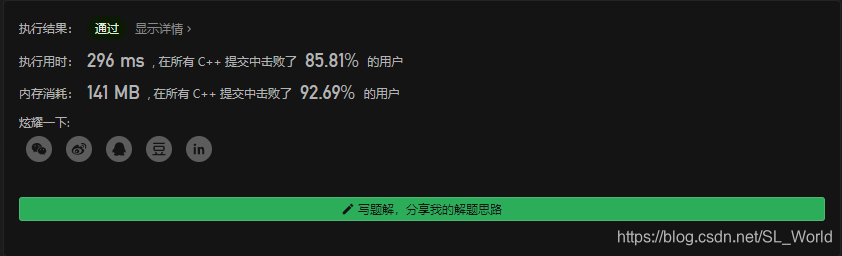