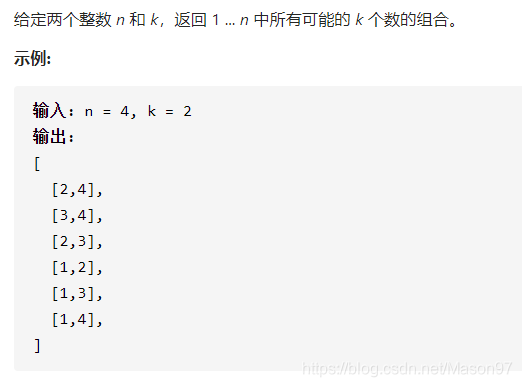
我用的方法是 搜索回溯的方法。
class Solution {
public static void main(String[] args) {
Solution s = new Solution();
List<List<Integer>> list = s.combine(4, 2);
System.out.println(list.toString());
}
public List<List<Integer>> combine(int n, int k) {
List<List<Integer>> res = new LinkedList<>();
boolean[] candidate = new boolean[n + 1];
for (int i = 1; i <= n; i++) {
LinkedList<Integer> tmp = new LinkedList<>();
tmp.add(i);
candidate[i] = true;
dfs(candidate, res, 1, k, tmp, n, i);
candidate[i] = false;
}
return res;
}
private void dfs(boolean[] candidate, List<List<Integer>> res, int count, int k, LinkedList<Integer> tmp, int n,int ii) {
if (count == k) {
LinkedList<Integer> clone = new LinkedList<>(tmp);
res.add(clone);
return;
}
if(ii == n) return;
for (int i = ii; i <= n; i++) {
if (!candidate[i]) {
tmp.add(i);
candidate[i] = true;
dfs(candidate, res, count + 1, k, tmp, n, i);
candidate[i] = false;
tmp.remove(tmp.size() - 1);
}
}
}
}
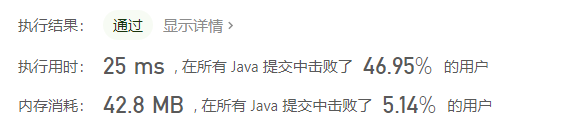
速度有点慢,考虑剪枝来提速一下:

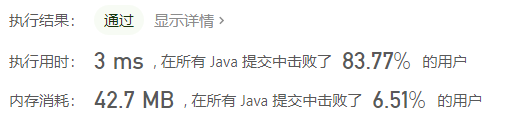
class Solution {
public static void main(String[] args) {
Solution s = new Solution();
List<List<Integer>> list = s.combine(4, 2);
System.out.println(list.toString());
}
public List<List<Integer>> combine(int n, int k) {
List<List<Integer>> res = new LinkedList<>();
boolean[] candidate = new boolean[n + 1];
for (int i = 1; i <= n; i++) {
LinkedList<Integer> tmp = new LinkedList<>();
tmp.add(i);
candidate[i] = true;
dfs(candidate, res, 1, k, tmp, n, i);
candidate[i] = false;
}
return res;
}
private void dfs(boolean[] candidate, List<List<Integer>> res, int count, int k, LinkedList<Integer> tmp, int n,
int ii) {
if (count == k) {
LinkedList<Integer> clone = new LinkedList<>(tmp);
res.add(clone);
return;
}
if ((n - ii) < (k - count)) return;
for (int i = ii; i <= n; i++) {
if (!candidate[i]) {
tmp.add(i);
candidate[i] = true;
dfs(candidate, res, count + 1, k, tmp, n, i);
candidate[i] = false;
tmp.remove(tmp.size() - 1);
}
}
}
}