一.单分支选择结构和if语句
#include <stdio.h>
#include <math.h>
int main()
{
double a = 3, b = 4, c = 5, s, area;
if (a + b > c && b + c > a && a + c > b)
{
s = (a + b + c) / 2;
area = sqrt(s * (s - a) * (s - b) * (s - c));
printf("area=%7.2f\n", area);
}
}
二.双分支选择结构和if语句
#include <stdio.h>
#include <math.h>
int main()
{
double result, n, d;
scanf_s("%lf %lf", &n, &d);
if (d != 0)
{
result = n / d;
printf("%f\n", result);
}
else
{
printf("除数不能为0!\n");
}
}
三.实例1:求三角形的面积的程序
#include <stdio.h>
#include <math.h>
int main()
{
double a = 3, b = 4, c = 5, s, area;
if (a + b > c && b + c > a && a + c > b)
{
s = (a + b + c) / 2;
area = sqrt(s * (s - a) * (s - b) * (s - c));
printf("area=%7.2f\n", area);
}
else
{
printf("三边数值不能构成三角形\n");
}
}
四.实例2:求一元二次方程的根
#include <stdio.h>
#include <math.h>
int main()
{
double a, b, c, x1, x2;
scanf_s("%lf %lf %lf", &a, &b, &c);
if ((b * b - 4 * a * c) >= 0)
{
x1 = (-b + sqrt(b * b - 4 * a * c)) / (2 * a);
x2 = (-b - sqrt(b * b - 4 * a * c)) / (2 * a);
printf("x1=%.2f\n", x1);
printf("x2=%.2f\n", x2);
}
else
{
printf("方程无实根!\n");
}
return 0;
}
运行结果:
-6 3 7
x1=-0.86
x2=1.36
五.使用if语句的典型错误
#include <stdio.h>
int main()
{
int a = 0;
if (a == 100);
printf("满分!\n");
}
#include <stdio.h>
int main()
{
int a = 0;
if (a == 100);
printf("满分!\n");
else
printf("再努力!");
}
#include <stdio.h>
int main()
{
int a = 0;
if (a == 100)
printf("满分!\n");
printf("哈哈!\n");
else
printf("再努力!");
}
六.实践项目
1.输入一个数,判断能否被3或5整除。如至少能被这两个数中的一个整除,则将此数打印出来,否则不打印。
#include <stdio.h>
int main()
{
int x;
scanf_s("%d", &x);
if (x % 3 == 0 || x % 5 == 0)
{
printf("%d\n", x);
}
}
2.输入整数a和b,若两数之和大于100,则输出两数和百位上的数字,否则输出两数之和。
#include <stdio.h>
int main()
{
int a, b, sum;
scanf_s("%d %d", &a, &b);
sum = a + b;
if (sum > 100)
{
sum /= 100;
sum %= 10;
printf("两数和百位上的数字为%d\n", sum);
}
else
{
printf("两数和为%d\n", sum);
}
}
3.编程序,计算分段函数的值并输出(x取整型即可)
#include <stdio.h>
int main()
{
int x, y;
scanf_s("%d", &x);
if (x >= 1)
{
y = x - 1;
}
else
{
y = -x + 1;
}
printf("y=%d\n", y);
}
4.写一程序,求y值 (x值由键盘输入,利用三角公式求值)。
#include <stdio.h>
#include <math.h>
int main()
{
double x, y;
const PI = 3.1415926;
scanf_s("%lf", &x);
x = x * PI / 180;
if (x >= 0)
{
y = (sin(x) + cos(x)) / 2;
}
else
{
y = (sin(x) - cos(x)) / 2;
}
printf("y=%.2f\n", y);
}
5.分段函数计算周工资
扫描二维码关注公众号,回复:
12979422 查看本文章
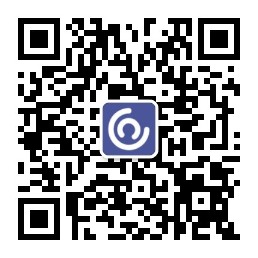
#include <stdio.h>
int main()
{
int rate, hour;
double salary;
scanf_s("%d %d", &rate, &hour);
if (hour > 40)
{
salary = 40 * rate + (hour - 40) * rate * 1.5;
}
else
{
salary = rate * hour;
}
printf("%.2f", salary);
}
6.输入小时和分,以hh:mm形式输出. 例:输入14 25,输出14:25,输入8 9,输出08:09
#include <stdio.h>
int main()
{
int h, m;
printf("输入小时和分钟");
scanf_s("%d %d", &h, &m);
printf("%02d:%02d\n", h, m);
}
#include <stdio.h>
int main()
{
int h, m;
printf("输入小时和分钟: ");
scanf_s("%d %d", &h, &m);
if (h < 10)
printf("0");
printf("%d:", h);
if (m < 10)
printf("0");
printf("%d\n", m);
}