1.属性分为两种:
一种是特性(固有属性),如id、class、src、value、href、alt、title等等;
一种是新增属性(自定义属性)
2.prop()只能获取固有属性,attr()可以获取所有属性,包括新增属性
<div id="test" class="myTest" myAttribute="ccy">div1</div>
<script src="./jQuery/jquery-3.6.0.js"></script>
<script>
console.log( $('div').attr('id'),
$('div').attr('class'),//获取固有属性
$('div').attr('myAttribute') );//获取新增属性
console.log( $('div').prop('id'),
$('div').prop('class'),
$('div').prop('myAttribute') );
</script>
效果:第一行是使用attr()获取的结果,第二行是使用prop()获取的结果,最后一列获取新增属性,prop()返回undefined
3.prop()对应js中的property,通常用点’.'调用
attr()对应js中的getAttribute()和setAttribute()
console.log(test.getAttribute('id'), test.getAttribute('class'), test.getAttribute('myAttribute'));
console.log(test.id, test.className, test.myAttribute);
用property获取class名时,需要用’.className’,用attribute时,只需要用’class’
效果同上
4.prop()和attr()取dom时,如果有多个可匹配的dom,只取第一个
<div id="test">div</div>
<div id="test2">div2</div>
console.log ( $('div').attr('id') );//只输出test
console.log ( $('div').prop('id') );//只输出test
5.prop()和attr()给dom设置属性可以影响所有被匹配的标签
prop()和attr()给dom设置属性时,如果有多个可匹配的dom,全部都被设置上属性;可以一次性设置一个属性或多个属性
5.1 设置一个属性:
<div>div1</div>
<div>div1</div>
<p>ppp</p>
<p>ppp</p>
<script src="./jQuery/jquery-3.6.0.js"></script>
<script>
//设置属性可影响一组
$('div').attr('class', 'test');
$('p').prop('class', 'test');
</script>
效果:class属性都设置上了
5.2 设置多个属性(参数可以是对象):
//设置多个属性
$('div').attr({
class: 'test',
myAttribute: 'ccy'});
$('p').prop({
class: 'test',
myAttribute: 'ccy'});
效果:一次性设置多个属性,由于prop只操作固有属性,所以myAttribute属性没设置上
6. 获取未设置的属性
attr()获取未设置的属性,返回undefined;
prop()获取未设置的固有属性,返回空值(prop()获取新增属性均返回undefined,不论是否被设置)
<div>div1</div>
<script src="./jQuery/jquery-3.6.0.js"></script>
<script>
console.log( $('div').attr('id'), $('div').attr('myAttribute'));//undefined undefined
console.log( $('div').prop('id'));//空值
</script>
7.attr()不能动态获取标签的状态,prop()可以
input标签未设置checked时,不论该input是否被选中,用attr()获取checked属性,均是返回undefined;
input标签设置checked时,不论该input是否被选中,用attr()获取checked属性,均是返回checked;
说明attr()只认明面上标签是否写了某属性,不能动态获取属性
<ul>喜欢吃的东西?
<li class="title">
<input type="checkbox"><!--没有设置checked属性-->
<span>以下都喜欢</span>
</li>
<li>
<input type="checkbox">
<span>苹果</span>
</li>
<li>
<input type="checkbox">
<span>香蕉</span>
</li>
<li>
<input type="checkbox">
<span>梨</span>
</li>
</ul>
<script src="./jQuery/jquery-3.6.0.js"></script>
<script>
$('.title input').change(function(){
console.log($('.title input').attr('checked'));
})//change捕获状态改变事件
</script>
效果:
当.title属性添加上checked时(checked为默认选中)
<li class="title">
<input type="checkbox" checked>
<span>以下都喜欢</span>
</li>
写成<input type="checkbox" checked='123'>
效果一样
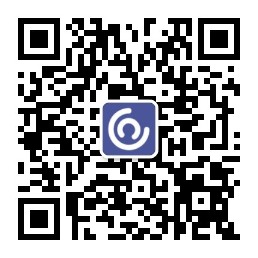
而当点击.title,该标签增加上checked属性时,prop能对此做出反应:
<ul>喜欢吃的东西?
<li class="title">
<input type="checkbox"><!--没有设置checked属性-->
<span>以下都喜欢</span>
</li>
<li>
<input type="checkbox">
<span>苹果</span>
</li>
<li>
<input type="checkbox">
<span>香蕉</span>
</li>
<li>
<input type="checkbox">
<span>梨</span>
</li>
</ul>
<script src="./jQuery/jquery-3.6.0.js"></script>
<script>
$('.title input').change(function(){
console.log($('.title input').prop('checked'));
})
</script>
效果:当从未选中状态变成选中状态时,返回true;从选中状态改成未选中状态时,返回false
标签被设置成默认选中状态也可以工作,功能一致:
因此,我们可以根据prop()返回的布尔值来判断这第一个选项是否被选中:如果被选中,所有的选项都要被选中;如果取消选中,所有的选项取消选中
$('.title input').change(function(){
// console.log($('.title input').prop('checked'));
if ( $('.title input').prop('checked') ){
$('ul input').prop('checked', true);//.title下的input被选中时,所有input都被选中
}else {
$('ul input').prop('checked', false);//.title下的input取消选中时,所有input都没被选中
}
})
效果:
8.移除属性removeAttr()、removeProp()
8.1 removeAttr():
给ul加一个class
然后移除掉这个属性:
$('ul').removeAttr('class');
效果:
增加一个新增属性:
$('ul').attr('aaa','aaa');
然后移除:
$('ul').removeAttr('aaa');
8.2 removeProp()只能移除由prop()设置的新增属性
prop()设置新增属性,虽然没有在标签中显示出来,没有对应上,但是设置还是设置了的,可以取这个属性值
$('ul').prop('aaa', 'aaa');
效果:并没有显示出来
当我取这个属性时:
console.log( $('ul').prop('aaa') );//输出为aaa
可见,设置是成功的,但是不显示
当我用removeProp()移除prop新增属性时:
$('ul').removeProp('aaa');
console.log( $('ul').prop('aaa') );//返回undefined
当我新增固有属性并移除时:
$('ul').prop('id', 'aaa');
console.log( $('ul').prop('id') );
$('ul').removeProp('id');
console.log( $('ul').prop('id') );
效果:并没有删除掉
所以,固有属性如果不需要了,建议设置成false或空,不移除