#include<stdlib.h>
#include<iostream>
#include<string>
using namespace std;
/*
4.5.2 左移运算符重载
可以输出自定义的数据类型
*/
class Person{
public:
int a;
int b;
// 尝试利用成员函数重载左移运算符 p.operator<<(cout) 简化为 p << cout
// 不会利用成员函数重载<<运算符,因为无法实现cout在左侧
// 成员函数 实现不了 p << cout 不是我们想要的效果
/*
void operator<<(Person & p){ // (cout)
}
*/
};
// 只能利用全局函数重载左移运算符
/*
void operator<<(ostream & cout, Person & p){ // 本质:operator<<(cout, p) 简化为 cout << p
cout << "a=" << p.a << ",b=" << p.b << endl;
}
*/
//ostream对象全局只能有一个
ostream & operator<<(ostream & cout, Person & p){ // 本质:operator<<(cout, p) 简化为 cout << p
cout << "a=" << p.a << ",b=" << p.b;
return cout;
}
// 本函数的cout可以改为out等其他名称,实质为起别名
void test1(){
Person p;
p.a = 10;
p.b = 10;
cout << p.a << endl;
cout << p.b << endl;
//cout << p; // 正常本行报错;重载<<后可以实现
cout << p << ",hello" << endl; // 链式编程思想
}
int main()
{
test1();
system("pause");
return 0;
}
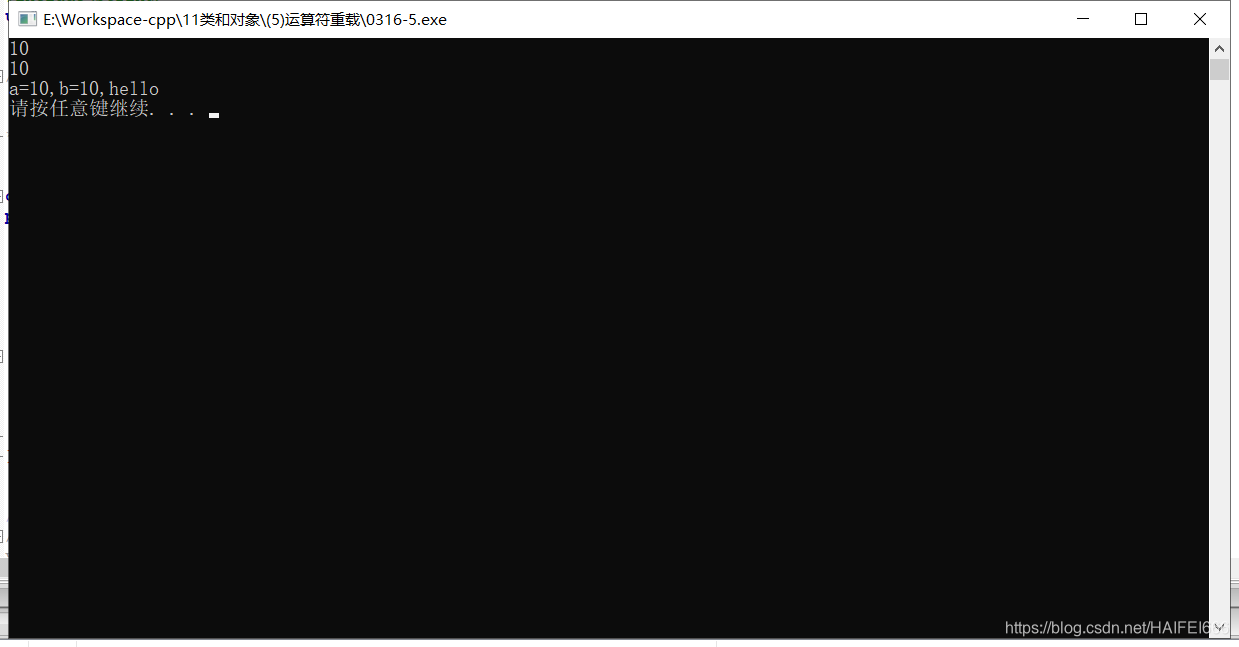
#include<stdlib.h>
#include<iostream>
#include<string>
using namespace std;
class Person{
friend ostream & operator<<(ostream & out, Person & p);
private:
int a;
int b;
public:
/*
Person(int _a, int _b){
a = _a;
b = _b;
}
*/
Person(int a, int b){ // 该函数效果实质同上
this->a = a;
this->b = b;
}
};
//ostream对象全局只能有一个
ostream & operator<<(ostream & out, Person & p){
out << "a=" << p.a << ",b=" << p.b;
return out;
}
void test1(){
Person p(10, 10);
cout << p << ",hello" << endl; // 链式编程思想
}
int main()
{
test1();
system("pause");
return 0;
}