一、.pro文件
QT += core gui network
greaterThan(QT_MAJOR_VERSION, 4): QT += widgets
CONFIG += c++11
# The following define makes your compiler emit warnings if you use
# any Qt feature that has been marked deprecated (the exact warnings
# depend on your compiler). Please consult the documentation of the
# deprecated API in order to know how to port your code away from it.
DEFINES += QT_DEPRECATED_WARNINGS
# You can also make your code fail to compile if it uses deprecated APIs.
# In order to do so, uncomment the following line.
# You can also select to disable deprecated APIs only up to a certain version of Qt.
#DEFINES += QT_DISABLE_DEPRECATED_BEFORE=0x060000 # disables all the APIs deprecated before Qt 6.0.0
SOURCES += \
main.cpp \
server.cpp
HEADERS += \
server.h
FORMS += \
server.ui
# Default rules for deployment.
qnx: target.path = /tmp/$${TARGET}/bin
else: unix:!android: target.path = /opt/$${TARGET}/bin
!isEmpty(target.path): INSTALLS += target
二、.h文件
#ifndef SERVER_H
#define SERVER_H
#include <QWidget>
#include <QUdpSocket>
#include <QByteArray>
#include <QHostAddress>
#include <QString>
#include <QDebug>
QT_BEGIN_NAMESPACE
namespace Ui { class Server; }
QT_END_NAMESPACE
class Server : public QWidget
{
Q_OBJECT
public:
Server(QWidget *parent = nullptr);
~Server();
QUdpSocket* serverSocket;
private:
Ui::Server *ui;
};
#endif // SERVER_H
三、.cpp文件
#include "server.h"
#include "ui_server.h"
QString clientIP = "192.xxx.xxx.xxx"; //此处为IP地址
QString clientPort = "8888";
QString serverPort = "9999";
Server::Server(QWidget *parent): QWidget(parent), ui(new Ui::Server)
{
ui->setupUi(this);
ui->lineEdit->setText(clientIP);
ui->lineEdit_2->setText(clientPort);
ui->lineEdit_3->setText(serverPort);
serverSocket = new QUdpSocket(this);
//服务器接收信息
serverSocket->bind(serverPort.toInt()); //绑定服务器接收端口"9999"
connect(serverSocket,&QUdpSocket::readyRead,this,[=](){
qint64 size = serverSocket->pendingDatagramSize();
QByteArray array(size,0);
serverSocket->readDatagram(array.data(),size);
ui->textEdit_2->append(array);
});
//服务器发送信息
connect(ui->pushButton,&QPushButton::clicked,this,[=](){
serverSocket->writeDatagram(ui->textEdit->toPlainText().toUtf8(),QHostAddress(clientIP),clientPort.toInt());
});
}
Server::~Server()
{
delete ui;
}
四、.ui文件
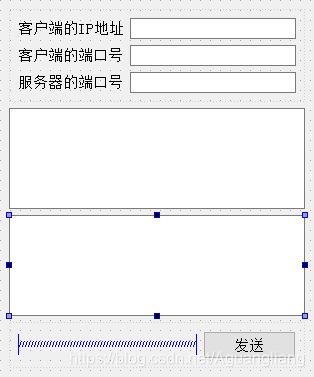