由易到难,循序渐进
A
> A - A 输入两点坐标(X1,Y1),(X2,Y2),计算并输出两点间的距离。
> Input
> 输入数据有多组,每组占一行,由4个实数组成,分别表示x1,y1,x2,y2,数据之间用空格隔开。
> Output
> 对于每组输入数据,输出一行,结果保留两位小数。
> Sample Input
> 0 0 0 1 0 1 1 0
> Sample Output
> 1.00.
> 1.41
// 两点距离
package Vj语法;
import java.util.Scanner;
public class A {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
while(sc.hasNext()) {
double x1 = sc.nextDouble();
double y1 = sc.nextDouble();
double x2 = sc.nextDouble();
double y2 = sc.nextDouble();
double x=x2-x1;
double y=y2-y1;
double d =Math.sqrt(x*x + y*y );
System.out.println(String.format("%.2f",d));
//%.2f是float后的小数只输bai出两位。
}
}
}
B
给定一个日期,输出这个日期是该年的第几天。
Input
输入数据有多组,每组占一行,数据格式为YYYY/MM/DD组成,具体参见sample input ,另外,可以向你确保所有的输入数据是合法的。
Output
对于每组输入数据,输出一行,表示该日期是该年的第几天。
Sample Input
1985/1/20
2006/3/12
Sample Output
20
71
Sponsor
//闰年判断
package Vj语法;
import java.util.Scanner;
public class B {
public static int month[]= {
0,31,28,31,30,31,30,31,31,30,31,30,31};
public static void year(int x) {
if(x % 4 == 0 && x %100 !=0 || (x % 400 == 0))
month[2]=29;
else
month[2]=28;
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
while(sc.hasNext()) {
String s[]=sc.nextLine().split("/");
int ans=0;
year(Integer.valueOf(s[0]));
for (int i = 1; i < Integer.valueOf(s[1]); i++) {
ans+=month[i];
}
System.out.println(ans+Integer.valueOf(s[2]));
}
}
}
C
给定一段连续的整数,求出他们中所有偶数的平方和以及所有奇数的立方和。
Input
输入数据包含多组测试实例,每组测试实例包含一行,由两个整数m和n组成。
Output
对于每组输入数据,输出一行,应包括两个整数x和y,分别表示该段连续的整数中所有偶数的平方和以及所有奇数的立方和。
你可以认为32位整数足以保存结果。
Sample Input
1 3
2 5
Sample Output
4 28
20 152
package Vj语法;
import java.util.Scanner;
public class C {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// TODO Auto-generated method stub
while(sc.hasNext()) {
int m = sc.nextInt();
int n = sc.nextInt();
long x = 0,y=0;
for (int i = Math.min(m, n); i <= Math.max(m, n); i++) {
if (i%2==0) {
x += i*i;
}else {
y+=i*i*i;
};
}
System.out.println(x+" "+y);
}
}
}
D
根据输入的半径值,计算球的体积。
Input
输入数据有多组,每组占一行,每行包括一个实数,表示球的半径。
Output
输出对应的球的体积,对于每组输入数据,输出一行,计算结果保留三位小数。
Sample Input
1
1.5
Sample Output
4.189
14.137
Hint
#define PI 3.1415927
package Vj语法;
import java.util.Scanner;
public class D {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
while(sc.hasNext()) {
double r = sc.nextDouble();
double s = 3.1415927*4/3*r*r*r;
System.out.println(String.format("%.3f",s));
}
}
}
E
输入三个字符后,按各字符的ASCII码从小到大的顺序输出这三个字符。
Input
输入数据有多组,每组占一行,有三个字符组成,之间无空格。
Output
对于每组输入数据,输出一行,字符中间用一个空格分开。
Sample Input
qwe
asd
zxc
Sample Output
e q w
a d s
c x z
package Vj语法;
import java.util.Arrays;
import java.util.Scanner;
public class E {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in); // TODO Auto-generated method stub
while (sc.hasNext()) {
char c[] = sc.next().toCharArray();
Arrays.sort(c);
for (int i = 0; i < 3; i++) {
if(i==2)
System.out.println(c[i]);
else
System.out.print(c[i]+" ");
}
}
}
}
F
数列的定义如下:
数列的第一项为n,以后各项为前一项的平方根,求数列的前m项的和。
Input
输入数据有多组,每组占一行,由两个整数n(n<10000)和m(m<1000)组成,n和m的含义如前所述。
Output
对于每组输入数据,输出该数列的和,每个测试实例占一行,要求精度保留2位小数。
Sample Input
81 4
2 2
Sample Output
94.73
3.41
package Vj语法;
import java.util.Scanner;
import java.lang.Math;
public class F {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
while (sc.hasNext()) {
double n = sc.nextDouble();
int m = sc.nextInt();
double arr[] = new double[m];
arr[0]=n;
double sum = n;
for (int i = 1; i <m; i++) {
arr[i] = Math.sqrt(arr[i-1]);
sum+=arr[i];
}
System.out.println(String.format("%.2f",sum));
}
}
}
G
统计每个元音字母在字符串中出现的次数。
Input
输入数据首先包括一个整数n,表示测试实例的个数,然后是n行长度不超过100的字符串。
Output
对于每个测试实例输出5行,格式如下:
a:num1
e:num2
i:num3
o:num4
u:num5
多个测试实例之间由一个空行隔开。
请特别注意:最后一块输出后面没有空行:)
Sample Input
2
aeiou
my name is ignatius
Sample Output
a:1
e:1
i:1
o:1
u:1
a:2
e:1
i:3
o:0
u:1
//统计每个元音字母在字符串中出现的次数
package Vj语法;
import java.util.Scanner;
public class G {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
sc.nextLine();
for (int t = 0; t < n; t++) {
String s = sc.nextLine();
int arr[] = new int[5];
char c[] = s.toCharArray();
for (int i = 0; i < s.length(); i++) {
if(c[i]=='a') {
arr[0]++;
}else if (c[i]=='e') {
arr[1]++;
}else if (c[i]=='i') {
arr[2]++;
}else if (c[i]=='o') {
arr[3]++;
}else if (c[i]=='u') {
arr[4]++;
}
}
System.out.println("a:"+arr[0]);
System.out.println("e:"+arr[1]);
System.out.println("i:"+arr[2]);
System.out.println("o:"+arr[3]);
System.out.println("u:"+arr[4]);
if(t!=n-1)
System.out.println();
}
}
}
H
多项式的描述如下:
1 - 1/2 + 1/3 - 1/4 + 1/5 - 1/6 + ...
现在请你求出该多项式的前n项的和。
Input
输入数据由2行组成,首先是一个正整数m(m<100),表示测试实例的个数,第二行包含m个正整数,对于每一个整数(不妨设为n,n<1000),求该多项式的前n项的和。
Output
对于每个测试实例n,要求输出多项式前n项的和。每个测试实例的输出占一行,结果保留2位小数。
Sample Input
2
1 2
Sample Output
1.00
0.50
package Vj语法;
import java.util.Scanner;
public class H {
public static double arr[]= new double[1001];
public static void Init() {
for (int i = 1; i < 1001; i++) {
arr[i]=1.0/i;
}
for (int i = 1; i < 1001; i++) {
if (i%2==0) {
arr[i]=arr[i-1]-arr[i];
}else {
arr[i]=arr[i-1]+arr[i];
}
}
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
int m=sc.nextInt();
Init();
for (int t=0;t<m;t++) {
int n =sc.nextInt();
System.out.println(String.format("%.2f", arr[n]));
}
}
}
I
“回文串”是一个正读和反读都一样的字符串,比如“level”或者“noon”等等就是回文串。请写一个程序判断读入的字符串是否是“回文”。
Input
输入包含多个测试实例,输入数据的第一行是一个正整数n,表示测试实例的个数,后面紧跟着是n个字符串。
Output
如果一个字符串是回文串,则输出"yes",否则输出"no".
Sample Input
4
level
abcde
noon
haha
Sample Output
yes
no
yes
no
// - 回文字符串
//“回文串”是一个正读和反读都一样的字符串,比如“level”或者“noon”等等就是回文串。请写一个程序判断读入的字符串是否是“回文”。
package Vj语法;
import java.util.Scanner;
public class I {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc =new Scanner(System.in);
int n =sc.nextInt();
for (int t = 0; t < n; t++) {
char c[] =sc.next().toCharArray();
for (int i = 0; i < c.length; i++) {
if(c[i]!=c[c.length-1-i]) {
System.out.println("no");
break;
}else if (i>c.length-1-i) {
System.out.println("yes");
break;
}
}
}
}
}
J
对于表达式n^2+n+41,当n在(x,y)范围内取整数值时(包括x,y)(-39<=x<y<=50),判定该表达式的值是否都为素数。
Input
输入数据有多组,每组占一行,由两个整数x,y组成,当x=0,y=0时,表示输入结束,该行不做处理。
Output
对于每个给定范围内的取值,如果表达式的值都为素数,则输出"OK",否则请输出“Sorry”,每组输出占一行。
Sample Input
0 1
0 0
Sample Output
OK
package Vj语法;
import java.util.Scanner;
public class J {
public static boolean arr[]=new boolean[3000];
public static void Init() {
for (int i = 0; i < 3000; i++)arr[i]=true;
for (int i = 2; i < 3000; i++) {
for (int j = i*2; j < 3000; j+=i) {
arr[j] = false;
}
}
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
Init();
while (sc.hasNext()) {
int x = sc.nextInt(),y=sc.nextInt();
if(x==0&&y==0)break;
boolean All = true;
for (int i = x; i <=y; i++) {
if(!arr[i*i+i+41])All = false;
}
System.out.println(All?"OK":"Sorry");
}
}
}
K
扫描二维码关注公众号,回复:
13135842 查看本文章
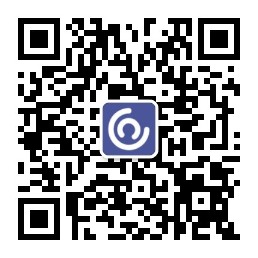
喜欢西游记的同学肯定都知道悟空偷吃蟠桃的故事,你们一定都觉得这猴子太闹腾了,其实你们是有所不知:悟空是在研究一个数学问题!
什么问题?他研究的问题是蟠桃一共有多少个!
不过,到最后,他还是没能解决这个难题,呵呵^-^
当时的情况是这样的:
第一天悟空吃掉桃子总数一半多一个,第二天又将剩下的桃子吃掉一半多一个,以后每天吃掉前一天剩下的一半多一个,到第n天准备吃的时候只剩下一个桃子。聪明的你,请帮悟空算一下,他第一天开始吃的时候桃子一共有多少个呢?
Input
输入数据有多组,每组占一行,包含一个正整数n(1<n<30),表示只剩下一个桃子的时候是在第n天发生的。
Output
对于每组输入数据,输出第一天开始吃的时候桃子的总数,每个测试实例占一行。
Sample Input
2
4
Sample Output
4
22
package Vj语法;
import java.util.Scanner;
public class K {
public static long arr[]=new long [32];
public static void Init() {
arr[1]=1;
for (int i = 2; i < 32; i++) {
arr[i]= (arr[i-1]+1)*2;
}
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
Init();
while (sc.hasNext()) {
int n = sc.nextInt();
System.out.println(arr[n]);
}
}
}
L
如果三条边长A,B,C能组成三角形的话,输出YES,否则NO。
Sample Input
2给定三条边,请你判断一下能不能组成一个三角形。
Input
输入数据第一行包含一个数M,接下有M行,每行一个实例,包含三个正数A,B,C。其中A,B,C <1000;
Output
对于每个测试实例,
1 2 3
2 2 2
Sample Output
NO
YES
Sponsor
package Vj语法;
import java.util.Arrays;
import java.util.Scanner;
public class L {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
int M = sc.nextInt();
double arr[] = new double[3];
for (int i = 0; i < M; i++) {
for (int j = 0; j < 3; j++) {
arr[j] = sc.nextDouble();
}
Arrays.sort(arr);
System.out.println((arr[0]+arr[1]>arr[2])?"YES":"NO");
}
}
}
M
青年歌手大奖赛中,评委会给参赛选手打分。选手得分规则为去掉一个最高分和一个最低分,然后计算平均得分,请编程输出某选手的得分。
Input
输入数据有多组,每组占一行,每行的第一个数是n(2<n<=100),表示评委的人数,然后是n个评委的打分。
Output
对于每组输入数据,输出选手的得分,结果保留2位小数,每组输出占一行。
Sample Input
3 99 98 97
4 100 99 98 97
Sample Output
98.00
98.50
package Vj语法;
import java.util.Arrays;
import java.util.Scanner;
public class M {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
while (sc.hasNext()) {
int n = sc.nextInt();
double arr[] = new double[n];
for (int i = 0; i < n; i++) {
arr[i] = sc.nextDouble();
}
Arrays.sort(arr);
double sum = 0;
for (int i = 1; i < n-1; i++) {
sum+=arr[i];
}
System.out.println(String.format("%.2f",sum/(n-2)));
}
}
}
N
还记得中学时候学过的杨辉三角吗?具体的定义这里不再描述,你可以参考以下的图形:
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
1 5 10 10 5 1
Input
输入数据包含多个测试实例,每个测试实例的输入只包含一个正整数n(1<=n<=30),表示将要输出的杨辉三角的层数。
Output
对应于每一个输入,请输出相应层数的杨辉三角,每一层的整数之间用一个空格隔开,每一个杨辉三角后面加一个空行。
Sample Input
2 3
Sample Output
1
1 1
1
1 1
1 2 1
package Vj语法;
import java.util.Scanner;
public class N {
public static long arr[][] =new long[32][32];
public static void Init() {
arr[1][1]=1;
for (int i = 2; i < 32; i++) {
for(int j=1;j < 32;j++) {
arr[i][j]= arr[i-1][j-1] + arr[i-1][j];
}
}
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
Init();
while(sc.hasNext()) {
int n = sc.nextInt();
for (int i = 1; i <=n; i++) {
for (int j = 1; j <=n; j++) {
if(arr[i][j]==0) {
continue;
}else {
System.out.print(arr[i][j]+(i==j?"":" "));
}
}
System.out.println();
}
System.out.println();
}
}
}
O
求n个数的最小公倍数。
Input
输入包含多个测试实例,每个测试实例的开始是一个正整数n,然后是n个正整数。
Output
为每组测试数据输出它们的最小公倍数,每个测试实例的输出占一行。你可以假设最后的输出是一个32位的整数。
Sample Input
2 4 6
3 2 5 7
Sample Output
12
70
package Vj语法;
import java.util.Scanner;
public class O {
public static long gcd(long x,long y) {
if (y==0) return x;
else return gcd(y,x%y);
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
while (sc.hasNext()) {
int n = sc.nextInt();
long ans =1;
for (int i = 0; i < n; i++) {
long x=sc.nextLong();
ans = ans*x/gcd(ans,x);
}
System.out.println(ans);
}
}
}
P
古希腊数学家毕达哥拉斯在自然数研究中发现,220的所有真约数(即不是自身的约数)之和为:
1+2+4+5+10+11+20+22+44+55+110=284。
而284的所有真约数为1、2、4、71、 142,加起来恰好为220。人们对这样的数感到很惊奇,并称之为亲和数。一般地讲,如果两个数中任何一个数都是另一个数的真约数之和,则这两个数就是亲和数。
你的任务就编写一个程序,判断给定的两个数是否是亲和数
Input
输入数据第一行包含一个数M,接下有M行,每行一个实例,包含两个整数A,B; 其中 0 <= A,B <= 600000 ;
Output
对于每个测试实例,如果A和B是亲和数的话输出YES,否则输出NO。
Sample Input
2
220 284
100 200
Sample Output
YES
NO
package Vj语法;
import java.util.HashSet;
import java.util.Scanner;
public class P {
public static int check(int x) {
HashSet<Integer> set= new HashSet<>();
set.add(1);
for (int j = 2; j < Math.sqrt(x); j++) {
if (x%j==0) {
set.add(j);
set.add(x/j);
}
}
int ans = 0;
for (int i : set) {
ans+=i;
}
return ans;
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
int m = sc.nextInt();
for (int i = 0; i < m; i++) {
int a = sc.nextInt();
int b = sc.nextInt();
if (check(a)==b&&check(b)==a) {
System.out.println("Yes");
}else {
System.out.println("No");
}
}
}
}
Q
你活的不容易,我活的不容易,他活的也不容易。不过,如果你看了下面的故事,就会知道,有位老汉比你还不容易。
重庆市郊黄泥板村的徐老汉(大号徐东海,简称XDH)这两年辛辛苦苦养了不少羊,到了今年夏天,由于众所周知的高温干旱,实在没办法解决牲畜的饮水问题,就决定把这些羊都赶到集市去卖。从黄泥板村到交易地点要经过N个收费站,按说这收费站和徐老汉没什么关系,但是事实却令徐老汉欲哭无泪:
(镜头回放)
近景:老汉,一群羊
远景:公路,收费站
......
收费员(彬彬有礼+职业微笑):“老同志,请交过路费!”
徐老汉(愕然,反应迟钝状):“锅,锅,锅,锅-炉-费?我家不烧锅炉呀?”
收费员(职业微笑依然):“老同志,我说的是过-路-费,就是你的羊要过这个路口必须交费,understand?”
徐老汉(近镜头10秒,嘴巴张开):“我-我-我知道汽车过路要收费,这羊也要收费呀?”
收费员(居高临下+不解状):“老同志,你怎么就不明白呢,那么我问你,汽车几个轮子?”
徐老汉(稍放松):“这个我知道,今天在家里我孙子还问我这个问题,4个!”
收费员(生气,站起):“嘿!老头,你还骂人不带脏字,既然知道汽车四个轮子,难道就不知道这羊有几条腿吗?!”
徐老汉(尴尬,依然不解状):“也,也,也是4个呀,这有关系吗?”
收费员(生气,站起):“怎么没关系!我们头说了,只要是4条腿的都要收费!”
......
(画外音)
由于徐老汉没钱,收费员就将他的羊拿走一半,看到老汉泪水涟涟,犹豫了一下,又还给老汉一只。巧合的是,后面每过一个收费站,都是拿走当时羊的一半,然后退还一只,等到老汉到达市场,就只剩下3只羊了。
你,当代有良知的青年,能帮忙算一下老汉最初有多少只羊吗?
Input
输入数据第一行是一个整数N,下面由N行组成,每行包含一个整数a(0<a<=30),表示收费站的数量。
Output
对于每个测试实例,请输出最初的羊的数量,每个测试实例的输出占一行。
Sample Input
2
1
2
Sample Output
4
6
Sponsor
package Vj语法;
import java.util.Scanner;
public class Q {
public static int arr[]= new int[32];
public static void name() {
arr[0]=3;
for (int j = 1; j < 32; j++) {
arr[j] = (arr[j-1]-1)*2;
}
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
name();
for (int i = 0; i < n; i++) {
int a = sc.nextInt();
System.out.println(arr[a]);
}
}
}
R
有一个长度为n(n<=100)的数列,该数列定义为从2开始的递增有序偶数,现在要求你按照顺序每m个数求出一个平均值,如果最后不足m个,则以实际数量求平均值。编程输出该平均值序列。
Input
输入数据有多组,每组占一行,包含两个正整数n和m,n和m的含义如上所述。
Output
对于每组输入数据,输出一个平均值序列,每组输出占一行。
Sample Input
3 2
4 2
Sample Output
3 6
3 7
package Vj语法;
import java.util.Scanner;
public class R {
public static int arr[]= new int[102];
public static void name() {
for (int j = 0; j < arr.length; j++) {
arr[j]=j*2;
}
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc =new Scanner(System.in);
name();
while (sc.hasNext()) {
int n = sc.nextInt();
int m = sc.nextInt();
int sum = 0;
for (int i = 1; i < n; i++) {
sum+=arr[i];
if (i%m==0) {
System.out.print(sum/m+" ");
sum=0;
}
}
sum+=arr[n];
if (n%m==0) {
System.out.println(sum/m);
}else {
System.out.println(sum/(n%m));
}
}
}
}
S
输入n(n<100)个数,找出其中最小的数,将它与最前面的数交换后输出这些数。
Input
输入数据有多组,每组占一行,每行的开始是一个整数n,表示这个测试实例的数值的个数,跟着就是n个整数。n=0表示输入的结束,不做处理。
Output
对于每组输入数据,输出交换后的数列,每组输出占一行。
Sample Input
4 2 1 3 4
5 5 4 3 2 1
0
Sample Output
1 2 3 4
1 4 3 2 5
package Vj语法;
import java.util.Scanner;
public class S {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
while (sc.hasNext()) {
int n = sc.nextInt();
if(n==0)break;
int arr[]= new int[n];
int min =Integer.MAX_VALUE;
int index=0;
for (int i = 0; i < n; i++) {
arr[i] = sc.nextInt();
if (arr[i]<min) {
min = arr[i];
index = i;
}
}
int t=arr[index];arr[index]=arr[0];arr[0]=t;
for (int i = 0; i < n-1; i++) {
System.out.print(arr[i]+" ");
}
System.out.println(arr[n-1]);
}
}
}
T
对于给定的一个字符串,统计其中数字字符出现的次数。
Input
输入数据有多行,第一行是一个整数n,表示测试实例的个数,后面跟着n行,每行包括一个由字母和数字组成的字符串。
Output
对于每个测试实例,输出该串中数值的个数,每个输出占一行。
Sample Input
2
asdfasdf123123asdfasdf
asdf111111111asdfasdfasdf
Sample Output
6
9
Sponsor
package Vj语法;
import java.util.Scanner;
public class T {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
sc.nextLine();
for (int t = 0; t < n; t++) {
String s = sc.nextLine();
char c[] = s.toCharArray();
int sum = 0;
for (int i = 0; i < c.length; i++) {
if (c[i]-'0'>=0&&c[i]-'0'<=9) {
sum++;
}
}
System.out.println(sum);
}
}
}
U
有一头母牛,它每年年初生一头小母牛。每头小母牛从第四个年头开始,每年年初也生一头小母牛。请编程实现在第n年的时候,共有多少头母牛?
Input
输入数据由多个测试实例组成,每个测试实例占一行,包括一个整数n(0<n<55),n的含义如题目中描述。
n=0表示输入数据的结束,不做处理。
Output
对于每个测试实例,输出在第n年的时候母牛的数量。
每个输出占一行。
Sample Input
2
4
5
0
Sample Output
2
4
6
Sponsor
package Vj语法;
import java.util.Scanner;
public class U {
public static long arr[] = new long [56];
public static void name() {
arr[1]=1;
arr[2]=2;
arr[3]=3;
arr[4]=4;
for (int i = 5; i < 56; i++) {
arr[i] = arr[i-1]+arr[i-3];
}
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
name();
while (sc.hasNext()) {
int n = sc.nextInt();
if(n==0)break;
System.out.println(arr[n]);
}
}
}
V
对于输入的每个字符串,查找其中的最大字母,在该字母后面插入字符串“(max)”。
Input
输入数据包括多个测试实例,每个实例由一行长度不超过100的字符串组成,字符串仅由大小写字母构成。
Output
对于每个测试实例输出一行字符串,输出的结果是插入字符串“(max)”后的结果,如果存在多个最大的字母,就在每一个最大字母后面都插入"(max)"。
Sample Input
abcdefgfedcba
xxxxx
Sample Output
abcdefg(max)fedcba
x(max)x(max)x(max)x(max)x(max)
package Vj语法;
import java.util.Scanner;
public class V {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
while (sc.hasNext()) {
String s = sc.next();
char c[] = s.toCharArray();
char maxs= 'A';
for (int i = 0; i < c.length; i++) {
if(maxs<c[i]) {
maxs=c[i];
}
}
StringBuilder sd= new StringBuilder();
for (int i = 0; i < c.length; i++) {
sd.append(c[i]);
if (maxs==c[i]) {
sd.append("(max)");
}
}
System.out.println(sd);
}
}
}
W
输入n(n<=100)个整数,按照绝对值从大到小排序后输出。题目保证对于每一个测试实例,所有的数的绝对值都不相等。
Input
输入数据有多组,每组占一行,每行的第一个数字为n,接着是n个整数,n=0表示输入数据的结束,不做处理。
Output
对于每个测试实例,输出排序后的结果,两个数之间用一个空格隔开。每个测试实例占一行。
Sample Input
3 3 -4 2
4 0 1 2 -3
0
Sample Output
-4 3 2
-3 2 1 0
package Vj语法;
import java.util.Scanner;
public class W {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
while (sc.hasNext()) {
int n = sc.nextInt();
if(n==0)break;
int arr[]=new int[n];
for (int i = 0; i < n; i++) {
arr[i] = sc.nextInt();
}
for (int i = 0; i < n; i++) {
for (int j = 0; j < n-i-1; j++) {
if(Math.abs(arr[j])<Math.abs(arr[j+1])) {
int t= arr[j];
arr[j] = arr[j+1];
arr[j+1] = t;
}
}
}
StringBuilder ans = new StringBuilder();
for (int i = 0; i < n; i++) {
ans.append(arr[i]+(i==n-1?"":" "));
}
System.out.println(ans);
}
}
}
X
作为杭电的老师,最盼望的日子就是每月的8号了,因为这一天是发工资的日子,养家糊口就靠它了,呵呵
但是对于学校财务处的工作人员来说,这一天则是很忙碌的一天,财务处的小胡老师最近就在考虑一个问题:如果每个老师的工资额都知道,最少需要准备多少张人民币,才能在给每位老师发工资的时候都不用老师找零呢?
这里假设老师的工资都是正整数,单位元,人民币一共有100元、50元、10元、5元、2元和1元六种。
Input
输入数据包含多个测试实例,每个测试实例的第一行是一个整数n(n<100),表示老师的人数,然后是n个老师的工资。
n=0表示输入的结束,不做处理。
Output
对于每个测试实例输出一个整数x,表示至少需要准备的人民币张数。每个输出占一行。
Sample Input
3
1 2 3
0
Sample Output
4
package Vj语法;
import java.util.Scanner;
public class X {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
while (sc.hasNext()) {
int n = sc.nextInt();
if (n==0) break;
int sum=0;
for (int i = 0; i < n; i++) {
int x = sc.nextInt();
sum+=x/100;
x=x%100;
sum+=x/50;
x=x%50;
sum+=x/10;
x=x%10;
sum+=x/5;
x=x%5;
sum+=x/2;
x=x%2;
sum+=x;
}
System.out.println(sum);
}
}
}
Y
potato老师虽然很喜欢教书,但是迫于生活压力,不得不想办法在业余时间挣点外快以养家糊口。
“做什么比较挣钱呢?筛沙子没力气,看大门又不够帅...”potato老师很是无奈。
“张艺谋比你还难看,现在多有钱呀,听说还要导演奥运开幕式呢!你为什么不去娱乐圈发展呢?”lwg在一旁出主意。
嗯,也是,为了生存,就委屈点到娱乐圈混混吧,马上就拍一部激光电影《杭电记忆——回来我的爱》。
说干就干,马上海选女主角(和老谋子学的,此举可以吸引媒体的眼球,呵呵),并且特别规定,演员必须具有ac的基本功,否则直接out!
由于策划师风之鱼(大师级水王)宣传到位,来应聘的MM很多,当然包括nit的蛋糕妹妹等呼声很高的美女,就连zjut的jqw都男扮女装来应聘(还好被安全顾问hdu_Bin-Laden认出,给轰走了),看来娱乐圈比acm还吸引人哪...
面试那天,刚好来了m*n个MM,站成一个m*n的队列,副导演Fe(OH)2为每个MM打了分数,分数都是32位有符号整数。
一开始我很纳闷:分数怎么还有负的?Fe(OH)2解释说,根据选拔规则,头发染成黄色、化妆太浓、穿的太少等等都要扣分数的,扣的多了就可能是负分了,当然,如果发现话语中夹有日语,就直接给-2147483648分了。
分数送上来了,是我做决定的时候了,我的一个选拔原则是,要选一个面试分数绝对值(必须还是32位整数)最大的MM。
特别说明:如果不幸选中一个负分的MM,也没关系,因为我觉得,如果不能吸引你,那要想法恶心你。
Input
输入数据有多组,每组的第一行是两个整数m和n,表示应聘MM的总共的行列数,然后是m行整数,每行有n个,m和n的定义见题目的描述。
Output
对于每组输入数据,输出三个整数x,y和s,分别表示选中的MM的行号、列号和分数。
note:行号和列号从一开始,如果有多个MM的分数绝对值一样,那么输出排在最前面的一个(即行号最小的那个,如果行号相同则取列号最小的那个)。
Sample Input
2 3
1 4 -3
-7 3 0
Sample Output
2 1 -7
package Vj语法;
import java.util.Scanner;
public class Y {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
while (sc.hasNext()) {
int m = sc.nextInt();
int n = sc.nextInt();
long arr[][] = new long[m][n]; // 定义long,否则转绝对值爆int
long x=0,y=0,value=0;
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
long c = sc.nextInt();
if (Math.abs(c)>Math.abs(value)) {
value = c;
x = i;
y = j;
}
}
}
System.out.println((x+1)+" "+(y+1)+" "+value);
}
}
}
Z
假设一个班有n(n<=50)个学生,每人考m(m<=5)门课,求每个学生的平均成绩和每门课的平均成绩,并输出各科成绩均大于等于平均成绩的学生数量。
Input
输入数据有多个测试实例,每个测试实例的第一行包括两个整数n和m,分别表示学生数和课程数。然后是n行数据,每行包括m个整数(即:考试分数)。
Output
对于每个测试实例,输出3行数据,第一行包含n个数据,表示n个学生的平均成绩,结果保留两位小数;第二行包含m个数据,表示m门课的平均成绩,结果保留两位小数;第三行是一个整数,表示该班级中各科成绩均大于等于平均成绩的学生数量。
每个测试实例后面跟一个空行。
Sample Input
2 2
5 10
10 20
Sample Output
7.50 15.00
7.50 15.00
1
package Vj语法;
import java.util.Scanner;
public class Z {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner sc = new Scanner(System.in);
while (sc.hasNext()) {
int n = sc.nextInt();
int m = sc.nextInt();
int count = 0;
double arr[][] = new double[n+1][m+1];
for (int i = 1; i <=n; i++) {
for (int j = 1; j <= m; j++) {
arr[i][j] = sc.nextDouble();
arr[i][0]+=arr[i][j];
arr[0][j]+=arr[i][j];
}
arr[i][0]/=m; // 学生平均分
}
for (int i = 1; i <=m; i++) {
arr[0][i]/=n; // 科目平均分
}
for (int i = 1; i <=n; i++) {
for (int j = 1; j <=m; j++) {
if(arr[i][j]<arr[0][j])break;
if(arr[i][j]>=arr[0][j]&&j==m) {
count++;
}
}
}
for (int i = 1; i <=n; i++) {
System.out.print(String.format("%.2f",arr[i][0])+(i==n?"":" "));
}
System.out.println();
for (int i = 1; i <=m; i++) {
System.out.print(String.format("%.2f",arr[0][i])+(i==m?"":" "));
}
System.out.println();
System.out.println(count);
System.out.println();
}
}
}
项目场景:
提示:这里简述项目相关背景:
例如:项目场景:示例:通过蓝牙芯片(HC-05)与手机 APP 通信,每隔 5s 传输一批传感器数据(不是很大)
问题描述:
提示:这里描述项目中遇到的问题:
例如:数据传输过程中数据不时出现丢失的情况,偶尔会丢失一部分数据
APP 中接收数据代码:
@Override
public void run() {
bytes = mmInStream.read(buffer);
mHandler.obtainMessage(READ_DATA, bytes, -1, buffer).sendToTarget();
}