1. 题目
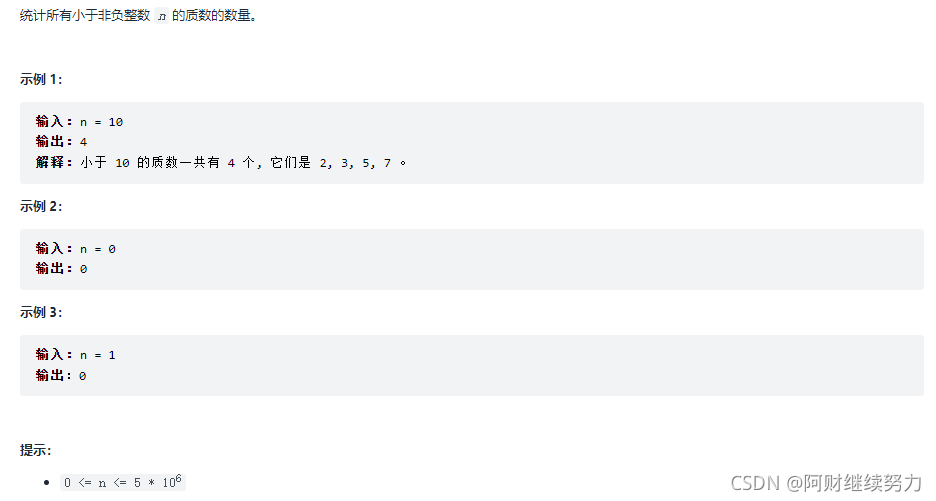
2. 思路
(1) ArrayList
- 将找到的质数存入集合中,若一个数不能被它之前的任何质数整除,则表示这个数是一个质数。
(2) 埃氏筛
- 创建一个数组用于标记质数,初始时假设所有的数都是质数,对于一个质数,其任何倍数必然是非质数,因此每当找到一个质数,将其所有倍数均标记为非质数。
- 当找到某个质数x时,其x*x之前的倍数必然被其他质数标记为非质数,因此可以从x*x开始标记。
3. 代码
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class Test {
public static void main(String[] args) {
Solution solution = new Solution();
System.out.println(solution.countPrimes(5));
}
}
class Solution {
public int countPrimes(int n) {
if (n == 0 || n == 1) {
return 0;
}
List<Integer> primes = new ArrayList<>();
int index;
for (int i = 2; i < n; i++) {
index = 0;
while (index < primes.size()) {
if (i % primes.get(index++) == 0) {
break;
}
}
if (index == primes.size()) {
primes.add(i);
}
}
return primes.size();
}
}
class Solution1 {
public int countPrimes(int n) {
int[] nonPrime = new int[n];
int res = 0;
for (int i = 2; i < n; i++) {
if (nonPrime[i] == 0) {
res++;
if ((long) i * i < n) {
for (int j = i * i; j < n; j += i) {
nonPrime[j] = 1;
}
}
}
}
return res;
}
}