小组分工
任务 | 姓名 |
---|---|
面向对象设计、功能设计、DAO模式设计、博客制作 | 郭宇豪 |
前期调查、编码规范、主菜单编写 | 黄常奇 |
1.前期调查
京东商城中的购物车
购物车最主要的功能为商品条目显示、商品小计、商品数量累加、商品单价显示、商品总价,附加功能为用户登录和结账。
编码规范
代码中的命名严禁使用拼音与英文混合的方式,更不允许直接使用中文的方式。说明:正确的英文拼写和语法可以让阅读者易于理解,避免歧义。注意,即使纯拼音命名方式也要避免采用。
正例:alibaba / taobao / youku / hangzhou 等国际通用的名称,可视同英文。
反例:DaZhePromotion [打折] / getPingfenByName() [评分] / int 某变量 = 3
类名使用 UpperCamelCase 风格,必须遵从驼峰形式,但以下情形例外:DO / BO /DTO / VO / AO
正例:MarcoPolo / UserDO / XmlService / TcpUdpDeal / TaPromotion
反例:macroPolo / UserDo / XMLService / TCPUDPDeal / TAPromotion
方法名、参数名、成员变量、局部变量都统一使用 lowerCamelCase 风格,必须遵从驼峰形式。
正例: localValue / getHttpMessage() / inputUserId
包名统一使用小写,点分隔符之间有且仅有一个自然语义的英语单词。包名统一使用单数形式,但是类名如果有复数含义,类名可以使用复数形式。
正例: 应用工具类包名为 com.alibaba.open.util、类名为 essageUtils(此规则参考spring 的框架结构)
各层命名规约:
A) Service/DAO 层方法命名规约
1) 获取单个对象的方法用 get 做前缀。
2) 获取多个对象的方法用 list 做前缀。
3) 获取统计值的方法用 count 做前缀。
4) 插入的方法用 save/insert 做前缀。
5) 删除的方法用 remove/delete 做前缀。
6) 修改的方法用 update 做前缀。
B) 领域模型命名规约
1) 数据对象:xxxDO,xxx 即为数据表名。
2) 数据传输对象:xxxDTO,xxx 为业务领域相关的名称。
3) 展示对象:xxxVO,xxx 一般为网页名称。
4) POJO 是 DO/DTO/BO/VO 的统称,禁止命名成 xxxPOJO。
2.系统功能结构图
3.系统描述
系统运行时应先进入用户登录模块输入账号密码用以登录 ,登录成功后展现功能列表,功能1展示商城中商品编号、名称和单价,功能为展示购物车内商品的编号、名称、数量、小计和总价,功能3、4为向购物车内增添商品,从购物车里删减商品(搜寻方式可为编号或者名称)(实现时都将对购物车内商品进行小计和购物车内商品总价的计算),功能5为清空购物车,功能6实现对话框形式的结账功能,功能7实现退出购物车功能。
4.UML类图
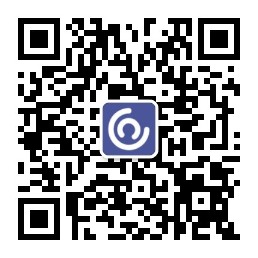
5.封装性体现
商品的信息存储在文件中,在操作中无法更改。
购物车属性在操作时以private数组形式存在,仅能用setter/getter来进行存取数据。操作完毕以后更新完的购物车信息会存入文件中,用户无法随意方法。
DAO模式的设计让使用者无需知道储存方式和具体实现,实现封装保证安全的同时又便于操作。
6.DAO模式设计
接口类分三类——商品、购物车、用户
实体类仅商品类,既能实现功能又能避免冗余
实现类有商品、购物车、用户三类
7.项目包结构与代码
CartDao类
package shoppingcart;
import java.io.FileNotFoundException;
import java.io.IOException;
public interface CartDao {
public void showCart()throws IOException;
//展示购物车内容
public void addCart(int i,int nuin) throws FileNotFoundException, IOException;
//执行添加功能,并返回是否成功
public boolean deleteCart(int i,int nuin) throws FileNotFoundException, IOException;
//执行删减功能,并返回是否成功
public void clearCart() throws FileNotFoundException;
//执行清空购物车功能
}
CartDaoImpl类
package shoppingcart;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.IOException;
import java.io.PrintStream;
public class CartDaoImpl implements CartDao {
Goods goods=new Goods();
@Override
public void showCart()throws IOException{
System.out.println("编号: 商品名称: 数量: 小计: ");
FileReader fr = new FileReader("D:\\大二上\\JAVA\\JAVA项目\\ShoppingCart(1.3-DAO)\\src\\shoppingcart\\data\\Cart.txt");
BufferedReader br = new BufferedReader(fr);
while(br.ready()){
System.out.println(br.readLine());
}
System.out.println("总计:"+goods.total+"元");
}//输出购物车内容
@Override
public void addCart(int i,int nuin) throws IOException {
goods.getQuantity()[i]+=nuin;
goods.getAccount()[i]+=goods.getPrice()[i]*nuin;
goods.total+=goods.getAccount()[i];
File file = new File("D:\\大二上\\JAVA\\JAVA项目\\ShoppingCart(1.3-DAO)\\src\\shoppingcart\\data\\Cart.txt");
PrintStream ps = new PrintStream(new FileOutputStream(file));
ps.println("");// 清空文件内容
FileOutputStream fos = new FileOutputStream ("D:\\大二上\\JAVA\\JAVA项目\\ShoppingCart(1.3-DAO)\\src\\shoppingcart\\data\\Cart.txt",true ) ;
String str="";
for(int index=0;index<4;index++) {
if(goods.getQuantity()[index]!=0) {
str = goods.getNumber()[index]+" "+goods.getName()[index]+" "+goods.getQuantity()[index]+" "+goods.getAccount()[index]+"\t\n";
fos.write(str.getBytes()) ;
}
}
fos.close ();
}//添加商品入购物车
@Override
public boolean deleteCart(int i,int nuin) throws IOException {
if(goods.getQuantity()[i]-nuin>=0) {
goods.getQuantity()[i]-=nuin;
goods.getAccount()[i]-=goods.getPrice()[i]*nuin;
goods.total-=goods.getPrice()[i]*nuin;
File file = new File("D:\\大二上\\JAVA\\JAVA项目\\ShoppingCart(1.3-DAO)\\src\\shoppingcart\\data\\Cart.txt");
PrintStream ps = new PrintStream(new FileOutputStream(file));
ps.println("");
FileOutputStream fos = new FileOutputStream ("D:\\大二上\\JAVA\\JAVA项目\\ShoppingCart(1.3-DAO)\\src\\shoppingcart\\data\\Cart.txt",true ) ;
String str="";
for(int index=0;index<4;index++) {
if(goods.getQuantity()[index]!=0) {
str = goods.getNumber()[index]+" "+goods.getName()[index]+" "+goods.getQuantity()[index]+" "+goods.getAccount()[index]+"\t\n";
fos.write(str.getBytes()) ;
}
}
fos.close ();
return true;
}
else return false;
}//从购物车中删除商品
@Override
public void clearCart() throws FileNotFoundException {
File file = new File("D:\\大二上\\JAVA\\JAVA项目\\ShoppingCart(1.3-DAO)\\src\\shoppingcart\\data\\Cart.txt");
PrintStream ps = new PrintStream(new FileOutputStream(file));
ps.println("");
}//清空购物车
}
GoodsDao类
package shoppingcart;
import java.io.IOException;
public interface GoodsDao {
public void showGoods()throws IOException;
//展示商城商品条目
public int searchNumber(int nu);
//判断商城是否有这件商品(通过编号)
public int searchName(String na);
//判断商城是否有这件商品(通过名称)
}
GoodsDaoImpl类
package shoppingcart;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class GoodsDaoImpl implements GoodsDao{
Goods goods=new Goods();
@Override
public void showGoods()throws IOException{
FileReader fr = new FileReader("D:\\大二上\\JAVA\\JAVA项目\\ShoppingCart(1.3-DAO)\\src\\shoppingcart\\data\\Goods.txt");
BufferedReader br = new BufferedReader(fr);
while(br.ready()){
System.out.println(br.readLine());
}//输出商品列表
}
@Override
public int searchNumber(int nu) {
int i=5;
for(i=0;i<4;i++) {
if(nu==goods.getNumber()[i]) {
break;
}}
return i;
}
@Override
public int searchName(String na) {
int i=5;
for(i=0;i<4;i++) {
if(na.equals(goods.getName()[i])) {
break;
}}
return i;
}
}
OrederDao类
package shoppingcart;
import java.io.IOException;
public interface OrderDao {
//allfuntions
public boolean accountNumber(String ia)throws Exception ;
//判断用户账号是否输入正确
public boolean passwordNumber(String ip) throws Exception ;
//判断用户密码是否输入正确
public void pickUp() throws IOException;
//结算
}
OrderDaoImpl类
package shoppingcart;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import javax.swing.JOptionPane;
public class OrderDaoImpl implements OrderDao{
Goods goods=new Goods();
@Override
public boolean accountNumber(String ia) throws Exception {
StringBuffer buffer = new StringBuffer();
BufferedReader bf= new BufferedReader(new FileReader("D:\\大二上\\JAVA\\JAVA项目\\ShoppingCart(1.3-DAO)\\src\\shoppingcart\\data\\Useraccount.txt"));
String s = null;
while((s = bf.readLine())!=null){
//使用readLine方法,一次读一行
buffer.append(s.trim());
}
String s1 = buffer.toString();
if(s1.equals(ia)) return true;
else return false;
}
@Override
public boolean passwordNumber(String ip) throws Exception {
StringBuffer buffer = new StringBuffer();
BufferedReader bf= new BufferedReader(new FileReader("D:\\大二上\\JAVA\\JAVA项目\\ShoppingCart(1.3-DAO)\\src\\shoppingcart\\data\\Userpassword.txt"));
String s = null;
while((s = bf.readLine())!=null){
buffer.append(s.trim());
}
String s2 = buffer.toString();
if(s2.equals(ip)) return true;
else return false;
}
@Override
public void pickUp() throws IOException {
//输入对话框
StringBuffer buffer = new StringBuffer();
BufferedReader bf= new BufferedReader(new FileReader("D:\\大二上\\JAVA\\JAVA项目\\ShoppingCart(1.3-DAO)\\src\\shoppingcart\\data\\Userpassword.txt"));
String s = null;
while((s = bf.readLine())!=null){
//使用readLine方法,一次读一行
buffer.append(s.trim());
}
String password = buffer.toString();
String buypassword = JOptionPane.showInputDialog("*请输入密码:");
while(true) {
if(password.equals(buypassword)) {
JOptionPane.showMessageDialog(null, "密码正确!");
break;
}
else {
JOptionPane.showMessageDialog(null, "密码错误!请重试!");
break;
}
}
//选择对话框:获得用户的选择结果
int choice = JOptionPane.showConfirmDialog(null, "共计"+goods.total +"元 是否支付?");
System.out.println("choice"+choice);
switch(choice){
case JOptionPane.YES_OPTION:{
System.out.println("yes");
JOptionPane.showMessageDialog(null, "支付成功!");
}
break;
case JOptionPane.NO_OPTION:{
System.out.println("no");
JOptionPane.showMessageDialog(null, "支付失败!");
}
break;
case JOptionPane.CANCEL_OPTION:{
System.out.println("cancle");
JOptionPane.showMessageDialog(null, "用户取消支付!");
}
break;
}
}
}
Goods类
package shoppingcart;
public class Goods {
private String name[]= {
"六神花露水(玻璃瓶195ml)","得力固体胶(净含量9g)","小牛电动车(GOVA白色)","冰露纯净水(中瓶装500ml)"};
private int number[]= {
1,2,3,4};
private int quantity[]= {
0,0,0,0};
private double price[]= {
16.0,1.0,2699.0,1.0};
private double account[]= {
0,0,0,0};
double total=0;
public int[] getNumber() {
return number;
}
public void setNumber(int number[]) {
this.number = number;
}
public String[] getName() {
return name;
}
public void setName(String name[]) {
this.name = name;
}
public int[] getQuantity() {
return quantity;
}
public void setQuantity(int quantity[]) {
this.quantity = quantity;
}
public double[] getAccount() {
return account;
}
public void setAccount(double account[]) {
this.account = account;
}
public double[] getPrice() {
return price;
}
public void setPrice(double price[]) {
this.price = price;
}
}//商品属性
User类
package shoppingcart;
import java.util.Scanner;
public class User {
public static void main(String[] args) throws Exception {
Scanner in = new Scanner(System.in);
OrderDao order=new OrderDaoImpl();
GoodsDao goods=new GoodsDaoImpl();
CartDao cart=new CartDaoImpl();
System.out.println("————————欢迎来到购物车系统!————————");
System.out.println("【1】登录 ");
System.out.println("【其它数字】退出 ");
int choice1 = in.nextInt();
if(choice1==1) {
while(true) {
System.out.println("*请输入账号:");
String ia=in.next();
if(order.accountNumber(ia)) {
System.out.println("*输入正确!");
break;
}
else {
System.out.println("*输入错误请重试!");
continue;
}
}
while(true) {
System.out.println("*请输入密码:");
String ip=in.next();
if(order.passwordNumber(ip)) {
System.out.println("*输入正确!");
break;
}
else {
System.out.println("*输入错误请重试!");
continue;
}
}
}
else {
System.out.println("*退出成功!");
System.exit(1);
}
//登录部分
int choice2;
int nuin;
while(true){
System.out.println("请选择功能:");
System.out.println("【1】展示商城");
System.out.println("【2】展示购物车");
System.out.println("【3】添加商品");
System.out.println("【4】删除商品");
System.out.println("【5】清空购物车");
System.out.println("【6】买单");
System.out.println("【其它数字】退出");
choice2 = in.nextInt();
switch(choice2) {
case 1:{
goods.showGoods();
}
break;
//功能1展示商城商品
case 2:{
System.out.println("*购物车如下:");
cart.showCart();
}
break;
//功能2展示购物车内商品
case 3:{
System.out.println("*请选择添加方式:按【0】编号/【1】名称");
int choice3 = in.nextInt();
if(choice3==0) {
System.out.println("*请输入商品编号:");
int nu = in.nextInt();
while(true) {
if(goods.searchNumber(nu)>=0&&goods.searchNumber(nu)<=3) {
System.out.println("*请输入要添加的数量:");
nuin = in.nextInt();
cart.addCart(goods.searchNumber(nu),nuin);
System.out.println("*添加成功!");
break;
}
else {
System.out.println("*查无此商品,请重试!");
break;
}
}
}
else{
System.out.println("*请输入商品名称:");
String na = in.next();
while(true) {
if(goods.searchName(na)>=0&&goods.searchName(na)<=3) {
System.out.println("*请输入要添加的数量:");
nuin = in.nextInt();
cart.addCart(goods.searchName(na),nuin);
System.out.println("*添加成功!");
break;
}
else {
System.out.println("*查无此商品,请重试!");
break;
}
}
}
}
break;
//功能3向购物车内添加商品
case 4:{
System.out.println("*请选择删除方式:按【0】编号/【1】名称");
int choice4 = in.nextInt();
if(choice4==0) {
System.out.println("*请输入商品编号:");
int nu = in.nextInt();
while(true) {
if(goods.searchNumber(nu)>=0&&goods.searchNumber(nu)<=3) {
System.out.println("*请输入要删除的数量:");
nuin = in.nextInt();
if(cart.deleteCart(goods.searchNumber(nu), nuin)) {
System.out.println("*删除成功!");
break;
}
else {
System.out.println("*删除失败!");
System.out.println("*删除数量大于购物车内该商品的数量,请重试!");
break;
}
}
else {
System.out.println("*查无此商品,请重试!");
break;
}
}
}
else{
System.out.println("*请输入商品名称:");
String na = in.next();
while(true) {
if(goods.searchName(na)>=0&&goods.searchName(na)<=3) {
System.out.println("*请输入要删除的数量:");
nuin = in.nextInt();
if(cart.deleteCart(goods.searchName(na), nuin)) {
System.out.println("*删除成功!");
break;
}
else {
System.out.println("*删除失败!");
System.out.println("*删除数量大于购物车内该商品的数量,请重试!");
break;
}
}
else {
System.out.println("*查无此商品,请重试!");
break;
}
}
}
}
break;
//功能4删除购物车内指定商品
case 5:{
cart.clearCart();
System.out.println("*清空成功!是否查看购物车?【1】是【0】否");
int choice5=in.nextInt();
if(choice5==0)break;
else cart.showCart();
}
break;
//功能5清空购物车内商品信息
case 6:{
order.pickUp();
System.exit(1);
}
break;
//功能6结账
default:{
System.out.println("*退出成功!");
System.exit(1);
}
//其它退出
}
}
}
}