数据结构
线性表可以用数组和链表来实现
目前的栈和队列的封装都是基于数组(因为js里面对于数组的函数有很多,在开始会比较容易)
栈
function Stack() {
this.items = [];
Stack.prototype.push = function (element) {
this.items.push(element);
}
Stack.prototype.pop = function () {
return this.items.pop();//返回被移除的内容
}
Stack.prototype.peek = function () {
return this.items[this.items.length - 1];
}
Stack.prototype.isEmpty = function () {
return this.items.length == 0;
}
Stack.prototype.size = function () {
return this.items.length;
}
Stack.prototype.toString = function () {
//内容被空格分开
let newstr = '';
for (let i = 0; i < this.items.length; i++) {
newstr += this.items[i] + ' ';
}
return newstr;
}
}
简单的栈的功能的实现(主要js是面向对象语言,很多功能都已经封装出功能了,所以自己封装的时候利用那些功能会很方便自己的封装,希望有时间可以用c语言来封装实现一下这个功能)
function decTobin(decNum) {
let stack = new Stack();//余数入栈
let bin = "";
while(decNum > 0){
stack.push(decNum % 2);
decNum = Math.floor(decNum / 2); //向下取整
}
while(!stack.isEmpty()){
bin += stack.pop() + '';
}
return bin;
}
简单的十进制转二进制
队列
一下是常见操作
function Queue() {
// 属性
this.items = [];
// 方法
Queue.prototype.enqueue = function (element) {
this.items.push(element);
}
Queue.prototype.dequeue = function () {
return this.items.shift();
}
Queue.prototype.front = function () {
return this.items[0];
}
Queue.prototype.isEmpty = function () {
return this.items.length == 0;
}
Queue.prototype.size = function () {
return this.items.length;
}
Queue.prototype.toString = function () {
let newstr = '';
for (let i = 0; i < this.items.length; i++) {
newstr += this.items[i] + ' ';
}
return newstr;
}
}
简单的基于js数组的队列封装
function passGame(nameList, num) {
let queue = new Queue();
for (let i = 0; i < nameList.length; i++) {
queue.enqueue(nameList[i]);
}
while(queue.size() > 1){
// 这个最重要的点,当不是num的时候把这个数字加在队尾
for (let i = 0; i < num - 1; i++) {
queue.enqueue(queue.dequeue());
}
queue.dequeue();//将num淘汰
}
console.log(queue.front());
return nameList.indexOf(queue.front());
}
let name = ['1','2','3','4','5'];
alert(passGame(name, 3));
简单的击鼓传花小demo
扫描二维码关注公众号,回复:
13303251 查看本文章
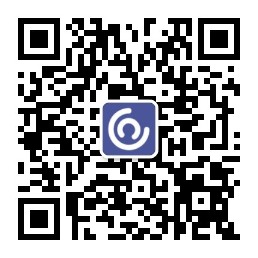
优先级队列
优先级队列就是在队列的基础上在进行内容的插入的时候要考虑优先级
这个是优先级队列的封装,其他的内容和上次的基本一样
function PriorityQueue() {
function QueueElement(element, priority) {
this.element = element;
this.priority = priority;
}
this.items = [];
PriorityQueue.prototype.enqueue = function (element, priority) {
var queueElement = new QueueElement(element, priority);
if (this.items.length == 0) {
this.items.push(queueElement);
}else{
var added = false;
for (let i = 0; i < this.items.length; i++) {
if (queueElement.priority > this.items[i].pirority) {
this.items.splice(i, 0, queueElement);//删除0个元素,向i处添加新元素
added = true;
break;
}
}
if (!added) {
this.items.push(queueElement)
}
}
}
}