实现效果如图:
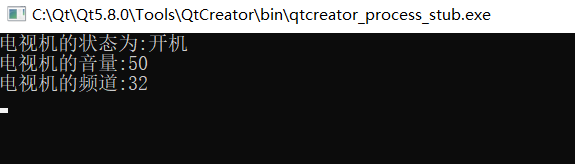
代码如下:
#include <iostream>
using namespace std;
class TV
{
friend class Remote;
enum{
On,Off };
enum{
minVol,maxVol = 100 };
enum{
minChannel = 1,maxChannel = 255 };
private:
int mState;
int mVolume;
int mChannel;
public:
TV()
{
this->mState = Off;
this->mVolume = minVol;
this->mChannel = minChannel;
}
void onOrOff(void)
{
this->mState = (this->mState == On ? Off:On);
}
void volumeUp(void)
{
if(this->mVolume >= maxVol)
return;
this->mVolume++;
}
void volumeDown(void)
{
if(this->mVolume <= minVol)
return;
this->mVolume--;
}
void channelUp(void)
{
if(this->mChannel >= maxChannel)
return;
this->mChannel++;
}
void channelDown(void)
{
if(this->mChannel <= minChannel)
return;
this->mChannel--;
}
void showTVState(void)
{
cout<<"电视机的状态为:"<< (this->mState==On ? "开机":"关机") <<endl;
cout<<"电视机的音量:"<<this->mVolume<<endl;
cout<<"电视机的频道:"<<this->mChannel<<endl;
}
};
class Remote
{
private:
TV *pTv;
public:
Remote(TV *pTv)
{
this->pTv = pTv;
}
void volumeUp(void)
{
this->pTv->volumeUp();
}
void volumeDown(void)
{
this->pTv->volumeDown();
}
void channelUp(void)
{
this->pTv->channelUp();
}
void channelDown(void)
{
this->pTv->channelDown();
}
void onOrOff(void)
{
this->pTv->onOrOff();
}
void setChannel(int num)
{
if(num >= TV::minChannel && num<= TV::maxChannel )
{
this->pTv->mChannel = num;
}
}
void setvolumep(int num)
{
if(num>=TV::minVol && num<=TV::maxVol)
{
this->pTv->mVolume=num;
}
}
void showTVState(void)
{
this->pTv->showTVState();
}
};
void test()
{
TV tv;
Remote remote(&tv);
remote.onOrOff();
remote.setvolumep(50);
remote.setChannel(32);
remote.showTVState();
}
int main(int argc, char *argv[])
{
test();
return 0;
}