实现效果如图:
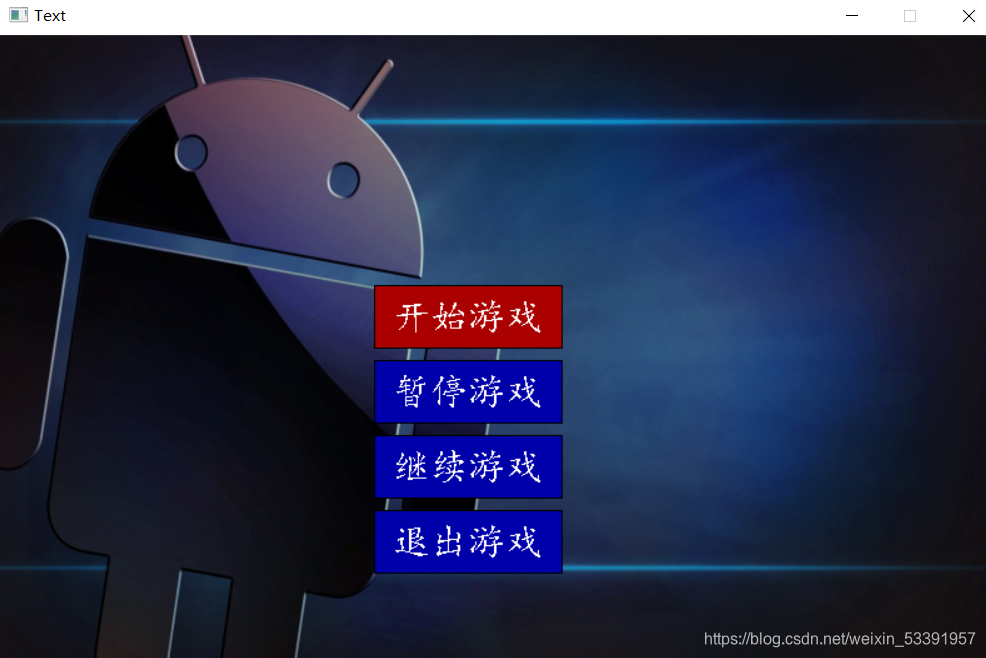
代码如下:
#include<stdio.h>
#include<graphics.h>
#include<conio.h>
#include<string.h>
typedef struct Button
{
int x;
int y;
int xx;
int yy;
COLORREF color;
char* buttonstr;
}*LPBTN;
LPBTN createButton(int x, int y, int xx, int yy, COLORREF color, const char* buttonstr)
{
LPBTN button = (LPBTN)malloc(sizeof(LPBTN));
button->x = x;
button->y = y;
button->xx = xx;
button->yy = yy;
button->color = color;
button->buttonstr = (char*)malloc(sizeof(char));
strcpy(button->buttonstr, buttonstr);
return button;
}
void drawButton(LPBTN button)
{
setfillcolor(button->color);
fillrectangle(button->x, button->y, button->xx, button->yy);
setbkmode(TRANSPARENT);
setlinecolor(BLACK);
settextstyle(30, 0, "楷体");
outtextxy(button->x + 15, button->y + 10, button->buttonstr);
}
int isInButton(LPBTN button, MOUSEMSG m)
{
if (button->x <= m.x && button->xx >= m.x && button->y <= m.y && button->yy >= m.y)
{
return 1;
}
return 0;
}
void buttonAction(LPBTN button, MOUSEMSG m)
{
if (isInButton(button, m))
{
button->color = RED;
}
else
{
button->color = BLUE;
}
}
int main()
{
LPBTN play = createButton(300, 200, 450, 250, BLUE, "开始游戏");
LPBTN pause = createButton(300, 260, 450, 310, BLUE, "暂停游戏");
LPBTN resume = createButton(300, 320, 450, 370, BLUE, "继续游戏");
LPBTN close = createButton(300, 380, 450, 430, BLUE, "退出游戏");
initgraph(800, 500);
IMAGE picture;
loadimage(&picture, "background.jpg", 800, 500);
putimage(0, 0, &picture);
MOUSEMSG m;
while (1)
{
BeginBatchDraw();
drawButton(play);
drawButton(pause);
drawButton(resume);
drawButton(close);
m = GetMouseMsg();
buttonAction(play, m);
buttonAction(pause, m);
buttonAction(resume, m);
buttonAction(close, m);
EndBatchDraw();
}
getch();
closegraph();
return 0;
}