目录
1、Collection集合的遍历方式之一:Iterator迭代器
前言
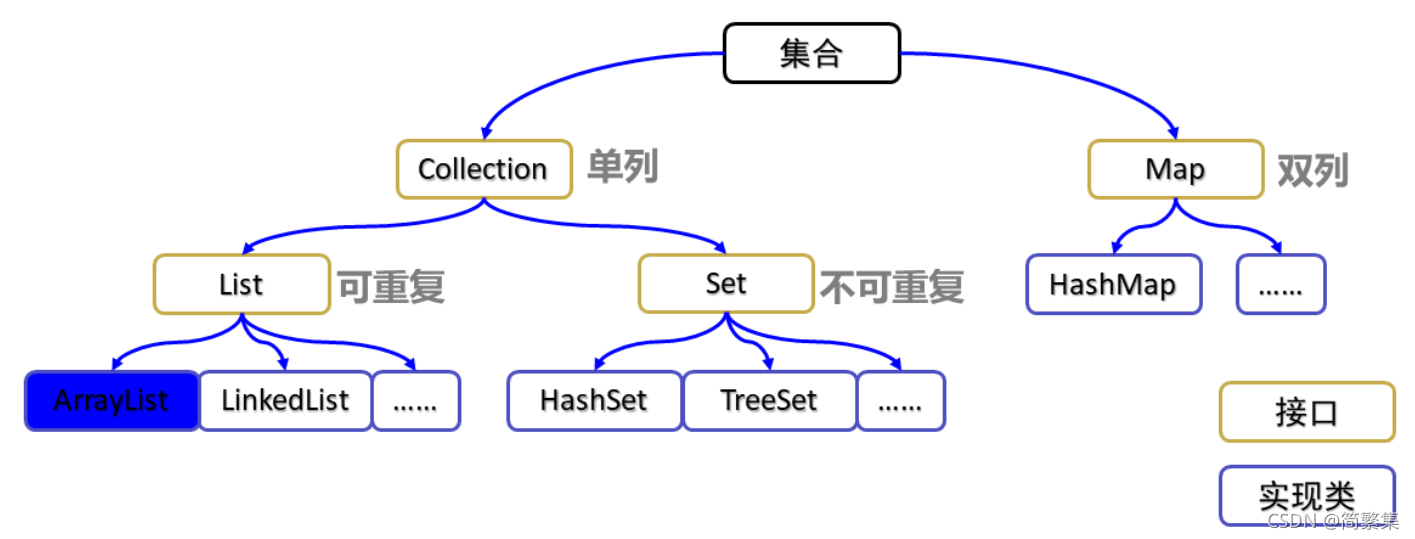
- Collection:这是个接口。单列集合接口。
- List:元素可重复的、有序的集合接口。
- ArrayList:是底层为数组,查询效率高的实现类。
- LinkedList:是底层为链表,增删效率高的实现类。
- Set:元素不可重复、无序的、没有带索引的方法的集合接口。
- HashSet:是底层为哈希表,元素不可重复,且存入和读取元素顺序不一致(即无序)的实现类。
- TreeSet:是底层为红黑树,元素不可重复,可以用自然排序Comparable 或者排序器Comparator 给元素排序的实现类。
- List:元素可重复的、有序的集合接口。
- Map:也是个接口。双列集合接口。
- HashMap:是底层为哈希表,key不能重复且无序的实现类。
- TreeMap:是底层为红黑树,key不能重复,可以用自然排序 / 排序器实现元素排序的实现类。
一、创建集合对象
1、接口怎么创建集合对象
采用多态的方式通过具体实现类创建对象。
比如:
Collection | Collection<String> collection = new ArrayList<String>(); |
List | List<String> list = new ArrayList<>(); |
Set | Set<String> set = new HashSet<>(); |
Map | Map<String, String> map = new HashMap<String, String>(); |
2、实现类怎么创建集合对象
用new创建。
比如:
ArrayList | ArrayList<String> arrayList = new ArrayList<>(); |
LinkedList | LinkedList<String> linkedList = new LinkedList<>(); |
HashSet | HashSet<String> hs = new HashSet<>(); |
TreeSet | TreeSet<Integer> ts = new TreeSet<Integer>(); |
HashMap | HashMap<String, String> hashMap = new HashMap<String, String>(); |
TreeMap | TreeMap<String, String> treeMap= new TreeMap<String, String>(); |
二、不同集合对象的遍历
一般我们都是用普通for循环遍历访问元素,但在这里如果仍旧使用普通for循环遍历,会出现一些问题:效率不高、集合对象没有索引方法等等。下面就来讲讲不同集合对象怎么遍历访问元素。
1、Collection集合的遍历方式之一:Iterator迭代器
Iterator迭代器是集合的专用的遍历方式。通过集合的iterator() 方法得到,所有我们说它是依赖于集合而存在的。
Iterator<E> iterator() ,返回此集合中元素的迭代器。
1.1 Iterator 中的常用方法
E next() | 返回迭代中的下一个元素。 |
boolean hasNext() | 如果迭代具有更多元素,则返回 true 。 |
1.2 代码示例
//创建集合对象
Collection<String> c = new ArrayList<String>();
//添加元素
c.add("hello");
c.add("world");
c.add("java");
//Iterator<E> iterator():返回此集合中元素的迭代器,通过集合的iterator()方法得到
Iterator<String> it = c.iterator();
//用while循环判断
while (it.hasNext()) {
String s = it.next();
System.out.println(s);
}
运行结果 | ![]() |
其他遍历Collection 集合的方法:普通for循环、增强for。
2、Set集合的遍历 —— 增强for
由于Set 集合中没有带索引的方法,所以不能用普通的for循环遍历,可以使用Iterator迭代器或者增强for。前面已经讲解了Iterator迭代器的使用,这里就举个增强for的例子遍历Set 集合。
//创建Set集合对象
Set<String> set = new HashSet<>();
//添加元素
set.add("hello,");
set.add("world!");
//遍历集合
for (String s : set){
System.out.println(s);
}
运行结果 | ![]() |
3、Map集合的遍历方式一:键找值
- 获取所有键的集合。用keySet()方法实现
- 遍历键的集合,获取到每一个键。用增强for实现
- 根据键去找值。用get(Object key)方法实现
//创建集合对象
Map<String, String> map = new HashMap<String, String>();
//添加元素
map.put("牛奶", "奶牛");
map.put("蜜蜂", "蜂蜜");
map.put("上海", "海上");
//获取所有键的集合。用keySet()方法实现
Set<String> keySet = map.keySet();
//遍历键的集合,获取到每一个键。用增强for实现
for (String key : keySet) {
//根据键去找值。用get(Object key)方法实现
String value = map.get(key);
System.out.println(key + "," + value);
}
运行结果 | ![]() |
4、Map集合的遍历方式二:键值对对象找键和值
- 获取所有键值对对象的集合
- Set<Map.Entry<K,V>> entrySet():获取所有键值对对象的集合
- 遍历键值对对象的集合,得到每一个键值对对象
- 用增强for实现,得到每一个Map.Entry
- 根据键值对对象获取键和值
- 用getKey()得到键
- 用getValue()得到值
//创建集合对象
Map<String, String> map = new HashMap<String, String>();
//添加元素
map.put("张无忌", "赵敏");
map.put("郭靖", "黄蓉");
map.put("杨过", "小龙女");
//获取所有键值对对象的集合
Set<Map.Entry<String, String>> entrySet = map.entrySet();
//遍历键值对对象的集合,得到每一个键值对对象
for (Map.Entry<String, String> me : entrySet) {
//根据键值对对象获取键和值
String key = me.getKey();
String value = me.getValue();
System.out.println(key + "," + value);
}
运行结果 | ![]() |
三、Collection框架中实现比较要实现什么接口
要实现比较有两种方式:
第一种,实体类实现Comparable<T>接口,并实现 compareTo(T t) 方法,我们称为内部比较器、自然排序。
示例:直接在学生类中实现Comparable<T>接口,并重写compareTo(T t)方法。
public class Student implements Comparable<Student> {
private String name;
private int age;
//有参/无参构造方法
……
//getter and setter方法
……
@Override
public int compareTo(Student o) {
//年龄从小到大排序
int num = this.age - o.age;//升序
//int num = o.age - this.age;//降序
//年龄相同时,按照姓名的字母顺序排序
int num2 = num == 0?this.name.compareTo(o.name):num;
return num2;
}
}
第二种,创建一个外部比较器,这个外部比较器要实现Comparator接口的 compare(T t1, T t2)。
示例:在创建对象时使用匿名内部类实现Comparator接口,并重写compare(T t1, T t2)方法。
public class Student {
private String name;
private int age;
//有参/无参构造方法
……
//getter and setter方法
……
}
TreeSet<Student> ts = new TreeSet<>(new Comparator<Student>() {
@Override
public int compare(Student o1, Student o2) {
//年龄从小到大排序
int num = o1.getAge() - o2.getAge();
//年龄相同时,按照姓名的字母顺序排序
int num2 = num==0?o1.getName().compareTo(o2.getName()):num;
return num2;
}
});
集合没什么好讲的,主要就是了解各种集合对象的特点,根据不同的需求使用不同的集合对象。当然如果面试的时候被问到了集合,你需要关注了解的不单单是它们的特点,更多的是它们的底层和原理。Java集合的面试题,大家可以自行百度搜索。