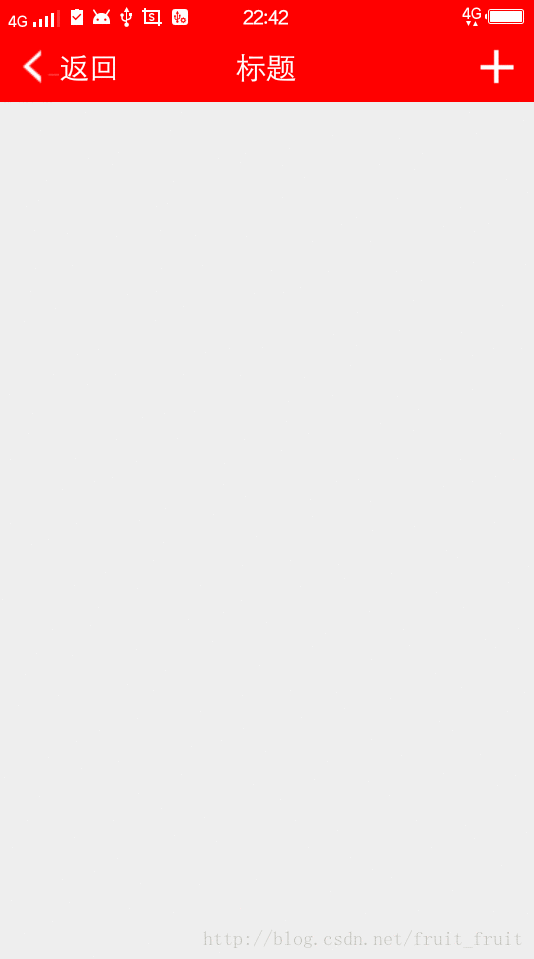
public class My extends Toolbar {
TextView textLeft;
TextView textCenter;
TextView textRight;
public My(Context context) {
super(context,null);
}
public My(Context context, @Nullable AttributeSet attrs) {
super(context, attrs,0);
}
public My(Context context, @Nullable AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
}
@Override
protected void onFinishInflate() {
super.onFinishInflate();
textLeft=(TextView) this.findViewById(R.id.txt_left);
textCenter=(TextView) this.findViewById(R.id.txt_center);
textRight=(TextView) this.findViewById(R.id.txt_right);
}
/**
* 设置中间文本框内容
* @param text 字符串对像
*/
public void setCenterTitle(String text){
this.setTitle("");
textCenter.setVisibility(VISIBLE);
textCenter.setText(text);
}
public void setCenterColor(int color){
textCenter.setTextColor(color);
}
public void setLeftText(String text){
this.setTitle("");
textLeft.setVisibility(VISIBLE);
textLeft.setText(text);
}
public void setLeftColor(int color){
textCenter.setTextColor(color);
}
/**
* 获取左侧文本框图片资源
* @param res
*/
public void setLeftDrawable(int res){
Drawable drawable= ContextCompat.getDrawable(getContext(),res);
drawable.setBounds(0,0,drawable.getMinimumWidth(),drawable.getMinimumHeight());
textLeft.setCompoundDrawables(drawable,null,null,null);
}
/**
* 左侧文本框绑定监听事件
* @param onClickListener
*/
public void setLeftListener(OnClickListener onClickListener){
textLeft.setOnClickListener(onClickListener);
}
/**
* 设置右侧文本框文字信息
* @param title
*/
public void setRightTitle(String title){
this.setTitle("");
textRight.setVisibility(VISIBLE);
textRight.setText(title);
}
/**
* 设置右侧文本框文字颜色
* @param color 颜色
*/
public void setRightColor(int color){
textRight.setTextColor(color);
}
/***
* 设置右侧文本框图片资源
* @param res 资源名
*/
public void setRightDrawable(int res){
Drawable drawable=ContextCompat.getDrawable(getContext(),res);
drawable.setBounds(0,0,drawable.getMinimumWidth(),drawable.getMinimumHeight());
textRight.setCompoundDrawables(drawable,null,null,null);
}
public void setRightListener(OnClickListener onClickListener){
textRight.setOnClickListener(onClickListener);
}
}
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="wz.com.mytoolbar.MainActivity">
<wz.com.mytoolbar.My
android:layout_width="match_parent"
android:layout_height="45dp"
android:layout_alignParentTop="true"
android:layout_alignParentStart="true"
android:background="@color/colorPrimaryDark"
android:fitsSystemWindows="true"
app:contentInsetLeft="0dp"
app:contentInsetStart="0dp"
android:id="@+id/my">
<TextView
android:textSize="20sp"
android:id="@+id/txt_left"
android:text="返回"
android:textColor="@color/textToolBarColor"
android:layout_marginLeft="10dp"
android:gravity="center"
android:visibility="visible"
android:lines="1"
android:drawableLeft="@drawable/call_back"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
tools:ignore="HardcodedText,RtlHardcoded" />
<TextView
android:textSize="20sp"
android:id="@+id/txt_center"
android:text="标题"
android:textColor="@color/textToolBarColor"
android:layout_gravity="center"
android:lines="1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
tools:ignore="HardcodedText" />
<TextView
android:drawableRight="@drawable/more_pic"
android:layout_gravity="right"
android:layout_marginRight="10dp"
android:gravity="center"
android:textSize="16sp"
android:visibility="visible"
android:id="@+id/txt_right"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
tools:ignore="RtlHardcoded" />
</wz.com.mytoolbar.My>
</RelativeLayout>
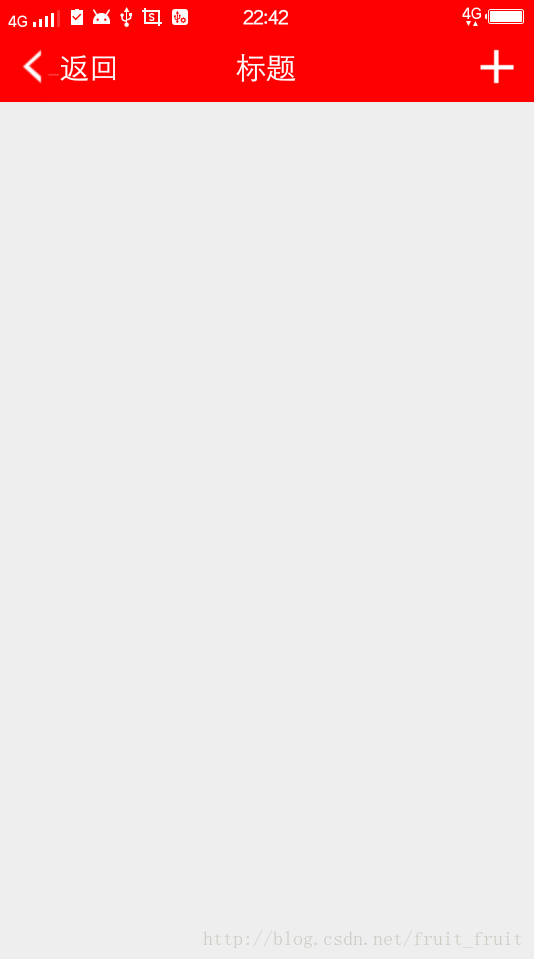
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
My my=(My) this.findViewById(R.id.my);
my.onFinishInflate();
my.setLeftListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Toast.makeText(MainActivity.this,"你点击了返回按钮",Toast.LENGTH_SHORT).show();
}
});
my.setRightListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Toast.makeText(MainActivity.this,"你点击了更多按钮",Toast.LENGTH_SHORT).show();
}
});
}
}