一、彩色图像变换灰度图像
1、彩色位图图像的颜色
图像像素的颜色由三种基本色,即红R、绿G、蓝B,称为三基色。每种基色可取0-255(占用8个位,即1个字节),因此一个像素的颜色可以由三基色可组合成1677万种颜色(255*255*255)。
R值 | G值 | B值 | |
红 | 255 | 0 | 0 |
蓝 | 0 | 0 | 255 |
绿 | 0 | 255 | 0 |
黄 | 255 | 255 | 0 |
青 | 0 | 255 | 255 |
品红 | 255 | 0 | 255 |
白 | 255 | 255 | 255 |
黑 | 0 | 0 | 0 |
2、彩色图像颜色的值的获取
在使用C#系统处理彩色图像时,使用Bitmap类的GetPixel方法获取图像上指定像素的颜色值。
Color c1 = new Color();
c1 = box1.GetPixel(i,j);
其中,(i,j)为像素的坐标位置。GetPixel方法取得指定位置的颜色值并返回一个长整型的整数。像素颜色值c1是一个长整型的数值,占4个字节,最上位字节的值为0,其他三个下位字节依次为B、G、R,值为0-255。
比如:Bitmap.GetPixel(Int32, Int32) 方法
3、彩色位图颜色值分解
从c1值中分解出的R、G、B值可以直接使用:
Color c1 = new Color();
c1 = box1.GetPixel(i,j);
r = c1.R;
g = c1.G;
b = c1.B;
4、图像像素颜色的设定
设置像素可使用SetPixel方法。
Color c1 = Color.FromArgb(r,g,b);
//Color.FromArgb基于一个 32 位 ARGB 值创建 Color 结构。
Box2.SetPixel(i,j,c1);
比如:Bitmap.SetPixel(Int32, Int32, Color) 方法
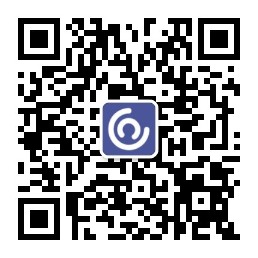
5、示例
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace _20220922_1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
OpenFileDialog ofd1 = new OpenFileDialog();//打开文件对话框
ofd1.Filter = "BMP|*.png";//定义选择的文件格式
if(ofd1.ShowDialog() == DialogResult.OK)//如果成功打开文件对话框
{
Bitmap image1 = new Bitmap(ofd1.FileName);
pictureBox1.Image = image1;//显示图像
}
}
private void button2_Click(object sender, EventArgs e)
{
Color c1 = new Color();
Bitmap box1 = new Bitmap(pictureBox1.Image);//
Bitmap box2 = new Bitmap(pictureBox1.Image);
int r, g, b, c2;
for (int i= 0;i<pictureBox1.Image.Width;i++)
{
for(int j=0;j<pictureBox1.Image.Height;j++)
{
c1 = box1.GetPixel(i,j);
r = c1.R;
g = c1.G;
b = c1.B;
c2 = (int)((r+g+b)/3);
if (c2 < 0)
c2 = 0;
if (c2 > 255)
c2 = 255;
Color c11 = Color.FromArgb(c2, c2, c2);//将整形转换为颜色值
box2.SetPixel(i, j, c11);//将新的值,逐步输入到box2中
}
pictureBox2.Refresh();//Control.Refresh 方法,强制控件使其工作区无效并立即重绘自己和任何子控件。
pictureBox2.Image = box2;
}
}
}
}
二、图像的模糊化处理
图象分辨率(ImageResolution):指图象中存储的信息量。这种分辨率有多种衡量方法,典型的是以每英寸的像素数(PPI)来衡量。图象分辨率和图象尺寸(高宽)的值一起决定文件的大小及输出的质量,该值越大图形文件所占用的磁盘空间也就越多。图象分辨率以比例关系影响着文件的大小, 即文件大小与其图象分辨率的平方成正比。如果保持图象尺寸不变,将图象分辨率提高一倍,则其文件大小增大为原来的四倍。
马赛克的原理就是将原图像分成4×4(或其他)像素的子图像块,该4×4像素子图像块的所有像素的颜色按F(i,j)的颜色来进行设定,达到降低分辨率的目的。
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace _20220922_1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
OpenFileDialog ofd1 = new OpenFileDialog();//打开文件对话框
ofd1.Filter = "BMP|*.png";//定义选择的文件格式
if(ofd1.ShowDialog() == DialogResult.OK)//如果成功打开文件对话框
{
Bitmap image1 = new Bitmap(ofd1.FileName);
pictureBox1.Image = image1;//显示图像
}
}
private void button2_Click(object sender, EventArgs e)
{
Color c1 = new Color();
Bitmap box1 = new Bitmap(pictureBox1.Image);
Bitmap box2 = new Bitmap(pictureBox1.Image);
int size, k1, k2, xres, yres;
xres = pictureBox1.Image.Width;
yres = pictureBox1.Image.Height;
size = 8;
for(int i = 0; i <= xres-1; i += size)
{
for(int j = 0; j <= yres-1; j += size)
{
c1 = box1.GetPixel(i, j);
for(k1 = 0; k1 <= size-1; k1++)
{
for(k2 = 0; k2 <= size-1; k2++)
{
if (i + k1 < pictureBox1.Image.Width && j + k2 < pictureBox1.Image.Height)
box2.SetPixel(i + k1, j + k2, c1);
}
}
}
pictureBox2.Refresh();
pictureBox2.Image = box2;
}
}
}
}
三、灰度图像处理
对图像像素的处理,可以达到灰度、对比度调节的效果。根据特定的输入输出灰度转换关系,可增强图像灰度,处理后图像的中等灰度值增大,图像变亮。
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace _20220922_1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
OpenFileDialog ofd1 = new OpenFileDialog();//打开文件对话框
ofd1.Filter = "BMP|*.png";//定义选择的文件格式
if(ofd1.ShowDialog() == DialogResult.OK)//如果成功打开文件对话框
{
Bitmap image1 = new Bitmap(ofd1.FileName);
pictureBox1.Image = image1;//显示图像
}
}
private void button2_Click(object sender, EventArgs e)
{
Color c1 = new Color();
Bitmap box1 = new Bitmap(pictureBox1.Image);
Bitmap box2 = new Bitmap(pictureBox1.Image);
int rr,m,lev,wid;
int[] lut = new int [256];
int[,,]pic = new int[700,700,3];
double dm;
lev = 5;//设置灰度值
wid = 180;//设置对比度值
for (int x = 0; x<256;x +=1)
{
lut[x]=255;
}
for(int x = lev; x < (lev+wid); x++)
{
dm = ((double)(x-lev)/(double)wid) * 255f;
lut[x] = (int)dm;
}
for(int i = 0;i<pictureBox1.Image.Width-1;i++)
{
for(int j = 0;j<pictureBox1.Image.Height-1;j++)
{
c1 = box1.GetPixel(i,j);
m = (int)((c1.R+c1.G+c1.B)/3);
rr = lut[m];
Color c2 = Color.FromArgb(rr,rr,rr);
box2.SetPixel(i,j,c2);
}
}
pictureBox2.Refresh();
pictureBox2.Image = box2;
}
}
}