文章目录
提示:以下是本篇文章正文内容,下面案例可供参考
一、为什么学习OpenFeign?
- 在之前我们都是通过调用RestTemplate 来实现远程调用的。使用RestTemplate有一个问题:繁琐,每个请求除了参数不同、请求地址不同、返回数据不同,其他都是一样的,所以我们希望能够简化,简化的方案就是 OpenFeign。
- OpenFeign 是 Spring cloud 团队在
Netflix Feign
基础上开发出来的声明式调用组件
二、OpenFeign和Feign介绍和区别
1.Feign是什么
Feign是一个声明式WebService客户端。使用Feign能让编写Web Service客户端更加简单。
Feign集成了Ribbon、RestTemplate实现了负载均衡的执行Http调用,只不过对原有的方式(Ribbon+RestTemplate)进行了封装,开发者不必手动使用RestTemplate调服务,而是定义一个接口,在这个接口中标注一个注解即可完成服务调用,这样更加符合面向接口编程的宗旨,简化了开发。
2.OpenFeign是什么
OpenFeign是springcloud在Feign的基础上支持了SpringMVC的注解,如@RequestMapping等等。
OpenFeign的@FeignClient可以解析SpringMVC的@RequestMapping注解下的接口,并通过动态代理的方式产生实现类,实现类中做负载均衡并调用其他服务。
OpenFeign的官网地址:https://docs.spring.io/spring-cloud-openfeign/docs/current/reference/html/
OpenFeign的Git地址:https://github.com/spring-cloud/spring-cloud-openfeign
3.Feign和OpenFeign两者区别
三、OpenFeign服务调用
服务提供者还是用之前的8001和8002支付服务
1.创建cloud-consumer-feign-order80消费者模块
2.改pom文件
<dependencies>
<!--openfeign-->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
<!--eureka client-->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
<!-- 引入自己定义的api通用包,可以使用Payment支付Entity -->
<dependency>
<groupId>com.heng</groupId>
<artifactId>cloud-api-commons</artifactId>
<version>${project.version}</version>
</dependency>
<!--web-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<!--一般基础通用配置-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
3.建yml
server:
port: 80
spring:
application:
name: cloud-feign-order-service
eureka:
client:
#表示是否将自己注册进Eurekaserver默认为true。
register-with-eureka: true
#是否从EurekaServer抓取已有的注册信息,默认为true。单节点无所谓,集群必须设置为true才能配合ribbon使用负载均衡
fetchRegistry: true
service-url:
defaultZone: http://eureka7001.com:7001/eureka, http://eureka7002.com:7002/eureka
4. 主启动类
package com.heng;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.openfeign.EnableFeignClients;
@SpringBootApplication
@EnableFeignClients
public class OrderFeignMain80 {
public static void main(String[] args) {
SpringApplication.run(OrderFeignMain80.class,args);
}
}
添加@EnableFeignClients注解,开启 OpenFeign 支持
5. 编写业务逻辑接口,使用@FeignClient
package com.heng.service;
import com.heng.entities.CommonResult;
import com.heng.entities.Payment;
import org.springframework.cloud.openfeign.FeignClient;
import org.springframework.stereotype.Component;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
@Component
@FeignClient(value ="CLOUD-PAYMENT-SERVICE")
public interface PaymentFeignService {
@GetMapping(value = "/payment/get/{id}")
public CommonResult<Payment> getPaymentById(@PathVariable("id") Long id);
}
6. 编写controller类
@RestController
@Slf4j
public class OrderFeignController {
@Resource
private PaymentFeignService paymentFeignService;
@GetMapping(value = "/consumer/payment/get/{id}")
public CommonResult<Payment> getPaymentById(@PathVariable("id") Long id) {
return paymentFeignService.getPaymentById(id);
}
}
测试:
注意:接口的方法建议和服务提供者的方法保持一致
四、OpenFeign超时控制
通过写延迟程序,测试OpenFeign超时控制
- 服务提供方8001/8002故意写暂停程序
@GetMapping(value = "/payment/feign/timeout")
public String paymentFeignTimeout()
{
// 业务逻辑处理正确,但是需要耗费3秒钟
try {
TimeUnit.SECONDS.sleep(3);
} catch (InterruptedException e) {
e.printStackTrace();
}
return serverPort;
}
- 服务消费方80添加超时方法
@Component
@FeignClient(value ="CLOUD-PAYMENT-SERVICE")
public interface PaymentFeignService {
@GetMapping(value = "/payment/get/{id}")
public CommonResult<Payment> getPaymentById(@PathVariable("id") Long id);
@GetMapping(value = "/payment/feign/timeout")
public String paymentFeignTimeout();
}
- controller编写调用方法
@GetMapping(value = "/consumer/payment/feign/timeout")
public String paymentFeignTimeout() {
// OpenFeign客户端一般默认等待1秒钟
return paymentFeignService.paymentFeignTimeout();
}
- 测试
http://localhost/consumer/payment/feign/timeout
OpenFeign默认等待1秒钟,超过后报错
通过修改配置文件,对OpenFeign客户端超时控制
#设置feign客户端超时时间(OpenFeign默认支持ribbon)(单位:毫秒)
ribbon:
#指的是建立连接所用的时间,适用于网络状况正常的情况下,两端连接所用的时间
ReadTimeout: 5000
#指的是建立连接后从服务器读取到可用资源所用的时间
ConnectTimeout: 5000
重新访问
五、OpenFeign日志增强
Feign提供了日志打印功能,我们可以通过配置来调整日恙级别,从而了解Feign 中 Http请求的细节。
对Feign接口的调用情况进行监控和输出
日志级别:
扫描二维码关注公众号,回复:
14642220 查看本文章
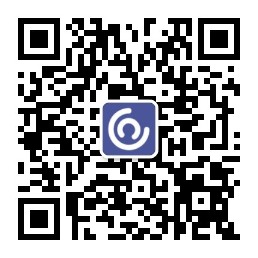
- NONE:默认的,不显示任何日志;
- BASIC:仅记录请求方法、URL、响应状态码及执行时间;
- HEADERS:除了BASIC中定义的信息之外,还有请求和响应的头信息;
- FULL:除了HEADERS中定义的信息之外,还有请求和响应的正文及元数据。
1.编写配置类,来设置日志级别
package com.heng.config;
import feign.Logger;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class FeignConfig {
@Bean
Logger.Level feignLoggerLevel()
{
return Logger.Level.FULL;
}
}
2.修改yml文件 开启日志的Feign客户端
logging:
level:
com.heng.service.PaymentFeignService: debug
当我们发起一个请求时,后台日志中能看到请求和响应的正文
整个moudle目录构成: