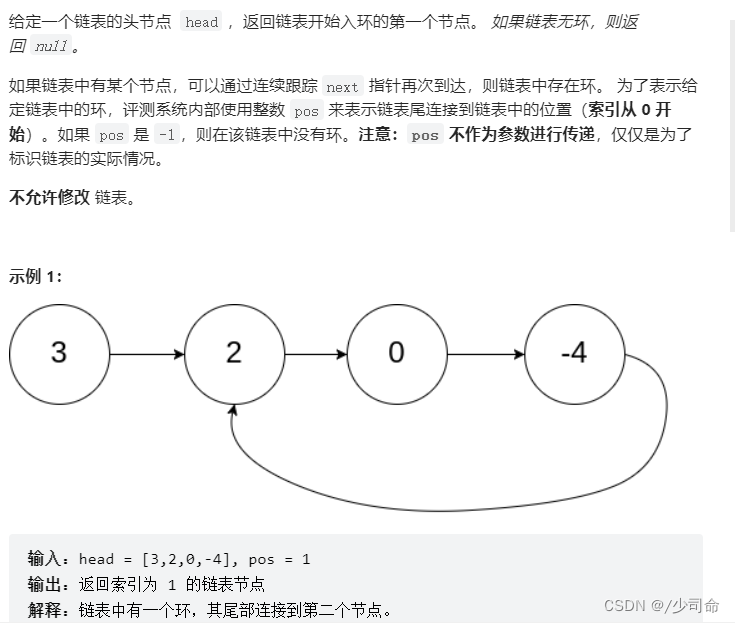
public ListNode detectCycle(ListNode head) {
ListNode pos = head;
HashSet<ListNode> set = new HashSet<>();
while (pos != null){
if (set.contains(pos)){
return pos;
}else {
set.add(pos);
}
pos = pos.next;
}
return null;
}
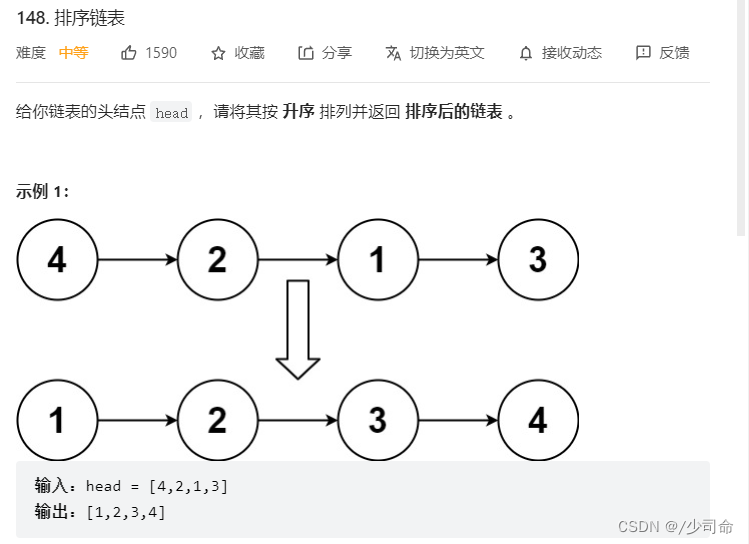
public ListNode sortList(ListNode head) {
if (head == null || head.next == null){
return head;
}
ListNode fast = head.next;
ListNode slow = head;
while (fast != null && fast.next != null){
fast = fast.next.next;
slow = slow.next;
}
ListNode tmp = slow.next;
slow.next = null;
ListNode left = sortList(head);
ListNode right = sortList(tmp);
ListNode h = new ListNode(0);
ListNode res = h;
while (left != null && right != null){
if (left.val < right.val){
h.next = left;
left = left.next;
}else {
h.next = right;
right = right.next;
}
h = h.next;
}
h.next = left != null ? left : right;
return res.next;
}
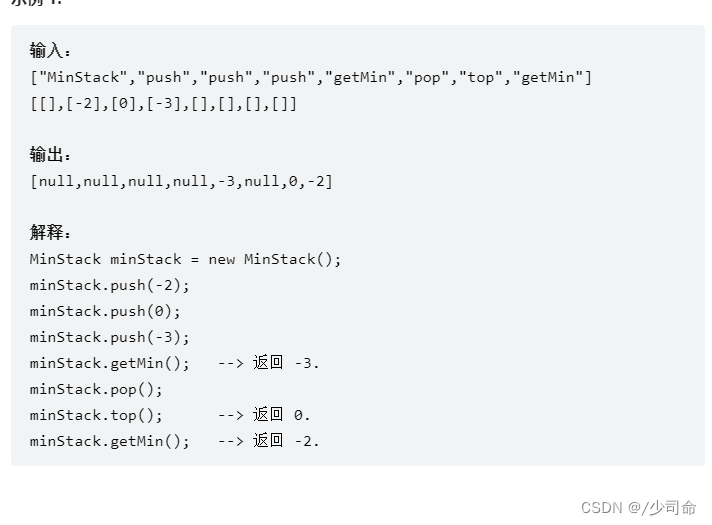
private Stack<Integer> stack;
private Stack<Integer> minStack;
public MinStack() {
stack = new Stack<>();
minStack = new Stack<>();
}
public void push(int val) {
stack.push(val);
if (minStack.empty()){
minStack.push(val);
}else {
int top = minStack.peek();
if (val <= top){
minStack.push(val);
}
}
}
public void pop() {
int val = stack.pop();
if (!minStack.empty()){
int top = minStack.peek();
if (val == top){
minStack.pop();
}
}
}
public int top() {
if (stack.empty()){
return -1;
}else {
return stack.peek();
}
}
public int getMin() {
if (minStack.empty()){
return -1;
}else {
return minStack.peek();
}
}
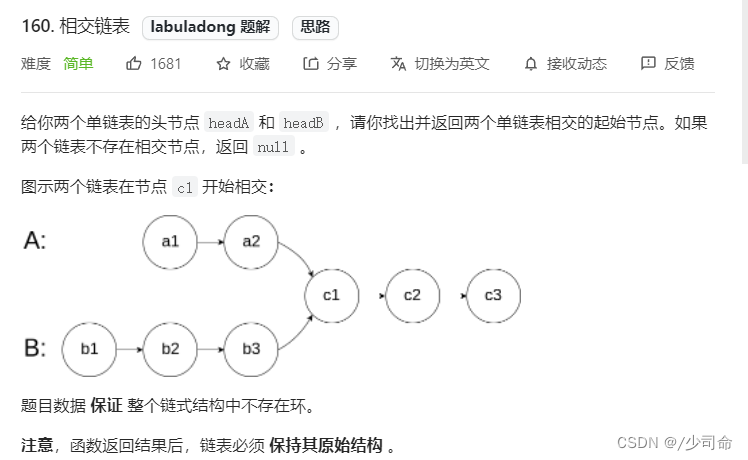
public ListNode getIntersectionNode(ListNode headA, ListNode headB) {
HashSet<ListNode> set = new HashSet<>();
ListNode tmp = headA;
while (tmp != null){
set.add(tmp);
tmp = tmp.next;
}
tmp = headB;
while (tmp != null){
if (set.contains(tmp)){
return tmp;
}
tmp = tmp.next;
}
return null;
}
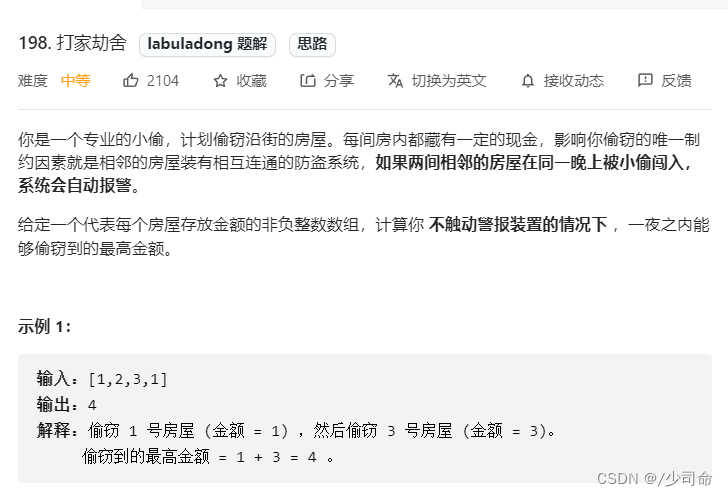
private int[] memo;
public int rob(int[] nums){
memo = new int[nums.length];
Arrays.fill(memo,-1);
return dp(nums,0);
}
private int dp(int[] nums, int i) {
if (i >= nums.length){
return 0;
}
if (memo[i] != -1){
return memo[i];
}
int res = Math.max(dp(nums,i+1),nums[i] + dp(nums,i+2));
memo[i] = res;
return res;
}
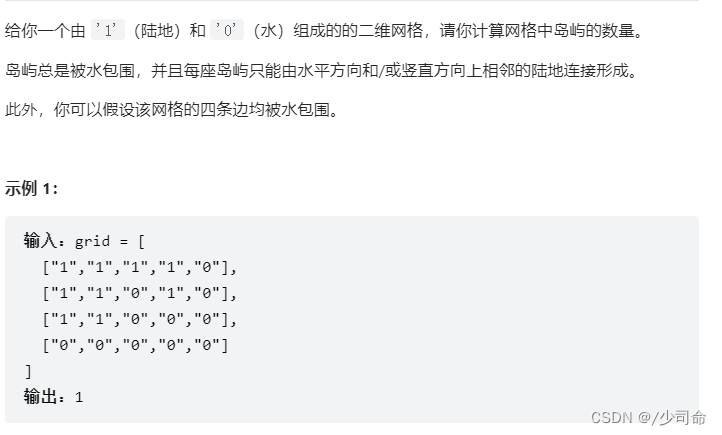
public int numIslands(char[][] grid) {
int res = 0;
int m = grid.length,n = grid[0].length;
for (int i = 0; i < m; i++){
for (int j = 0; j < n; j++){
if (grid[i][j] == '1'){
res++;
dfs(grid,i,j);
}
}
}
return res;
}
private void dfs(char[][] grid, int i, int j) {
int m = grid.length,n = grid[0].length;
if (i < 0 || j < 0 || i >= m || j >= n){
return;
}
if (grid[i][j] == '0'){
return;
}
grid[i][j] = '0';
dfs(grid,i+1,j);
dfs(grid,i,j+1);
dfs(grid,i-1,j);
dfs(grid,i,j-1);
}
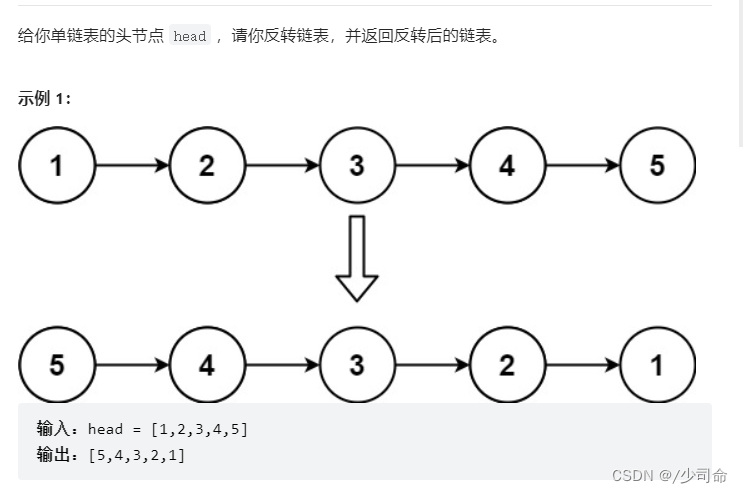
public ListNode reverseList(ListNode head) {
ListNode pre = null;
ListNode cur = head;
while (cur != null){
ListNode curNext = cur.next;
cur.next = pre;
pre = cur;
cur = curNext;
}
return pre;
}
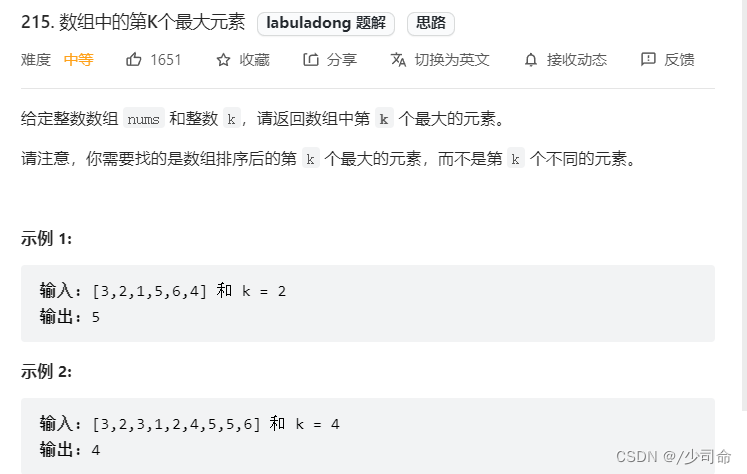
public int findKthLargest(int[] nums, int k) {
PriorityQueue<Integer> pq = new PriorityQueue<>();
for (int e : nums){
pq.offer(e);
if (pq.size() > k){
pq.poll();
}
}
return pq.peek();
}v
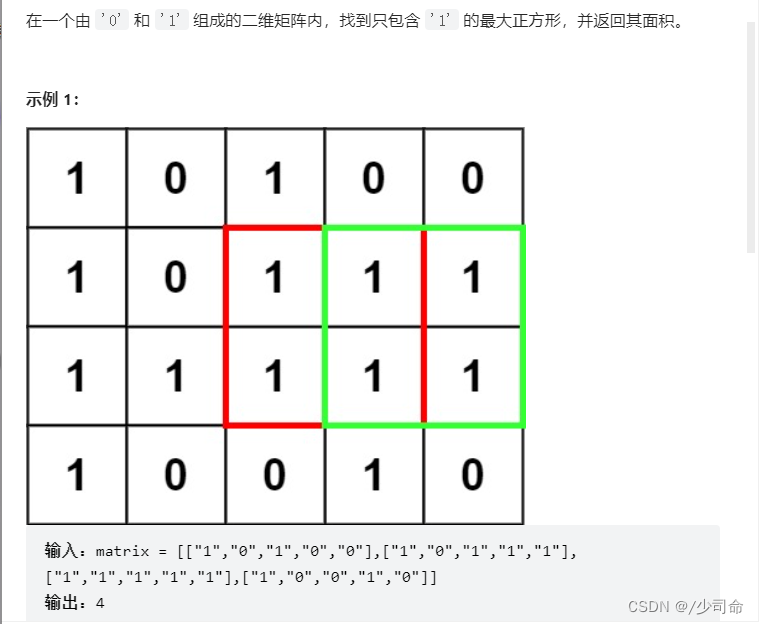
public int maximalSquare(char[][] matrix) {
int m = matrix.length, n = matrix[0].length;
int[][] dp = new int[m][n];
for (int i = 0; i < m; i++){
dp[i][0] = matrix[i][0] - '0';
}
for (int j = 0; j < n; j++){