一、xml文件
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:orientation="vertical">
<EditText
android:id="@+id/et_one"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="5dp"
android:hint="请输入第一个数字"
android:inputType="numberDecimal"/>
<EditText
android:id="@+id/et_two"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="5dp"
android:hint="请输入第二个数字"
android:inputType="numberDecimal"/>
</LinearLayout>
<Button
android:id="@+id/bt_random"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="5dp"
android:text="随机生成" />
<TextView
android:id="@+id/tv_random"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="5dp" />
二、MainActivity.java
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
//输入的数字
private EditText et_one, et_two;
//点击随机生成
private Button bt_random;
//显示随机生成的数字
private TextView tv_random;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initView();
}
private void initView() {
et_one = findViewById(R.id.et_one);
et_two = findViewById(R.id.et_two);
bt_random = findViewById(R.id.bt_random);
tv_random = findViewById(R.id.tv_random);
bt_random.setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.bt_random:
random();
break;
default:
break;
}
}
/**
* DecimalFormat df = new DecimalFormat("0.0");
* 代表保留整数位以及小数一位的数据,小数位会保留0,即1.0格式后结果为1.0
* 若想小数位为0时不保留,为其他数字时保留,则可使用#.#
* 即DecimalFormat df = new DecimalFormat("#.#");
*/
private void random() {
String s1 = et_one.getText().toString();
String s2 = et_two.getText().toString();
if (!StringUtils.equals(s1, "") && !StringUtils.equals(s2, "")) {
try {
double num1 = Double.parseDouble(s1);
double num2 = Double.parseDouble(s2);
Random random = new Random();
double w = 0;
if (num1 >= num2) {
w = random.nextDouble() * (num1 - num2) + num2;
} else if (num2 >= num1) {
w = random.nextDouble() * (num2 - num1) + num1;
}
DecimalFormat df = new DecimalFormat("0.0");//保留1位小数
String str = df.format(w);
tv_random.setText("随机生成的数字:" + str);
} catch (NumberFormatException e) {
e.printStackTrace();
}
} else {
Toast.makeText(this, "请输入数字", Toast.LENGTH_SHORT).show();
}
}
}
三、结果图
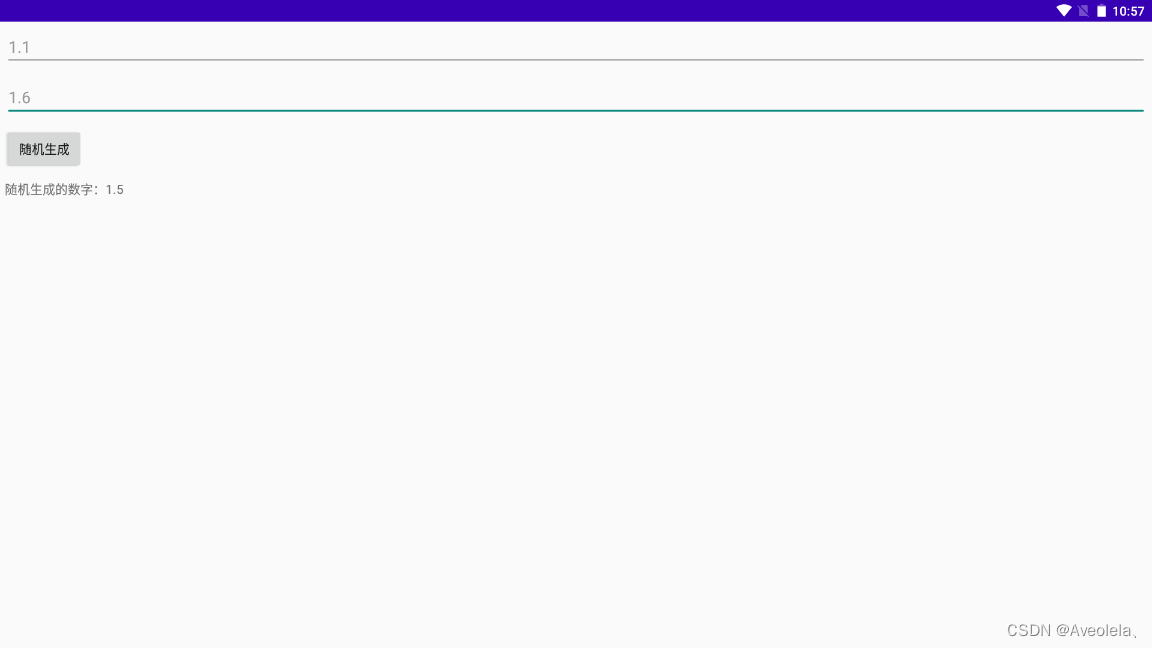