文章目录
1. 事件绑定
React 元素的事件处理和 DOM 元素类似。但是有一点语法上的不同:
- React 事件绑定属性的命名采用驼峰式写法,而不是小写。
- 如果采用 JSX 的语法你需要传入一个函数作为事件处理函数
<button onClick={()=>{this.tick()}}> 增加 </button>
在通过Class创建的组件内,想要使用this调用函数,可以在构造函数内bind this对象为当前this,也可以在绑定处使用this调用
class App extends React.Component{
constructor(props){
super(props)
this.state = {
age:this.props.age
}
this.ageChange = this.ageChange.bind(this);
}
ageChange(){
this.setState({
age:this.state.age+1
})
}
render(){
return <div>
<span>我今年{
this.state.age}岁了</span>
<button onClick={
()=>{
this.ageChange()}}>过年了</button>
</div>
}
}
2. 组件props
组件state 和 props 主要的区别在于 props 是不可变的,而 state 可以根据与用户交互来改变。state是组件内部自己定义的,而props是父组件调用时提供的
function HelloMessage(props) {
return <h1>Hello {
props.name}!</h1>;
}
const element = <HelloMessage name="Runoob"/>;
通过Class创建的组件,使用this调用props,而在使用之前,需要在类构造函数内,使用super(props)
注入
class HelloMessage extends React.Component {
constructor(props){
super(props)
}
render() {
return <h1>Hello {
this.props.name}!</h1>;
}
}
3. 条件渲染
3.1 基本概述
在 React 中,可以创建不同的组件来封装各种你需要的行为。然后还可以根据应用的状态变化只渲染其中的一部分。也可以在React 中的使用 if 或条件运算符来创建表示当前状态的元素,然后让 React 根据它们来更新 UI。
const NavTitle=(props)=>{
if(props.type===1){
return <h1>{
props.title}</h1>
}else if(props.type===2){
return <h2>{
props.title}</h2>
}
}
3.2 三目运算
const NavTitle=(props)=>{
return props.type===1?<h1>{
props.title}</h1>: <h2>{
props.title}</h2>
}
4. 列表
react中,可以通过数组生成一系列标准结构组件,通过map
方法完成列表渲染
const numbers = [1, 2, 3, 4, 5];
const listItems = numbers.map((numbers) =>
<li>{
numbers}</li>
);
生成原理其实是使listItems
成为一个数组容器,容器内部则是<li>{numbers}</li>
组件,最后react内部可直接展开数组渲染
如果直接将numbers
最为组件,则会展开成为内容被渲染
react在列表渲染的时候需要给组件指定一个key
作为组件的唯一标识,该属性不会作为组件的props被接收,仅供react内部计算使用
Key 可以在 DOM 中的某些元素被增加或删除的时候帮助 React 识别哪些元素发生了变化。
5. Refs
5.1 概述
React 支持一种非常特殊的属性 Ref ,你可以用来绑定到render() 输出的标签上。
这个特殊的属性允许你引用相应的实例。
<input ref="myInput" />
var input = this.refs.myInput;
5.2 引用传递 Forwarding Refs
如果需要给组件绑定ref则上面的方式不支持,react提供了forwardRef
方法来支持组件的引用,引用测方式是用过forwardRef
函数传递ref的引用
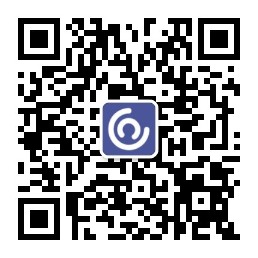
const InpuDom= forwardRef((props, ref) => (
<input type="text" ref={
ref} />
));
class ForwardRef extends Component {
constructor(props) {
super(props);
this.ref = createRef();
}
componentDidMount() {
const {
current } = this.ref;
current.focus();
}
render() {
return (
<div>
<p>forward ref</p>
<InpuDom ref={
this.ref} />
</div>
);
}
}
6. 组件生命周期
组件生命周期的方法有:
- componentWillMount --在渲染前调用
- componentDidMount --在第一次渲染后调用
- componentWillReceiveProps --在组件接收到一个新的 prop (更新后)时被调用。这个方法在初始化render时不会被调用。
- shouldComponentUpdate --返回一个布尔值。在组件接收到新的props或者state时被调用。在初始化时或者使用forceUpdate时不被调用。(可以在你确认不需要更新组件时使用。)
- **componentWillUpdate ** --在组件接收到新的props或者state但还没有render时被调用。在初始化时不会被调用。
- componentDidUpdate --在组件完成更新后立即调用。在初始化时不会被调用。
- **componentWillUnmount ** --在组件从 DOM 中移除之前立刻被调用。