我们先来回顾下shell的典型互动:
[root@localhost epoll]# ls
client.cpp readme.md server.cpp utility.h
[root@localhost epoll]# ps
PID TTY TIME CMD
3451 pts/0 00:00:00 bash
3514 pts/0 00:00:00 ps
用下图的时间轴来表示事件的发生次序。其中时间从左向右。
shell
由标识为
sh
的方块代表,它随着时间的流逝从左向右移动。
shell
从用户读入字符串
"ls"
。
shell
建立一个新的进程,然后在那个进程中运行
ls
程序并等待那个进程结
束。
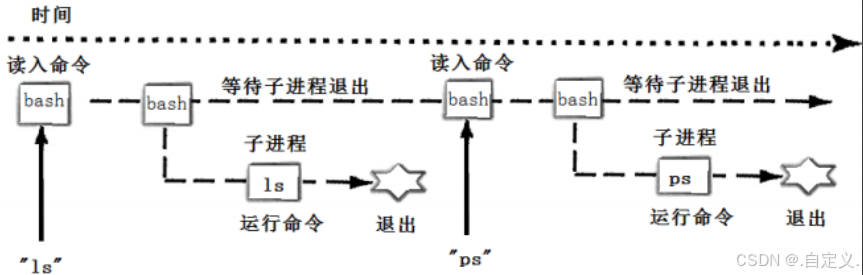
然后
shell
读取新的一行输入,建立一个新的进程,在这个进程中运行程序 并等待这个进程结束。
所以要写一个
shell
,需要循环以下过程
:
1. 获取命令行2. 解析命令行3. 建立一个子进程( fork )4. 替换子进程( execvp )5. 父进程等待子进程退出( wait )
根据这些思路,和我们前面的学的技术,就可以自己来实现一个
shell
了。
完整代码实现:
#define _XOPEN_SOURCE//用于处理putenv函数缺少声明的问题,且必须定义在stdlib.h之前
#include <stdio.h>
#include <unistd.h>
#include <sys/wait.h>
#include <sys/types.h>
#include <string.h>
#include <stdlib.h>
#include <errno.h>
#define SEP " "//标识符,用于截取命令字符串
#define SIZE 512//命令行最多字符数
#define NUM 32//命令行指示数组中最多的指令数
#define ZERO '\0'//处理双回车问题
#define SkipPath(p) do{ p += strlen(p); while(*p != '/') --p; }while(0)//从后往前遍历打印输出工作路径最右值
char* gArgv[NUM];//储存输入截取后的命令行字符串
char cwd[SIZE*2];//用于更新环境变量储存当前工作路径
int lastcode;//错误码
//获取用户名
const char* GetUserName()
{
const char* name = getenv("USER");
if(name == NULL) return "None";
return name;
}
//获取主机名字
const char* GetHomeName()
{
const char* hostname = getenv("HOSTNAME");
if(hostname == NULL) return "None";
return hostname;
}
//获取环境变量PWD打印输出工作路径
const char* GetCwd()
{
const char* cwd = getenv("PWD");
if(cwd == NULL) return "None";
SkipPath(cwd);
return cwd;
}
//获取环变量HOME
const char* GetHOME()
{
const char* home = getenv("HOME");
if(home == NULL) return "/";
return home;
}
void MakeCOmmandLineAndPrint()
{
char commandline[SIZE];
snprintf(commandline,sizeof(commandline),"[%s@%s %s]> ",GetUserName(),GetHomeName(),strlen(GetCwd()) == 1 ? "/" : GetCwd()+1);//这里逗号表达式解决输出工作路径打印根目录的问题
printf("%s",commandline);
fflush(stdout);
}
int GetUserCommand(char line[],size_t n)
{
char* s = fgets(line,n,stdin);
if(s == NULL) return -1;//获取失败
line[strlen(line)-1] = ZERO;//这里处理双回车问题,fgets函数会将输入的回车也写进line中
return strlen(line);
}
void Splitcommand(char line[],size_t n)
{
gArgv[0] = strtok(line,SEP);
size_t i=1;
while((gArgv[i++] = strtok(NULL,SEP)));//循环赋值并判断
}
void ExecuteCommand()
{
pid_t id = fork();
if(id == 0)
{
//child
execvp(gArgv[0],gArgv);//进程替换
exit(errno);
}
else if(id>0)
{
//father
int status = 0;
pid_t rid = waitpid(id,&status,0);
if(rid > 0)
{
//等待成功
lastcode = WEXITSTATUS(status);
if(lastcode != 0)
printf("%s:%s:%d\n",gArgv[0],strerror(lastcode),lastcode);//处理错误码对应错误信息
}
}
else
{
printf("创建子进程失败\n");
}
}
void cd()
{
const char* path = gArgv[1];
if(path == NULL || strcmp(path,"~") == 0)
{
path = GetHOME();//回到家目录
}
//更新路径
chdir(path);
//更新环境变量保证命令行输出工作目录打印正确
char temp[SIZE*2];
getcwd(temp,sizeof(temp));//获取当前工作目录
snprintf(cwd,sizeof(cwd),"PWD=%s",temp);
putenv(cwd);//更新环境变量
}
int CheckBuildin()
{
int yes = 0;//标记是否为内建命令返回
const char* enter_cmd = gArgv[0];
if(strcmp(enter_cmd,"cd") == 0)
{
yes = 1;
cd();
}
else if(strcmp(enter_cmd,"echo")==0 && strcmp(gArgv[1],"$?")==0)
{
yes = 1;
printf("%d\n",lastcode);
lastcode = 0;
}
return yes;
}
int main()
{
int Q=0;
while(!Q)
{
//制作命令行
MakeCOmmandLineAndPrint();
//获取用户命令行字符串
char command[SIZE];
int n = GetUserCommand(command,sizeof(command));
if(n<0) return -1;
//截取命令字符串
Splitcommand(command,sizeof(command));
//判断是否是内建命令
int i = CheckBuildin();
if(i) continue;
//程序替换执行这个命令
ExecuteCommand();
}
return 0;
}