概述
本文通过两种方式播放序列动画,第一种(逐图播放)简单易操作但性能略差,第二种(图集播放)性能相对更好一些但操作相对复杂。不过还是建议使用第二种,因为第二种更适合做合理的架构,不仅仅是序列动画,平时用的 UI 资源也可以一起放到图集资源中,通过不同的名称进行获取。
注意:本文关注的是那种多张图切换的动画,如果需要类似于 Spine 那种形变动画可以看我的另外一篇文章:【Unity】在Unity 3D中使用Spine开发2D动画
播放序列帧动画
准备图片
首先将你所有的图片都导入到项目中,然后在 Project 视窗选中你所有的图片并将其 Texture Type 改为 Sprite(2D and UI),然后确保 Sprite Mode 为 Single 。
如图:
准备脚本代码
using System.Collections;
using UnityEngine;
using UnityEngine.UI;
namespace Core
{
/// <summary>
/// 序列帧动画播放器
/// 将该脚本挂载到拥有Image组件的GameObject下即可
/// framesPerSecond用于调节每一秒播放的帧数
/// sprites用于存放每一帧的图片
/// <remarks>开发者 : Genesis (*╹▽╹*) </remarks>
/// </summary>
public class SequenceFramePlayer : MonoBehaviour
{
/// <summary>
/// 每秒播放的帧数
/// </summary>
public float framesPerSecond = 10.0f;
/// <summary>
/// 存储序列帧动画的所有帧
/// </summary>
public Sprite[] sprites;
private Image _image;
private void Start()
{
_image = GetComponent<Image>();
StartCoroutine(PlayAnimation());
}
private void OnEnable()
{
StartCoroutine(PlayAnimation());
}
private IEnumerator PlayAnimation()
{
while (true)
{
for (var i = 0; i < sprites.Length; i++)
{
if (_image != null)
{
_image.sprite = sprites[i];
}
yield return new WaitForSeconds(1f / framesPerSecond);
}
}
}
}
}
处理场景
在场景中建立一个 Image ,或者给你的UI组件添加一个 Image 组件也行。
使用脚本
将脚本 SequenceFramePlayer 挂载到刚才的 Image 游戏对象上,并将图片拖拽到 Sprites 中,友情提示:Inspector 右上角有个小锁头,我们可以先选中 Image ,然后点击锁定,然后再去使用 Shift 批量选中图片进行拖拽,这样会方便很多。
Frames Per Second 属性是用来调节速度的,也就是每秒钟播放多少张图片。
播放图集动画
修改设置
首先要更改一下设置,操作步骤为 Edit -> Project Settings -> Editor ,将 Sprite Packer -> Mode 设置为 V1 Always 或者 V2。
修改图片资源类型
注意:此处跟前面略有不同,需要将 Mesh Type 设置为 Full Rect 。
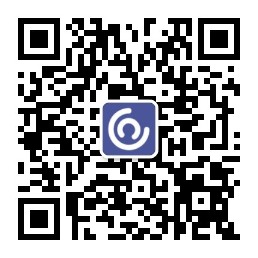
创建 Sprite Atlas
在 Projects 中创建一个 Sprite Atlas ,如下图:
将图集拖放到 Sprite Atlas 的 Objects for Packing 中,然后点击下方的 Pack Preview 按钮进行预览。
准备脚本
using System.Collections;
using UnityEngine;
using UnityEngine.U2D;
using UnityEngine.UI;
namespace Core
{
/// <summary>
/// 图集动画播放器
/// 将该脚本挂载到拥有Image组件的GameObject下即可
/// framesPerSecond用于调节每一秒播放的帧数
/// spriteAtlas需要制定创建好的SpriteAtlas
/// <remarks>开发者 : Genesis (*╹▽╹*) </remarks>
/// </summary>
public class SpriteAtlasPlayer : MonoBehaviour
{
/// <summary>
/// 每秒播放的帧数
/// </summary>
public float framesPerSecond = 10.0f;
public string prefix;
/// <summary>
/// 存储序列帧动画的所有帧
/// </summary>
public SpriteAtlas spriteAtlas;
private Image _image;
private void Start()
{
_image = GetComponent<Image>();
StartCoroutine(PlayAnimation());
}
private void OnEnable()
{
StartCoroutine(PlayAnimation());
}
private IEnumerator PlayAnimation()
{
while (true)
{
for (var i = 0; i < spriteAtlas.spriteCount; i++)
{
if (_image != null)
{
_image.sprite = spriteAtlas.GetSprite($"{
prefix}{
i}");
}
yield return new WaitForSeconds(1f / framesPerSecond);
}
}
}
}
}
使用脚本
与前面的设置基本相同,只不过这次不需要拖动图片,而是直接将刚才创建的 Sprite Atlas 文件放进来即可。
更多内容请查看总目录【Unity】Unity学习笔记目录整理