在Unity3D中,ECS(Entity Component System)架构是一种不同于传统OOP(面向对象编程)的方法,它强调数据驱动的设计,可以显著提升性能和扩展性。在ECS中,主要有三个核心概念:Entity(实体)、Component(组件)和System(系统)。
下面是一个基本的Unity3D ECS例子,用于展示如何创建一个简单的ECS架构来管理实体和组件。
1. 创建Entities
Entities在ECS中是一个唯一的标识符,通常是一个空的容器,仅仅用来持有Components。
2. 创建Components
Components是纯数据结构,没有任何行为。每个Component包含一个实体的特定数据。
3. 创建Systems
Systems包含处理逻辑,操作Entities及其Components。
示例:简单的移动系统
Step 1: 安装必要的包
确保在你的项目中安装了ECS相关的包(如Unity.Entities
,Unity.Transforms
,Unity.Mathematics
等)。
Step 2: 定义Component
创建一个Position和Velocity组件。
using Unity.Entities;
using Unity.Mathematics;
public struct Position : IComponentData
{
public float3 Value;
}
public struct Velocity : IComponentData
{
public float3 Value;
}
Step 3: 创建Entity和添加Component
在一个MonoBehaviour脚本中创建Entity并为其添加Component。
using Unity.Entities;
using Unity.Transforms;
using Unity.Mathematics;
using UnityEngine;
public class EntitySpawner : MonoBehaviour
{
private EntityManager entityManager;
void Start()
{
entityManager = World.DefaultGameObjectInjectionWorld.EntityManager;
// 创建 Archetype
EntityArchetype entityArchetype = entityManager.CreateArchetype(
typeof(Position),
typeof(Velocity)
);
// 创建 Entity
Entity entity = entityManager.CreateEntity(entityArchetype);
// 为 Entity 添加组件数据
entityManager.SetComponentData(entity, new Position {
Value = new float3(0, 0, 0) });
entityManager.SetComponentData(entity, new Velocity {
Value = new float3(1, 0, 0) });
}
}
Step 4: 创建System
创建一个System来更新Position基于Velocity。
using Unity.Entities;
using Unity.Transforms;
using Unity.Mathematics;
public class MovementSystem : SystemBase
{
protected override void OnUpdate()
{
float deltaTime = Time.DeltaTime;
Entities.ForEach((ref Position position, in Velocity velocity) =>
{
position.Value += velocity.Value * deltaTime;
}).Schedule();
}
}
Step 5: 启用System
在Unity中,系统会自动被调度和更新,只要它们存在于当前的世界中。
完整示例
综合以上步骤,完整的代码结构如下:
扫描二维码关注公众号,回复:
17441685 查看本文章
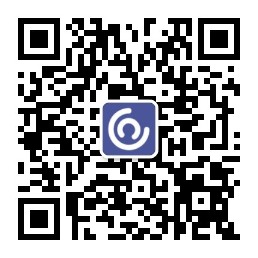
Position.cs
using Unity.Entities;
using Unity.Mathematics;
public struct Position : IComponentData
{
public float3 Value;
}
Velocity.cs
using Unity.Entities;
using Unity.Mathematics;
public struct Velocity : IComponentData
{
public float3 Value;
}
EntitySpawner.cs
using Unity.Entities;
using Unity.Transforms;
using Unity.Mathematics;
using UnityEngine;
public class EntitySpawner : MonoBehaviour
{
private EntityManager entityManager;
void Start()
{
entityManager = World.DefaultGameObjectInjectionWorld.EntityManager;
// 创建 Archetype
EntityArchetype entityArchetype = entityManager.CreateArchetype(
typeof(Position),
typeof(Velocity)
);
// 创建 Entity
Entity entity = entityManager.CreateEntity(entityArchetype);
// 为 Entity 添加组件数据
entityManager.SetComponentData(entity, new Position {
Value = new float3(0, 0, 0) });
entityManager.SetComponentData(entity, new Velocity {
Value = new float3(1, 0, 0) });
}
}
MovementSystem.cs
using Unity.Entities;
using Unity.Transforms;
using Unity.Mathematics;
public class MovementSystem : SystemBase
{
protected override void OnUpdate()
{
float deltaTime = Time.DeltaTime;
Entities.ForEach((ref Position position, in Velocity velocity) =>
{
position.Value += velocity.Value * deltaTime;
}).Schedule();
}
}
这样,一个基本的ECS架构就完成了。这个示例中,我们创建了一个实体,并为它添加了Position和Velocity组件,然后在系统中更新Position以实现基本的移动逻辑。通过这种方式,可以很容易地扩展系统,添加更多的实体和组件,从而实现复杂的游戏逻辑。