目录
2.2 Pattern Matching for instanceof
扫描二维码关注公众号,回复:
17452384 查看本文章
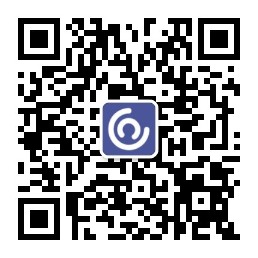
1.简介
Java 17 是一个长期支持(LTS)版本,引入了许多新特性和改进。本文将详细介绍 Java 17 的几个关键特性,并通过示例代码展示它们的实际应用以及这些特性出现之前的使用方法,并分析各自的使用限制。
温馨提示:在使用jdk17新特性的前提是:当前项目环境是jdk17
2.特性介绍
主要新特性进行介绍:
2.1 Sealed Classes
2.1.1 介绍
Sealed Classes 允许开发者限制哪些类可以继承当前类,被限制继承的类用关键字Sealed 修饰,而继承类必须用Sealed 或者non-Sealed或者final关键字修饰。
2.1.2 案例
/**
* 限制类LimitClass只能被LimitClassA和LimitClassB继承,
而继承类LimitClassA和LimitClassB只能被sealed、non-sealed、或final修饰
*/
public abstract sealed class LimitClass permits LimitClassA, LimitClassB{
public abstract void test();
}
/**
* @description: final表示 LimitClassA类不能再被继承
*/
public final class LimitClassA extends LimitClass{
@Override
public void test() {
System.out.println("LimitClassA");
}
}
/**
* @description: non-sealed表示 LimitClassB可以被继承
*/
public non-sealed class LimitClassB extends LimitClass{
@Override
public void test() {
System.out.println("LimitClassB");
}
}
2.1.3 比较
Sealed Classes:
- 优点:确保类层次结构的完整性。
- 缺点:必须显式列出所有允许的子类,增加了代码的复杂度。
传统方式:
- 优点:灵活性高,任何类都可以实现接口。
- 缺点:难以控制类层次结构,可能导致不一致或错误的实现。
2.2 Pattern Matching for instanceof
2.2.1 介绍
Pattern Matching for instanceof 允许在 instanceof 表达式中直接提取字段等信息(
如果变量类型经过instanceof判断能够匹配目标类型,则对应分支中无需再做类型强转。
),简化了类型检查和变量声明,优化之前需要将Object转为指定的对象之后才能提取字段等信息。
2.2.2 案例
新特性之前:
public class InstanceTest {
public static void main(String[] args) {
Object obj = new Point(10, 20);
if (obj instanceof Point) {
//多下面这一步
Point p = (Point) obj;
System.out.println(p.getX() + ", " + p.getY()); // 输出: 10, 20
}
}
public static class Point {
private int x;
private int y;
public Point(int x, int y) {
this.x = x;
this.y = y;
}
public int getX(){
return x;
}
public int getY(){
return y;
}
}
}
新特性:
public class InstanceTest {
public static void main(String[] args) {
Object obj = new Point(10, 20);
if (obj instanceof Point p) {
System.out.println(p.getX() + ", " + p.getY()); // 输出: 10, 20
}
}
public static class Point {
private int x;
private int y;
public Point(int x, int y) {
this.x = x;
this.y = y;
}
public int getX(){
return x;
}
public int getY(){
return y;
}
}
}
2.2.3 比较
Pattern Matching:
- 优点:简化类型检查和变量声明。
- 缺点:需要显式地处理每一种情况,可能增加代码长度。
传统方式:
- 优点:适用于所有类型的对象。
- 缺点:需要额外的类型转换,容易出错。
2.3 Text Blocks
2.3.1 介绍
Text Blocks 允许使用三重引号 (""") 来创建多行字符串,使字符串更易于阅读和编写。
2.3.2 案例
新特性之前:
public class Main {
public static void main(String[] args) {
String html = "<html>\n" +
" <body>\n" +
" <h1>Hello, World!</h1>\n" +
" </body>\n" +
"</html>";
System.out.println(html);
}
}
新特性:
public class Main {
public static void main(String[] args) {
String html = """
<html>
<body>
<h1>Hello, World!</h1>
</body>
</html>
""";
System.out.println(html);
}
}
2.3.3 比较
Text Blocks:
- 优点:提高代码可读性,减少转义字符。
- 缺点:对于简单的字符串,可能会显得冗余。
传统方式:
- 优点:适用于所有字符串。
- 缺点:需要手动添加换行符和缩进,容易出错。
2.4 Vector API (Incubator)
2.4.1 介绍
Vector API 提供了一种高效的方式来进行向量化计算,显著提升了性能
2.4.2 案例
新特性之前:
public class Main {
public static void main(String[] args) {
double[] array = {1.0, 2.0, 3.0, 4.0};
for (int i = 0; i < array.length; i++) {
array[i] += 1.0;
}
for (double d : array) {
System.out.print(d + " ");
}
// 输出: 2.0 3.0 4.0 5.0
}
}
新特性:
import java.lang.foreign.ValueLayout;
import java.lang.invoke.VarHandle;
public class Main {
public static void main(String[] args) {
var vector = VectorSpecies.load(ValueLayout.JAVA_DOUBLE, 1.0, 2.0, 3.0, 4.0);
vector = vector.add(VectorSpecies.load(ValueLayout.JAVA_DOUBLE, 1.0, 2.0, 3.0, 4.0));
System.out.println(vector); // 输出: [2.0, 4.0, 6.0, 8.0]
}
}
2.4.3 比较
Vector API:
- 优点:显著提升性能,适用于大规模数据处理。
- 缺点:API 处于 incubator 阶段,可能不稳定。
传统方式:
- 优点:适用于所有场景。
- 缺点:性能较低,不适合大规模数据处理。
2.5 Records
2.5.1 介绍
Records 是一种特殊的类,主要用于表示不可变的数据容器。它们简化了类的定义,并自动提供了构造器、getter 方法等
2.5.2 案例
新特性之前:
public class Person {
private final String name;
private final int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
public class Main {
public static void main(String[] args) {
Person person = new Person("Alice", 30);
System.out.println(person.getName()); // 输出: Alice
System.out.println(person.getAge()); // 输出: 30
}
}
使用新特性:
record Person(String name, int age) {}
public class Main {
public static void main(String[] args) {
Person person = new Person("Alice", 30);
System.out.println(person.name()); // 输出: Alice
System.out.println(person.age()); // 输出: 30
}
}
2.5.3 比较
Records:
- 优点:简化类定义,自动生成构造器和 getter 方法。
- 缺点:仅适用于简单数据容器,不适用于复杂逻辑。
传统方式:
- 优点:适用于所有
2.6 Switch Expressions
2.6.1 介绍
Switch Expressions 允许将 switch 语句用作表达式,使其更简洁和灵活
2.6.2 案例
新特性之前:
public class Main {
public static void main(String[] args) {
int dayOfWeek = 3;
String dayName;
switch (dayOfWeek) {
case 1:
dayName = "Monday";
break;
case 2:
dayName = "Tuesday";
break;
case 3:
dayName = "Wednesday";
break;
default:
dayName = "Unknown";
}
System.out.println(dayName); // 输出: Wednesday
}
}
新特性:
public class Main {
public static void main(String[] args) {
int dayOfWeek = 3;
String dayName = switch (dayOfWeek) {
case 1 -> "Monday";
case 2 -> "Tuesday";
case 3,4 -> "Wednesday";
default -> "Unknown";
};
System.out.println(dayName); // 输出: Wednesday
}
}
2.6.3 比较
Switch Expressions:
- 优点:简化类型检查,提高代码可读性。
- 缺点:需要显式处理每一种情况,可能增加代码长度。
传统方式:
- 优点:适用于所有场景。
- 缺点:代码冗长,不易维护。