构建Web应用的首选:Flask框架基础与实战
在Python的Web开发生态中,Flask框架以其轻量、灵活和易用的特性成为构建Web应用的首选之一。无论是快速搭建一个小型应用原型,还是构建复杂的后端服务,Flask都提供了便捷的接口和丰富的扩展支持。本博客将介绍Flask的基础知识和核心概念,并通过一个简单的实例展示如何用Flask构建Web应用。
一、Flask框架简介
Flask 是由 Armin Ronacher 开发的一个微框架,其“微”主要体现在它只包含一些Web应用的基本工具,而没有捆绑数据库、表单验证、用户认证等功能。这样做的好处是让开发者拥有更多的选择空间,可以根据需求选择最适合的库和插件。Flask基于Werkzeug工具箱和Jinja2模板引擎,具有以下特点:
- 轻量简洁:只包含最核心的功能,简单易用。
- 灵活性高:自由选择扩展和插件。
- 社区支持广泛:Flask生态活跃,插件丰富。
安装Flask非常简单,可以通过以下命令完成:
pip install flask
二、Flask应用的基本结构
在Flask中,构建一个Web应用主要依赖于一个核心类 Flask
。通常,我们创建Flask应用的基本结构如下:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return "Hello, Flask!"
if __name__ == '__main__':
app.run(debug=True)
代码解析:
- 创建Flask应用实例:
app = Flask(__name__)
将当前模块注册为一个Flask应用。 - 定义路由和视图函数:
@app.route('/')
定义了一个路由,告诉Flask这个视图函数会处理根路径/
的请求。def home()
是视图函数,当用户访问指定路由时会调用该函数返回内容。 - 启动服务器:
app.run(debug=True)
启动Flask的开发服务器,并开启调试模式。
以上代码将启动一个简单的Web服务,用户访问根路径时将收到 Hello, Flask!
的响应。
三、Flask的核心概念
在构建Flask应用时,以下几个核心概念非常重要。
1. 路由(Routing)
路由决定了用户访问URL时会调用哪个视图函数。使用 @app.route()
装饰器定义路由路径和方法:
@app.route('/hello/<name>')
def greet(name):
return f"Hello, {
name}!"
在这里,用户可以通过 /hello/用户名
的路径访问这个视图函数,<name>
是动态变量,将传递给视图函数。
2. 请求对象(Request)
Flask的 request
对象封装了客户端请求的所有信息,例如表单数据、URL参数、Cookies等。可以通过以下方式获取请求中的数据:
from flask import request
@app.route('/submit', methods=['POST'])
def submit():
name = request.form.get('name')
return f"Received data: {
name}"
3. 响应对象(Response)
Flask自动将视图函数的返回值封装为响应对象,但我们也可以手动构建响应对象,以自定义状态码、头部信息等:
from flask import make_response
@app.route('/custom-response')
def custom_response():
response = make_response("Custom response", 202)
response.headers["Content-Type"] = "text/plain"
return response
4. 模板渲染(Templates)
Flask集成了Jinja2模板引擎,使得我们可以将HTML模板和Python代码分离,提升代码复用性和可维护性:
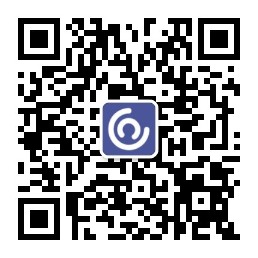
from flask import render_template
@app.route('/welcome/<name>')
def welcome(name):
return render_template('welcome.html', name=name)
在 welcome.html
中,可以通过 {
{ name }}
使用Python变量:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Welcome</title>
</head>
<body>
<h1>Welcome, {
{ name }}!</h1>
</body>
</html>
四、实战:构建一个简单的博客应用
接下来,我们将用Flask构建一个简单的博客应用,包含以下功能:
- 显示所有博客文章
- 查看单篇文章详情
- 新增博客文章
1. 项目结构
我们将创建以下文件结构:
flask_blog/
├── app.py
├── templates/
│ ├── index.html
│ ├── post.html
│ └── new_post.html
└── posts.json
2. 项目代码实现
1)在 app.py
中创建应用:
from flask import Flask, render_template, request, redirect, url_for, jsonify
import json
app = Flask(__name__)
# 加载博客数据
def load_posts():
with open('posts.json') as f:
return json.load(f)
# 保存博客数据
def save_posts(posts):
with open('posts.json', 'w') as f:
json.dump(posts, f)
# 首页,显示所有文章
@app.route('/')
def index():
posts = load_posts()
return render_template('index.html', posts=posts)
# 文章详情页
@app.route('/post/<int:post_id>')
def post(post_id):
posts = load_posts()
post = next((post for post in posts if post['id'] == post_id), None)
return render_template('post.html', post=post)
# 新增文章页面
@app.route('/new', methods=['GET', 'POST'])
def new_post():
if request.method == 'POST':
posts = load_posts()
new_id = max(post['id'] for post in posts) + 1
new_post = {
'id': new_id,
'title': request.form['title'],
'content': request.form['content']
}
posts.append(new_post)
save_posts(posts)
return redirect(url_for('index'))
return render_template('new_post.html')
2)创建模板文件
index.html
:展示所有博客文章列表
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Blog Home</title>
</head>
<body>
<h1>All Blog Posts</h1>
<ul>
{% for post in posts %}
<li><a href="{
{ url_for('post', post_id=post.id) }}">{
{ post.title }}</a></li>
{% endfor %}
</ul>
<a href="{
{ url_for('new_post') }}">Create a new post</a>
</body>
</html>
post.html
:展示单篇博客文章内容
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>{
{ post.title }}</title>
</head>
<body>
<h1>{
{ post.title }}</h1>
<p>{
{ post.content }}</p>
<a href="{
{ url_for('index') }}">Back to all posts</a>
</body>
</html>
new_post.html
:新增文章的表单页面
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>New Post</title>
</head>
<body>
<h1>Create a New Blog Post</h1>
<form method="POST">
<label>Title:</label><br>
<input type="text" name="title"><br><br>
<label>Content:</label><br>
<textarea name="content"></textarea><br><br>
<input type="submit" value="Submit">
</form>
<a href="{
{ url_for('index') }}">Back to all posts</a>
</body>
</html>
3)创建posts.json
文件
[
{
"id": 1, "title": "My First Blog Post", "content": "This is my first post!"},
{
"id": 2, "title": "Another Day", "content": "Here is another blog post."}
]
3. 运行应用
在项目根目录下运行以下命令:
python app.py
然后访问 http://127.0.0.1:5000
,你将看到博客首页,可以查看文章详情并创建新文章。
五、总结
Flask以其简单的API、灵活的配置和丰富的扩展性,成为构建Python Web应用的绝佳选择。在这个简单的博客应用中,我们展示了Flask的路由定义、请求处理、模板渲染和数据交互等基础功能。通过这些基础知识和实例,你可以开始构建更复杂的Web应用,如电商网站、社交网络或数据可视化平台等。
希望本文帮助你快速上手Flask,构建出属于你的Web应用!