文件的上传 和 下载
读取文件
@Test
public void testInputStream(){
InputStream inputStream = null;
try {
// InputStream 读取文件
inputStream = new FileInputStream("test.txt");
// 设置文件读取的缓冲区大小 数组
byte[] bytes = new byte[1024];
// 记录读取的字节数
int len = 0;
// 循环读取文件
while ((len = inputStream.read(bytes))!= -1) {
System.out.println(new String(bytes, 0, len));
}
} catch (IOException e) {
throw new RuntimeException(e);
}finally {
// 关闭流
if (inputStream!= null) {
try {
inputStream.close();
}catch (IOException e){
throw new RuntimeException(e);
}
}
}
}
读取 和 写入 文件
package cn.djx.springio;
import org.junit.jupiter.api.Test;
import org.springframework.boot.test.context.SpringBootTest;
import java.io.*;
@SpringBootTest
class SpringIoApplicationTests {
@Test
public void testInputStream(){
InputStream inputStream = null;
try {
// InputStream 读取文件
inputStream = new FileInputStream("test.txt");
// 设置文件读取的缓冲区大小 数组
byte[] bytes = new byte[1024];
// 记录读取的字节数
int len = 0;
// 循环读取文件
while ((len = inputStream.read(bytes))!= -1) {
System.out.println(new String(bytes, 0, len));
}
} catch (IOException e) {
throw new RuntimeException(e);
}finally {
// 关闭流
if (inputStream!= null) {
try {
inputStream.close();
}catch (IOException e){
throw new RuntimeException(e);
}
}
}
}
@Test
public void testInputStreamAndOutputStream(){
InputStream inputStream = null;
OutputStream outputStream = null;
try {
// InputStream 读取文件
inputStream = new FileInputStream("test.txt");
// OutputStream 写入文件
outputStream = new FileOutputStream("test_copy.txt");
// 设置文件读取的缓冲区大小 数组
byte[] bytes = new byte[1024];
// 记录读取的字节数
int len = 0;
// 循环读取文件
while ((len = inputStream.read(bytes))!= -1) {
// 写入文件
outputStream.write(bytes, 0, len);
// 刷新缓冲区 可与预防数据丢失
outputStream.flush();
}
} catch (IOException e) {
throw new RuntimeException(e);
} if (inputStream!= null) {
// 关闭流
try {
inputStream.close();
outputStream.close();
}catch (IOException e){
throw new RuntimeException(e);
}
}
}
}
AES 加密
引入了依赖
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-crypto</artifactId>
<version>5.8.28</version>
</dependency>
测试代码
package cn.djx.springfile;
import cn.hutool.core.util.CharsetUtil;
import cn.hutool.crypto.SecureUtil;
import cn.hutool.crypto.symmetric.AES;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.boot.test.context.SpringBootTest;
import java.io.*;
@SpringBootTest
class SpringFileApplicationTests {
@Value("${aes.key}")
public String aesKey;
@Test
void testAES() {
String content = "Hello, Spring Boot!";
byte[] key = aesKey.getBytes();
//AES aes = new AES(key);
AES aes = SecureUtil.aes(aesKey.getBytes());
// 加密
byte[] encrypt = aes.encrypt(content);
// 解密
String decrypt = aes.decryptStr(encrypt);
// 加密为16进制表示
String encryptHex = aes.encryptHex(content);
// 解密为字符串
String decryptStr = aes.decryptStr(encryptHex, CharsetUtil.CHARSET_UTF_8);
}
@Test
public void testEncrypt(){
AES aes = SecureUtil.aes(aesKey.getBytes());
// 文件的操作
InputStream inputStream = null;
OutputStream outputStream = null;
try {
// InputStream 读取文件
inputStream = new FileInputStream("test.png");
// OutputStream 写入文件
outputStream = new FileOutputStream("test_encrypt.png");
// 设置文件读取的缓冲区大小 数组
byte[] bytes = inputStream.readAllBytes();
byte[] aesBytes = aes.encrypt(bytes);
// 写入文件
outputStream.write(aesBytes);
// 刷新缓冲区 可与预防数据丢失
outputStream.flush();
} catch (IOException e) {
throw new RuntimeException(e);
} if (inputStream!= null) {
// 关闭流
try {
inputStream.close();
outputStream.close();
}catch (IOException e){
throw new RuntimeException(e);
}
}
}
@Test
public void testDecrypt(){
AES aes = SecureUtil.aes(aesKey.getBytes());
// 文件的操作
InputStream inputStream = null;
OutputStream outputStream = null;
try {
// InputStream 读取文件
inputStream = new FileInputStream("test_encrypt.png");
// OutputStream 写入文件
outputStream = new FileOutputStream("test_decrypt.png");
// 设置文件读取的缓冲区大小 数组
byte[] bytes = inputStream.readAllBytes();
// 写入文件 并解密
outputStream.write(aes.decrypt(bytes));
// 刷新缓冲区 可与预防数据丢失
outputStream.flush();
} catch (IOException e) {
throw new RuntimeException(e);
} if (inputStream!= null) {
// 关闭流
try {
inputStream.close();
outputStream.close();
}catch (IOException e){
throw new RuntimeException(e);
}
}
}
}
源文件
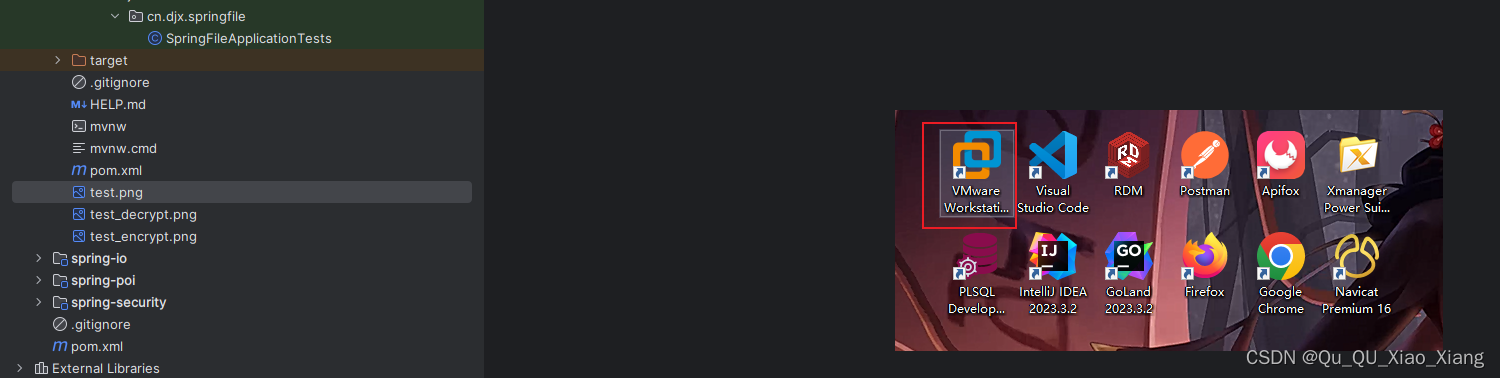
加密文件
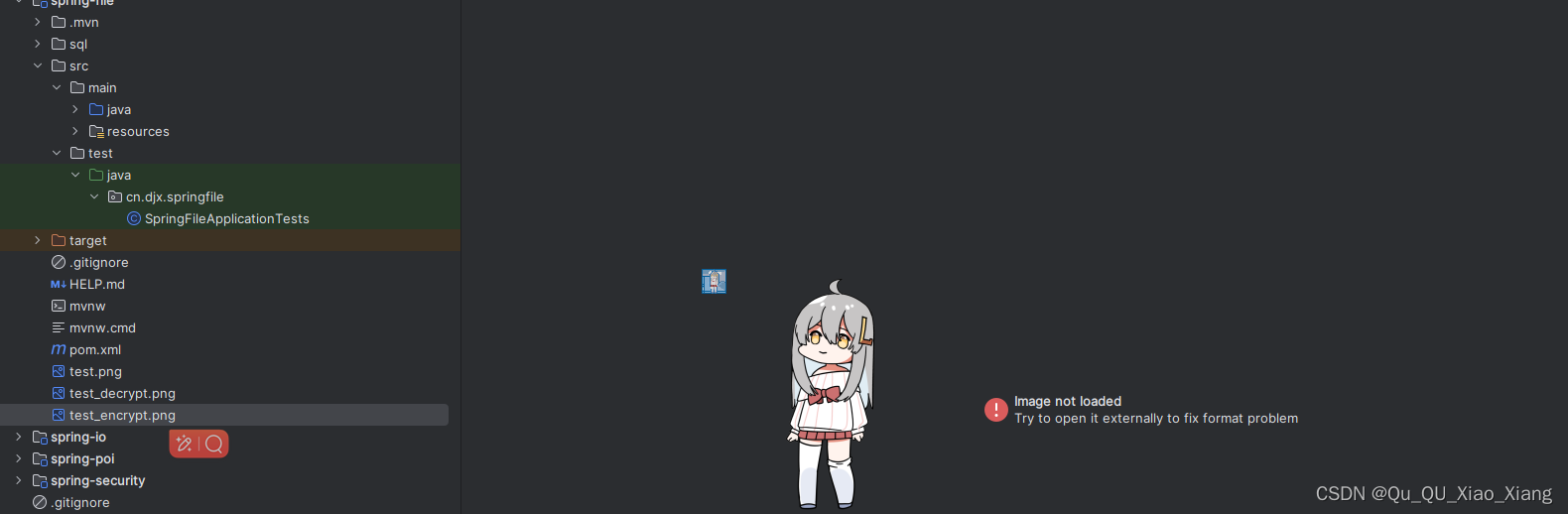
解密文件