There is a brick wall in front of you. The wall is rectangular and has several rows of bricks. The bricks have the same height but different width. You want to draw a vertical line from the top to the bottom and cross the least bricks.
The brick wall is represented by a list of rows. Each row is a list of integers representing the width of each brick in this row from left to right.
If your line go through the edge of a brick, then the brick is not considered as crossed. You need to find out how to draw the line to cross the least bricks and return the number of crossed bricks.
You cannot draw a line just along one of the two vertical edges of the wall, in which case the line will obviously cross no bricks.
Example:
Input: [[1,2,2,1], [3,1,2], [1,3,2], [2,4], [3,1,2], [1,3,1,1]] Output: 2 Explanation:
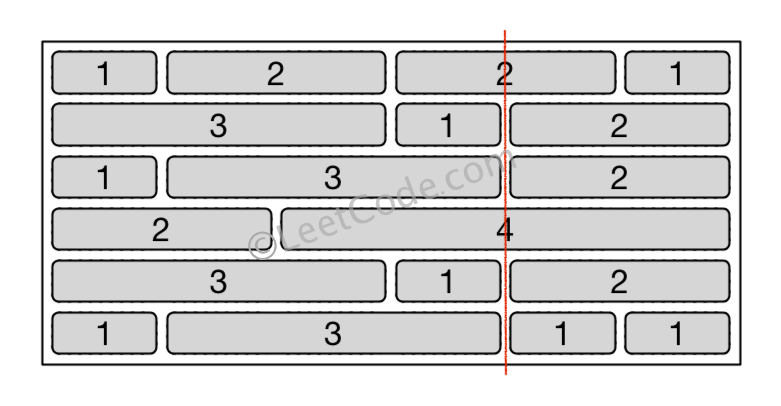
Note:
- The width sum of bricks in different rows are the same and won't exceed INT_MAX.
- The number of bricks in each row is in range [1,10,000]. The height of wall is in range [1,10,000]. Total number of bricks of the wall won't exceed 20,000.
1 class Solution { 2 public int leastBricks(List<List<Integer>> wall) { 3 int res = Integer.MAX_VALUE; 4 int n = wall.size(); 5 for (int times = 0; times < n; times++) { 6 int target = 0; 7 for (int i = 0; i < wall.get(times).size() - 1 ; i++) { 8 int count = 0; 9 target += wall.get(times).get(i); 10 for (int j = 0; j < wall.size(); j++) { 11 List<Integer> cur_wall = wall.get(j); 12 int sum = 0; 13 for (int t : cur_wall) { 14 sum += t; 15 if (sum == target) break; 16 if (sum > target) { 17 count++; 18 break; 19 } 20 } 21 } 22 res = Math.min(res, count); 23 } 24 } 25 return res == Integer.MAX_VALUE?wall.size():res; 26 } 27 }
可以看到是四层循环,第一层循环是从第一堵墙到最后一堵墙,第二层循环是找每堵墙中间的空隙,第三层循环是重新遍历每层墙,第四层循环是计算每个墙到空隙是否单独成立。虽然AC了,但是时间爆炸啊,751ms了解一下。。。肯定不能这么搞,要进行优化。我们发现这个四成循环,其中最后面两层完全是重复前面两层。因此在这里还是有很大的优化空间的。
1 class Solution { 2 public int leastBricks(List<List<Integer>> wall) { 3 int res = wall.size(); 4 Map<Integer,Integer> map = new HashMap<>(); //保存每个空隙长度出现的次数 5 for ( List<Integer> list : wall ){ 6 int sum = 0; 7 for ( int i = 0 ; i < list.size() - 1 ; i ++ ){ 8 sum += list.get(i); 9 map.put(sum,map.getOrDefault(sum,0)+1); 10 } 11 } 12 for ( int length : map.keySet() ){ 13 int temp = map.get(length); 14 res = Math.min(wall.size()-temp,res); 15 } 16 return res; 17 } 18 }
这里还做了个优化,原始的长度设为wall.size()就可以了,发现这个优化竟然提速了14ms,很神奇了。
最后运行时间20ms,击败了97.01%的人。
这个题目收获最大的就是如何进行优化,首先按照最普通的思路做出一个版本,然后看这个版本存在什么问题,然后再针对问题进行优化。