As part of a CS course, Alice just finished programming her robot to explore a graph having nn nodes, labeled 1,2,…,n1,2,…,n, and mm directed edges. Initially the robot starts at node 11.
While nodes may have several outgoing edges, Alice programmed the robot so that any node may have a forced move to a specific one of its neighbors. For example, it may be that node 55 has outgoing edges to neighbors 11, 44, and 66 but that Alice programs the robot so that if it leaves 55 it must go to neighbor 44.
If operating correctly, the robot will always follow forced moves away from a node, and if reaching a node that does not have a forced move, the robot stops. Unfortunately, the robot is a bit buggy, and it might violate those rules and move to a randomly chosen neighbor of a node (whether or not there had been a designated forced move from that node). However, such a bug will occur at most once (and might never happen).
Alice is having trouble debugging the robot, and would like your help to determine what are the possible nodes where the robot could stop and not move again.
We consider two sample graphs, as given in Figures 1 and 2. In these figures, a red arrow indicate an edge corresponding to a forced move, while black arrows indicate edges to other neighbors. The circle around a node is red if it is a possible stopping node.
Figure 1: First sample graph.
Figure 2: Second sample graph.
In the first example, the robot will cycle forever through nodes 11, 55, and 44 if it does not make a buggy move. A bug could cause it to jump from 11 to 22, but that would be the only buggy move, and so it would never move on from there. It might also jump from 55 to 66 and then have a forced move to end at 77.
In the second example, there are no forced moves, so the robot would stay at 11without any buggy moves. It might also make a buggy move from 11 to either 22 or 33, after which it would stop.
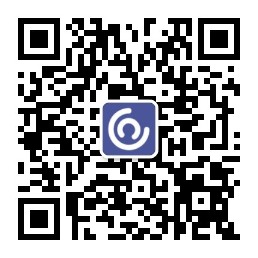
Input
The first line contains two integers nn and mm, designating the number of nodes and number of edges such that 1≤n≤1031≤n≤103, 0≤m≤1040≤m≤104. The next mm lines will each have two integers aa and bb, 1≤|a|,b≤n1≤|a|,b≤n and |a|≠b|a|≠b. If a>0a>0, there is a directed edge between nodes aa and bb that is not forced. If aa < 0, then there is a forced directed edge from −a−a to bb. There will be at most 900900 such forced moves. No two directed edges will be the same. No two starting nodes for forced moves will be the same.
Output
Display the number of nodes at which the robot might come to a rest.
Sample Input 1 | Sample Output 1 |
---|---|
|
|
Sample Input 2 | Sample Output 2 |
---|---|
|
|
题意:给出红黑边,寻找有多少个无法继续下去的点,红边可以走无数次,但是在寻走过程中只可以走一次黑边
思路:(刚开始没有搞清楚题意,后来才明白,一直把红黑边搞反尴尬了)
因为只可以走一次黑边,所以特判黑边是否走过即可,剩下的用DFS搜索,如果无法走下一步即可记录该点。
至于构成点与点之间的关系,采用vector的二维数组,一维是普通的整型,二维是结构体的节点。
(感觉自己还是太菜了,这个题其实不难,思维到不了尴尬)
#include <cstdio>
#include <iostream>
#include <cstring>
#include <algorithm>
#include <map>
#include <set>
#include <queue>
#include <cmath>
using namespace std;
const int MAXN=1e3+5;
typedef long long ll;
struct node
{
int a,b;
int flag;
};
int vis[MAXN];
int ok[MAXN];
int flag=1;
vector<node>s[MAXN];
void DFS(int v)
{
int f=0;
for(int i=0;i<s[v].size();i++){
if(s[v][i].flag){ //红边处理
f=1; //有路可走
if(vis[s[v][i].b])
continue;
vis[s[v][i].b]=1;
DFS(s[v][i].b);
vis[s[v][i].b]=0;
}
else if(flag){
if(vis[s[v][i].b])
continue;
flag=0;
vis[s[v][i].b]=1;
DFS(s[v][i].b);
vis[s[v][i].b]=0;
flag=1;
}
}
if(!f) //无地方可去则终止
ok[v]=1;
}
int main()
{
int n,m;
scanf("%d%d",&n,&m);
memset(vis,0,sizeof(vis));
memset(ok,0,sizeof(ok));
for(int i=1;i<=m;i++) //存贮两点关系
{
int a,b;
node now;
scanf("%d%d",&a,&b);
if(a<0) now.a=-a,now.flag=1; //红边
else now.a=a,now.flag=0; //黑边
now.b=b;
s[now.a].push_back(now);
}
int ans=0;
vis[1]=1;
DFS(1);
for(int i=1;i<=n;i++)
if(ok[i])
ans++;
printf("%d\n",ans);
return 0;
}