在web项目中,定时任务和邮件发送功能是非常常见的,所以这里我将这两个功能添加进去。
内容:每五分钟发送一封邮件给用户(QQ邮箱发送给163邮箱,并且抄送一份给QQ邮箱)。
本次需要导入的jar包为:
mail-1.4.jar
quartz-2.2.3.jar
quartz-jobs-2.2.3.jar
首先,将发送邮件的JOB类完成,同样,注释非常详细,如果有看不太明白的,可以留言咨询。
SendMailJob.java
/**
* 邮件自动发送入口
*/
public void init(){
SendMailJob.send("[email protected]", "标题:newbie邮件测试", "内容:邮件测试", "smtp", "smtp.qq.com", "[email protected]", "端口号", "sendMail", "password");
}
/**
* QQ邮箱发送邮件到163邮箱
*
* @param toMail 收件人
* @param subject 主题
* @param content 内容
* @param smtp 协议
* @param fromHost 发送方的服务器
* @param sendName 邮件发送人
* @param sendPort 邮件发送人端口(可以不指定)
* @param userName 邮件发送人名
* @param userPwd 邮件发送人密码(不是邮箱密码,而是开启SMTP功能时候设置的那个密码)
* @return 成功或失败
*/
public static boolean send(String toMail, String subject, String content, String smtp, String fromHost,
String sendName, String sendPort, String userName, String userPwd) {
// 创建Session
Properties props = new Properties();
// 指定邮件的传输协议,smtp(Simple Mail Transfer Protocol 简单的邮件传输协议)
props.put("mail.transport.protocol", smtp);
// 指定邮件发送服务器服务器 "smtp.qq.com"
props.put("mail.host", fromHost);
// 指定邮件的发送人(QQ邮箱)
props.put("mail.from", sendName);
// 设置安全传输协议为true
props.put("mail.smtp.starttls.enable", "true");
// 设置需要身份验证(不验证会不通过)
props.put("mail.smtp.auth", "true");
Authentication authentication = new Authentication(userName, userPwd);
Session session = Session.getDefaultInstance(props,authentication);
// 开启调试模式(能够在控制台看到日志)
session.setDebug(true);
try {
// 第二步:获取发送方对象
Transport transport = session.getTransport();
// 连接邮件服务器,链接您的QQ邮箱,用户名(可以不用带后缀)、密码
transport.connect(userName, userPwd);
// 接收方地址
Address toAddress = new InternetAddress(toMail);
// 第三步:创建邮件消息体
MimeMessage message = new MimeMessage(session);
// 设置发件人昵称
String nick="";
try {
nick=javax.mail.internet.MimeUtility.encodeText("神级程序员");
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
// 用上面的nick作为发件人的昵称(收到邮件,发件人那一栏的内容)
message.setFrom(new InternetAddress(nick+" <"+sendName+">"));
// 直接用发件人的邮箱作为发件人那一栏的内容
// message.setFrom(new InternetAddress(sendName));
// 邮件的主题
message.setSubject(subject);
// 收件人
message.addRecipient(Message.RecipientType.TO, toAddress);
// 抄送人
Address ccAddress = new InternetAddress("[email protected]");
message.addRecipient(Message.RecipientType.CC, ccAddress);
// 邮件的内容(最简单的方法,纯文本邮件内容)
// message.setContent(content, "text/html;charset=utf-8");
// 向multipart对象中添加邮件的各个部分内容,包括文本内容和附件
Multipart multipart = new MimeMultipart();
// 添加邮件正文 (如果没有附件,可以直接这样设置)
BodyPart contentPart = new MimeBodyPart();
contentPart.setContent(content, "text/html;charset=utf-8");
multipart.addBodyPart(contentPart);
// 添加附件的内容
BodyPart attachmentPart = new MimeBodyPart();
File file = new File("C:\\Users\\Administrator\\Desktop\\新建文本文档.txt");
DataSource source = new FileDataSource(file);
// 将文件资源添加到邮件体中,并设定附件名
attachmentPart.setDataHandler(new DataHandler(source));
attachmentPart.setFileName(MimeUtility.encodeWord(file.getName()));
multipart.addBodyPart(attachmentPart);
message.setContent(multipart);
message.saveChanges();
// 邮件发送时间
message.setSentDate(new Date());
// 第四步:发送邮件
// 第一个参数:邮件的消息体
// 第二个参数:邮件所有的接收人/抄送人
transport.sendMessage(message, message.getAllRecipients());
return true;
} catch (Exception e) {
e.printStackTrace();
}
return false;
}
扫描二维码关注公众号,回复:
2587935 查看本文章
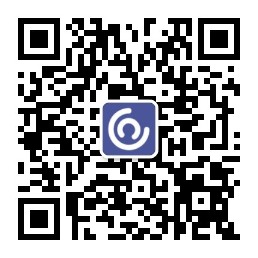
任务类写好后,需要将这个JOB类添加到配置文件中去。
quartz-job.xml
<!-- 邮件自动发送JOB begin -->
<!-- 定义JOB类 -->
<bean id="getStockMoneyDirectionActualJob" class="com.leq.stock.moneydirection.job.GetStockMoneyDirectionActualJob"></bean>
<!-- 指定JOB执行的方法 -->
<bean id="methodGetStockMoneyDirectionActualJob" class="org.springframework.scheduling.quartz.MethodInvokingJobDetailFactoryBean">
<!-- 定时执行的类 -->
<property name="targetObject" ref="getStockMoneyDirectionActualJob"/>
<!-- 具体的方法 -->
<property name="targetMethod" value="init"/>
</bean>
<!-- 调度触发器,设置自己想要的时间规则 -->
<bean id="timeGetStockMoneyDirectionActualTrigger" class="org.springframework.scheduling.quartz.CronTriggerFactoryBean">
<!-- 加入相关的执行类和方法 -->
<property name="jobDetail" ref="methodGetStockMoneyDirectionActualJob"/>
<!-- 设置时间规则 -->
<property name="cronExpression" value="0 0/1 * ? * *"/>
</bean>
<!-- 加入调度工厂 ,设置调度触发器即可-->
<bean class="org.springframework.scheduling.quartz.SchedulerFactoryBean">
<property name="triggers">
<list>
<ref bean="timeGetStockMoneyDirectionActualTrigger"/>
</list>
</property>
</bean>
<!-- 邮件自动发送JOB end -->
配置文件配好后,需要在服务器启动的时候就加载这个配置文件。
上一篇有web.xml的配置详情,这里讲quartz-job.xml添加进去。
web.xml
<!-- 加载Spring容器配置 -->
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<!-- 设置Spring容器加载所有的配置文件的路径 -->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:applicationContext*.xml,classpath:quartz-job.xml</param-value>
</context-param>
好了,大功告成!