1、父组件引入子组件:
父组件引入子组件,三步走:
第一步:引入子组件
import StateWatchListPage from '子组件的路径';
第二步:将子组件名称加入到components对象
components:{
StateWatchListPage
}
第三步:使用子组件
<state-watch-list-page></state-watch-list-page>
2、利用v-for生成的li列表,需要给每个li添加不存在的属性,使用:
<ul>
<li v-for="(item,index) in items" :class="{'active':item.isActive}"
@click="select(item)"><li>
<ul>
Select(item){
this.$nextTick(function(){
this.items.forEach(function(item){ //给每个数组元素添加属性isActive
Vue.set(item,'isActive',false);
});
Vue.set(item,'isActive',true); //给当前选中的元素添加
})
}
其中this.$nextTick(function(){ }); //表示异步更新队列
3、父组件给子组件传值(静态值,即data中的数据):
第一步:父组件的data中定义要传给子组件的值
export default{
data(){
return{
itemLists:[.......],
}
}
}
第二部:父组件与子组件要有契合点
在父组件中调用、注册、引用子组件
调用:
import StateWatchListPage from '子组件的路径';
注册:
components:{
StateWatchListPage
}
引用:
<state-watch-list-page></state-watch-list-page>
第三步:在引用子组件的标签上,将父组件的值绑定给子组件
<state-watch-list-page v-bind:itemLists="itemLists"></state-watch-list-page>
第四步:子组件内部接收父组件传过来的值
props:{
itemLists:{
type:Array,
required:true,
}
}
props验证,定义接收值的类型,并且用对象的形式
传值的类型:变量、方法【不可加括号】、【父组件的this即父组件整个实例】
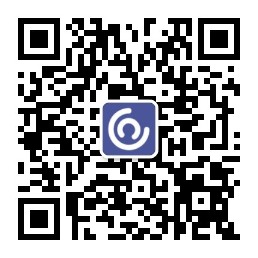
4、如果父组件向子组件中传递的是动态变化的值,那又当如何?
当然是在问题3的基础上了。假设子组件收到的值是字符串tabView:
props:{
tabView:String,
}
那么此时,我们需要在子组件中对接收到的动态变量tabView进行监听,即:
watch:{
tabView(newVal,oldVal){ //注意,函数名是要监听的变量名
this.tabView=newVal;
}
}
5、【获取dom节点的方法】:
给元素添加ref="name",
使用this.$refs.name可以获得dom节点
还可以通过事件对象$event获取当前DOM节点,且可以传递自定义属性
6、使用vue-resource请求数据的步骤:
安装vue-resource:
npm install vue-resource --save
main.js文件中引入:
import VueResource from 'vue-resource';
main.js文件中调用:
Vue.use(VueResource);
在组件中使用:
this.$http.get(url).then((responce)=>{
console.log(responce);
},(error)=>{
console.log(error);
})
7、使用axios请求数据的步骤:
在项目文件中安装vue-resource插件:cd到该项目文件夹,
npm install axios --save
哪里用哪里引入:
import Axios from 'axios';
使用:
Axios.get(url).then((response)=>{
console.log(response);
}).catch((error)=>{
console.log(error);
})
8、父组件主动获取子组件的方法和数据:
调用子组件的时候定义属性ref="name"
在父组件中使用:this.$refs.name.属性/方法
可以调用子组件的方法或数据
反之,子组件主动获取父组件的方法和数据也可以。
9、【非父子组件传值(兄弟组件),即事件广播】:
利用一个空的vue实例作为事件总线:
在vueEvent.js文件中
import Vue from 'vue';
export default new Vue;
组件一广播:
import VueEvent from '../vueEvent.js';
VueEvent.$emit('key',value);
组件二监听(尽量在mounted()周期函数中监听):
import VueEvent from '../vueEvent.js';
VueEvent.$on('key',(message)=>{
console.log(message);
})
【注意】:必须在一个公共的组件中引入这两个传值的组件并调用
10、vue路由配置
第一步:安装
npm install vue-router --save
第二部:main.js文件中引入并use
import VueRouter from 'vue-router';
Vue.use(VueRouter);
第三步:main.js文件中配置路由
引入组件component
import Home from './components/home.component.vue';
import News from './components/news.component.vue';
定义路由:
const routes=[
{path:'/foo',component:Foo},
{path:'/bar',component:Bar},
{path:'*',redirect:'/foo'} //表示默认路由
]
实例化VueRouter
const router=new VueRouter({
routes
})
在main.js文件中挂载:
new Vue({
el:'#app',
router,
render: h => h(App)
})
第四步:在根组件App.vue中放入路由出口
<router-view></router-view>
注意:一定要把routes和router写正确,不然会出问题,而且很难查出来!!!
11、动态路由的配置与获取
在问题10的问题上,
配置:{path:'/car/:aid',component:Car}, //配置动态路由
标签:<router-link to="/car/123"></router-link>
获取:this.$route.params
12、路由的get传值
在问题10的基础上
标签:<router-link to="/car?aid=123"></router-link>
获取:this.$route.query
13、动态路由编程式导航(登录页面点击按钮跳转):
this.$route.push({path:'news'})