题干:启动三个线程打印自增数字,从1一直打印到45,每个线程依次打印5个数字。
首先看两个伪实现:
1.
public class test1 implements Runnable
{
public static int num=1;
@Override
public void run() {
for(int i=0;i<5;i++){
System.out.println(Thread.currentThread().getName()+": "+num++);
}
}
public static void main(String[] args) throws Exception{
for(int i=0;i<3;i++) {
Thread test1 = new Thread(new Main(), "thread1");
test1.start();
test1.join();
Thread test2 = new Thread(new Main(), "thread2");
test2.start();
test2.join();
Thread test3 = new Thread(new Main(), "thread3");
test3.start();
test3.join();
}
}
}
其实,上面启动了3*3个线程;
2
下面方法只有一个主线程,并不是用线程实现,网上有看到有人强写的让这个类继承Thread方法,实现线程类,实际调用的只是该类的run()方法。
public class test2{
String name;
public test3(String name) {
this.name=name;
}
public static int num = 1;
public void run() {
for (int i = 0; i <5; i++) {
System.out.println(name + ": " + num++);
}
}
public static void main(String[] args) {
test3 t1 = new test3("thread1");
test3 t2 = new test3("thread2");
test3 t3 = new test3("thread3");
for(int i=0;i<3;i++){
t1.run();
t2.run();
t3.run();
}
}
}
下面正式进入多线程实现。
3.
扫描二维码关注公众号,回复:
2744726 查看本文章
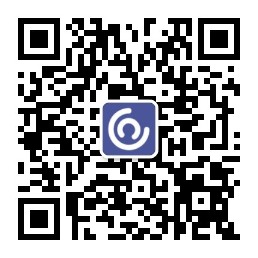
对线程这里初步接触,有的点还是有点不明白,虽然能敲出代码。
先想一个思路:利用线程的synchronized机制,实现线程的等待-唤醒机制,每个线程打印5个数字,则加入等待池,那么问题来了,我该怎样唤醒这些进程呢?有两个方案,利用notify或利用notifyAll:
(1)notify每次随机唤醒,能不能每个进程有一个锁来控制线程顺序——一个线程进行必须等待前一个线程释放锁;这种方法就不上代码了,详细说下思想。
例如,例如,大的while控制打印的数字在45范围,线程1每打印5个数字,释放自身的锁,等待前一个锁,线程2可以动了,动完记得释放自身锁,等待1的锁,……这个有点麻烦,代码参考https://blog.csdn.net/c______________/article/details/78655374,
感觉这个实现起来逻辑不好控制,于是想了想其他方案。
(2)notifyAll唤醒所有线程,那么不该唤醒的也唤醒了咋整,所以注意下面代码标红部分,引入标记位state,每次进来判断下,没轮到他,什么也没做,立即加入等待池。
class run implements Runnable {
private int count=0;
@Override
public void run() {
while(count<3){//这个线程进行三次打印操作,每次打印5个数字
count++;
new Main().printNum(Thread.currentThread().getName());
}
}
}
public class Main {
public static int num=1;
public static int state=1;
public void printNum(String tag) {
synchronized (Main.class) {
int intTag = Integer.parseInt(tag);
while (intTag != state) {
try {
Main.class.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
for (int i = 0; i < 5; i++) {
System.out.println(Thread.currentThread().getName() + ": " + num++);
}
state = state % 3+1; //state=(state+1)%3不一样的效果
Main.class.notifyAll();
}
}
public static void main(String[] args){
new Thread(new run(),1+"").start();
new Thread(new run(),2+"").start();
new Thread(new run(),3+"").start();
}
}