批注:由于python GIL破坏了python使用cpu执行并发的可能性,
多线程不纯粹,但至少保证能够跑多个任务,共享全局变量不出错
1、最简单的多线程

import threading import time #简单的并发 def run(n): print("task ",n) time.sleep(2) start_time = time.time() t_thread = [] for i in range(50): t = threading.Thread(target=run,args=("task-%s" %i,)) t.start() t_thread.append(t) for t in t_thread: t.join() print("main thread .....",threading.current_thread()) print("cost time:",time.time()-start_time)
2、守护线程
import threading import time #简单的并发 def run(n): print("task ",n) time.sleep(1) print("task-%s...done",threading.current_thread()) start_time = time.time() t_thread = [] for i in range(50): t = threading.Thread(target=run,args=("task-%s" %i,)) t.setDaemon(True) #把当前线程设置为守护线程 t.start() t_thread.append(t) # # for t in t_thread: # t.join() time.sleep(1) print("main thread .....",threading.current_thread()) print("cost time:",time.time()-start_time)
注意这里和上面的差别,运行时间能看出来,因为主线程已经不需要等待子线程运行完成
不知道用的多不多,以后遇上了再更新使用
3、线程上锁(互斥)

import threading import time #简单的并发 def run(n): print("task ",n) lock.acquire() time.sleep(2) global num num += 1 lock.release() print("task-%s...done",threading.current_thread()) num = 0 # lock = threading.Lock() lock = threading.RLock()#在多重锁的情况使用,递归锁,否则死锁 start_time = time.time() t_thread = [] for i in range(10): t = threading.Thread(target=run,args=("task-%s" %i,)) t.start() t_thread.append(t) for t in t_thread: t.join() while threading.active_count() !=1: print(threading.active_count()) else: print("main thread .....",threading.current_thread()) print("num ",num) print("cost time:",time.time()-start_time)
多个线程同时访问全局变量需要锁住临界区
4、简单的信号量使用

import threading,os import time def run(n): semaphore.acquire() print("run ",n) time.sleep(1) semaphore.release() semaphore = threading.BoundedSemaphore(5) for i in range(10): t = threading.Thread(target=run,args=("task-%s" %i,)) t.start() while threading.active_count() != 1: pass else: print("main thread....",threading.current_thread())
5、Event的使用(同步)

import threading import time def light(): count = 0 while True: if count >5 and count <10: #红灯 event.clear() print("\033[41;1mred light is on...\033[0m") elif count >=10: #标志位 event.set() count = 0 else: print("\033[42;1mgreen light is on...\033[0m") time.sleep(1) count += 1 def car(name): while True: if event.is_set(): print("%s running..." %name) time.sleep(1) else: print("red light on....") event.wait() event = threading.Event() event.set() lighter = threading.Thread(target=light) lighter.start() car = threading.Thread(target=car,args=("aotu",)) car.start()
6、生产者、消费者模型
扫描二维码关注公众号,回复:
2762762 查看本文章
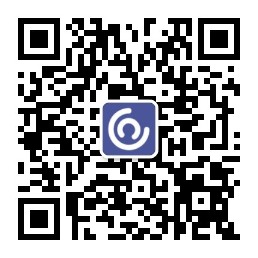

import queue,time import threading q = queue.Queue(maxsize=10) def producer(name): count = 1 while True: print("%s produce %d" %(name,count)) q.put("product %s" %count) time.sleep(0.5) count += 1 def consumer(name): while True: if q.qsize() > 0: print("%s is eating %s" %(name,q.get())) else: continue p = threading.Thread(target=producer,args=("qu",)) c = threading.Thread(target=consumer,args=("Li",)) c1 = threading.Thread(target=consumer,args=("wang",)) p.start() c.start() c1.start()
线程中可以串接各种操作,使用面也很广,因此很重要!